Modularisation in Android: Engineering Scalable, Maintainable Projects
Modularisation is not just a buzzword — it’s a necessity in today’s world of ever-growing codebases. I have witnessed firsthand the pitfalls of monolithic projects and the transformative benefits of a well‐modularised architecture. In this article, we’ll cover not only the “what” and “why” of modularisation but also how to architect your project into clean, maintainable, and scalable modules. We will discuss concepts, common patterns, and essential best practices along the way. The Growing Codebase Problem As Android projects expand, complexity increases rapidly. What starts as a neat project may soon morph into an unmanageable spaghetti codebase. When your app comprises thousands of classes and interwoven dependencies, building, testing, and scaling become significant challenges. Over time, new features introduce more moving parts, and even minor changes can trigger unforeseen side effects in unrelated areas. A monolithic approach often leads to long build times, low testability, and difficulty in onboarding new team members. In contrast, modularisation breaks down the monolith into discrete units with clear responsibilities, allowing teams to work more autonomously and confidently. As described in the Android Developers’ guide, a modularised codebase improves maintainability, scalability, and overall code quality by isolating concerns and enforcing separation between components. What Is Modularisation? At its core, modularisation is the practice of organising your application into self-contained, loosely coupled modules. Each module encapsulates a specific functionality or domain, has a well-defined public API, and hides its internal implementation details. This separation means that each module can be developed, tested, and maintained independently. The benefits of modularisation manifest themselves in several ways: Reusability: Modules can be shared across multiple apps. For instance, a feature module — say, a news feed — can be enabled or disabled depending on the app flavour, making your project more versatile. Strict Visibility Control: With modularisation, you enforce clear boundaries using visibility modifiers like private or internal. This prevents module internals from leaking out into the rest of the codebase. Customisable Delivery: Modular architectures work well with modern packaging systems like Play Feature Delivery, allowing features to be delivered conditionally or on-demand. Simply put, modularisation is a way to decompose a large problem into smaller, manageable problems — a practice that has proven indispensable throughout my career. Benefits of a Modularised Codebase Let’s delve deeper into the tangible benefits that a modularised project offers: Scalability When modules are loosely coupled, a change in one module rarely forces widespread modifications across your codebase. This isolation is crucial as your project grows, ensuring that different teams can work on separate modules concurrently without stepping on each other’s toes. In a modular architecture, the separation of concerns limits how changes in one module affect others, resulting in shorter build times and easier debugging. Ownership and Accountability Modularisation naturally lends itself to dedicated module ownership. Each module can have a responsible team or developer who takes charge of its upkeep, bug fixes, and refactoring efforts. This focused accountability encourages better testing and quality assurance practices, since the module’s maintainers truly “own” its code quality. Encapsulation Encapsulation ensures that modules expose only what is necessary. Internal workings, helper functions, and specific implementations remain hidden, reducing the risk of inadvertent changes from external dependencies. This means that if you need to refactor internals, you can do so without impacting the entire project. Testability Isolated modules are far easier to test. When your modules interact via clearly defined interfaces, you can write unit tests for each module without having to bootstrap the entire app. This significantly improves the reliability of tests and speeds up the development cycle. Improved Build Times Gradle offers incremental builds, parallel processing, and caching features that work best with a modularised project. By splitting the project into multiple modules, only the modules that have changed get recompiled, thus minimising build time overhead. Common Pitfalls in Modularisation While modularisation offers tremendous benefits, it is not without its challenges. Here are some pitfalls that you should avoid based on lessons learned over the years: Over-Modularisation (Too Fine-Grained) It might seem that “more is better,” but over-segmentation can introduce needless complexity. Each module comes with its own overhead: build configurations, boilerplate code, and potential for misconfiguration. I
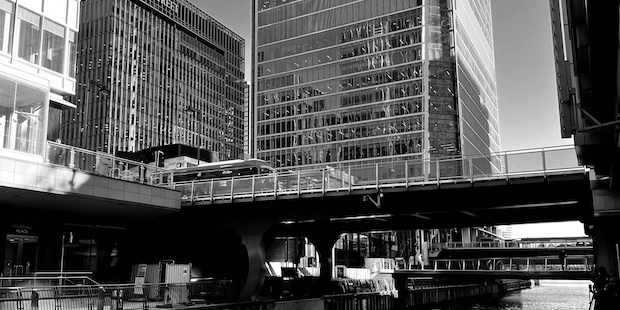
Modularisation is not just a buzzword — it’s a necessity in today’s world of ever-growing codebases. I have witnessed firsthand the pitfalls of monolithic projects and the transformative benefits of a well‐modularised architecture. In this article, we’ll cover not only the “what” and “why” of modularisation but also how to architect your project into clean, maintainable, and scalable modules. We will discuss concepts, common patterns, and essential best practices along the way.
The Growing Codebase Problem
As Android projects expand, complexity increases rapidly. What starts as a neat project may soon morph into an unmanageable spaghetti codebase. When your app comprises thousands of classes and interwoven dependencies, building, testing, and scaling become significant challenges. Over time, new features introduce more moving parts, and even minor changes can trigger unforeseen side effects in unrelated areas.
A monolithic approach often leads to long build times, low testability, and difficulty in onboarding new team members. In contrast, modularisation breaks down the monolith into discrete units with clear responsibilities, allowing teams to work more autonomously and confidently. As described in the Android Developers’ guide, a modularised codebase improves maintainability, scalability, and overall code quality by isolating concerns and enforcing separation between components.
What Is Modularisation?
At its core, modularisation is the practice of organising your application into self-contained, loosely coupled modules. Each module encapsulates a specific functionality or domain, has a well-defined public API, and hides its internal implementation details. This separation means that each module can be developed, tested, and maintained independently.
The benefits of modularisation manifest themselves in several ways:
- Reusability: Modules can be shared across multiple apps. For instance, a feature module — say, a news feed — can be enabled or disabled depending on the app flavour, making your project more versatile.
- Strict Visibility Control: With modularisation, you enforce clear boundaries using visibility modifiers like private or internal. This prevents module internals from leaking out into the rest of the codebase.
- Customisable Delivery: Modular architectures work well with modern packaging systems like Play Feature Delivery, allowing features to be delivered conditionally or on-demand.
Simply put, modularisation is a way to decompose a large problem into smaller, manageable problems — a practice that has proven indispensable throughout my career.
Benefits of a Modularised Codebase
Let’s delve deeper into the tangible benefits that a modularised project offers:
Scalability
When modules are loosely coupled, a change in one module rarely forces widespread modifications across your codebase. This isolation is crucial as your project grows, ensuring that different teams can work on separate modules concurrently without stepping on each other’s toes.
In a modular architecture, the separation of concerns limits how changes in one module affect others, resulting in shorter build times and easier debugging.
Ownership and Accountability
Modularisation naturally lends itself to dedicated module ownership. Each module can have a responsible team or developer who takes charge of its upkeep, bug fixes, and refactoring efforts. This focused accountability encourages better testing and quality assurance practices, since the module’s maintainers truly “own” its code quality.
Encapsulation
Encapsulation ensures that modules expose only what is necessary. Internal workings, helper functions, and specific implementations remain hidden, reducing the risk of inadvertent changes from external dependencies. This means that if you need to refactor internals, you can do so without impacting the entire project.
Testability
Isolated modules are far easier to test. When your modules interact via clearly defined interfaces, you can write unit tests for each module without having to bootstrap the entire app. This significantly improves the reliability of tests and speeds up the development cycle.
Improved Build Times
Gradle offers incremental builds, parallel processing, and caching features that work best with a modularised project. By splitting the project into multiple modules, only the modules that have changed get recompiled, thus minimising build time overhead.
Common Pitfalls in Modularisation
While modularisation offers tremendous benefits, it is not without its challenges. Here are some pitfalls that you should avoid based on lessons learned over the years:
Over-Modularisation (Too Fine-Grained)
It might seem that “more is better,” but over-segmentation can introduce needless complexity. Each module comes with its own overhead: build configurations, boilerplate code, and potential for misconfiguration. If your modules are too fine-grained, you risk ending up with a system that is as hard to manage as a monolith.
Under-Modularisation (Too Coarse-Grained)
On the other hand, if your modules are too coarse, you might inadvertently recreate a monolithic structure that doesn’t truly offer the benefits of separation. For instance, keeping your entire data layer within one module might be acceptable for a small project, but as your project scales, separating repositories and data sources into distinct modules becomes necessary for maintaining clarity.
Excessive Coupling
Even with modularisation, it is possible to introduce tight coupling between modules. A common mistake is having modules depend on one another’s inner implementation. The high cohesion and low coupling principle emphasises that modules should interact only through well-defined public interfaces. Overly interconnected modules lead to a scenario where a change in one module causes cascading modifications throughout the system.
Patterns for Android Modularisation
There isn’t a one-size-fits-all approach to modularisation, rather, you need to select a pattern that aligns with your project’s requirements. The Android Developers documentation outlines several common patterns, which I have found immensely useful in various projects.
Data Modules
Data modules encapsulate the logic that deals with data retrieval, storage, and transformation. Here are a few key aspects:
- Encapsulation of Business Logic: A data module focuses on a specific domain, containing repositories, data sources, and model classes.
- Separation of Concerns: It hides the implementation details of the data sources behind a repository interface, exposing only a clean API to consumers.
- Visibility Control: Use Kotlin’s internal or private modifiers to ensure that the specifics of data handling remain confined within the module.
In practice, this means that if you have a module managing user data, only the repository’s public methods are exposed to the rest of the app, safeguarding against unintended alterations from other modules.
Feature Modules
Feature modules represent isolated aspects of your app’s functionality — each one usually corresponds to a specific user-facing feature (like a checkout flow, profile screen, or a news feed).
- Encapsulation of UI & Logic: A feature module typically includes screen layouts, ViewModels, and associated business logic.
- Independent Deployment: With tools like Play Feature Delivery, feature modules can be delivered conditionally or on-demand, reducing the initial download size and providing a flexible user experience.
- Inter-module Dependencies: While feature modules must rely on shared data modules for business logic, they should not depend on each other directly to avoid tight coupling. These modules allow teams to iterate on features independently and even swap out entire parts of your app without affecting the core functionality.
App Modules
The app module serves as the entry point of the application. It ties together feature modules and provides the navigation and dependency injection setup required to assemble the application at runtime.
- Multiple App Modules: If your application targets various platforms like wearables, TVs, or cars, it might be beneficial to have distinct app modules for each platform. This separation minimises the platform-specific dependencies and adaptations.
- Root Navigation: The app module typically owns the navigation graph and coordinates transitions between feature modules, making it essential to maintain low coupling here.
Common or Core Modules
Common modules contain utility code and shared resources that cut across multiple features. Typical examples include:
- UI Modules: For consistent theming and shared UI components.
- Analytics Modules: To track user interactions and app events, ensuring that the analytics logic is centralised.
- Network Modules: Providing a common HTTP client and network configuration that all feature modules can use.
- Utility Modules: Containing helper functions, string resources, and other generic code that would otherwise be duplicated. By pulling these common dependencies into separate modules, you minimise redundancy and ensure that any changes ripple through your entire codebase systematically.
Test Modules
Test modules are an essential part of any modularised architecture. They isolate test code and resources from production code, making them easier to manage and scale.
- Shared Test Code: When multiple modules share test utilities (such as custom assertions, test data, or mock objects), a dedicated test module reduces duplication and simplifies maintenance.
- Cleaner Build Configurations: Isolating test dependencies in their own modules means your main build files remain free of clutter, leading to simpler and more maintainable configurations.
- Integration Tests: These modules also play a role in isolating integration tests that span multiple modules, ensuring that cross-module interactions are verified systematically.
Module-to-Module Communication
Even in a decoupled environment, modules rarely operate in complete isolation. You must manage inter-module communication while preserving the low coupling and high cohesion objectives. A common scenario involves one module triggering an action in another — say, a user selects an item in a product listing (feature module A), and the checkout feature (feature module B) must receive that data.
A practical solution is to use a mediating module, often owned by the app module that manages navigation. Instead of passing complex objects directly, use primitive identifiers (such as an ID) that the receiving module can use to fetch the full object from a shared data repository. This pattern minimises dependencies by not exposing the internal structure of your data .
For example, when navigating from a home screen to a checkout screen, pass a simple book ID through the navigation component:
navController.navigate("checkout/$bookId")
Inside the checkout module’s ViewModel, you would then use a saved state handle to retrieve and process the ID, ensuring that each module remains responsible for managing its own data retrieval. This approach adheres to the single source of truth principle and minimises tight coupling between modules.
Embracing Dependency Inversion
As your modules start to interact, the principle of dependency inversion becomes critical. In a well-modularised architecture, higher-level modules should not depend on the details of lower-level modules. Instead, both should depend on abstractions.
Abstract Contracts
Define clear contracts — often via interfaces — that outline the interactions between modules. For instance, instead of having a feature module directly instantiate a repository from a data module, define an interface that both the data module and the consumer of the data adhere to. This approach lets you swap out the concrete implementation without affecting downstream code.
Using Dependency Injection Frameworks
Frameworks such as Hilt and Dagger simplify the management of dependencies across modules. They automatically resolve module dependencies at compile-time or runtime, ensuring that each module only depends on the abstractions it needs. This practice not only improves testability but also helps in enforcing the desired boundaries between modules.
Real-World Lessons Learned
In my journey from smaller apps to multi-featured platforms, several crucial lessons have emerged:
- Start Early: Ideally, modularisation should be part of your project’s architecture from day one. Retrofitting a modular structure into an existing monolith is possible but requires significant effort and disciplined refactoring.
- Adopt Incrementally: You don’t have to modularise everything at once. Start by isolating the most volatile or complex parts of your app and gradually carve out additional modules as the codebase grows.
- Balance is Key: Strive for the sweet spot between too fine-grained and too coarse modules. This balance will ensure that the benefits of modularisation (improved build time, testability, and scalability) are realised without introducing unnecessary overhead.
- Document Your Architecture: As your application evolves, clear documentation of module boundaries and interdependencies becomes a living reference for your team. This is particularly important when onboarding new developers or scaling up teams.
- Automate Integration Testing: With independent modules, integration tests become critical in ensuring that changes in one module do not inadvertently break another. Investing in automated integration testing ensures that your loosely coupled modules communicate effectively without regression.
Final Thoughts
Modularisation in Android development is more than an architectural decision — it’s a practice that influences every aspect of your development lifecycle. From reducing build times to fostering a culture of ownership and accountability, modularisation empowers teams to build robust, scalable, and maintainable applications.
By decomposing your application into data, feature, app, common, and test modules, you create a codebase that not only scales but also adapts to evolving requirements with minimal friction. Embrace dependency inversion, enforce strict visibility boundaries, and use mediating modules for communication to preserve the low-coupling/high-cohesion balance.
With the Android Developers’ guidelines and patterns as your blueprint , you can set up an architecture that not only supports current needs but also paves the way for future innovations. As with any architectural decision, there are trade-offs and challenges. However, with a clear strategy in place — and lessons learned from years of hands-on development — the rewards of modularisation far outweigh its initial costs.
In our fast-paced development environment, where rapid iteration is as essential as code quality, modularisation stands out as a key enabler for sustainable growth. By thinking about your app as a composition of interacting modules, you’re building not just an app, but an ecosystem that is prepared for the future.
In conclusion, modularisation is both a strategy and an art. It requires a keen understanding of your app’s domain, a disciplined approach to code separation, and a commitment to continuous improvement. As Android developers, our goal is to write code that is as clean and robust as it is innovative. A modular architecture is a powerful tool in achieving that vision.