Mastering Python: A Comprehensive Guide
Mastering Python: A Comprehensive Guide Python is one of the most versatile and widely used programming languages today. Whether you're a beginner or an experienced developer, mastering Python can open doors to numerous opportunities in web development, data science, automation, and more. In this guide, we'll explore Python's core concepts, best practices, and advanced techniques to help you become proficient. And if you're looking to monetize your web programming skills, check out MillionFormula—a great platform to turn your expertise into income. Why Learn Python? Python's popularity stems from its simplicity, readability, and vast ecosystem. Here’s why it’s a must-learn language: Easy to Learn: Python’s syntax is intuitive, making it beginner-friendly. Versatile: Used in web development (Django, Flask), data science (Pandas, NumPy), AI (TensorFlow, PyTorch), and automation. Strong Community Support: Extensive documentation and active communities like Stack Overflow and Python’s Official Docs. Setting Up Python Before diving in, ensure you have Python installed: Download Python from the official website. Verify Installation: bashCopyDownload python --version Use a Virtual Environment (Best Practice): bashCopyDownload python -m venv myenv source myenv/bin/activate # Linux/Mac .myenvScriptsactivate # Windows Python Basics You Must Know 1. Variables and Data Types Python is dynamically typed, meaning you don’t need to declare variable types explicitly. pythonCopyDownloadname = "Alice" # String age = 25 # Integer height = 5.9 # Float is_programmer = True # Boolean 2. Control Structures Python uses indentation (no braces!) for blocks. pythonCopyDownload# If-Else if age >= 18: print("Adult") else: print("Minor") # Loops for i in range(5): # 0 to 4 print(i) while age
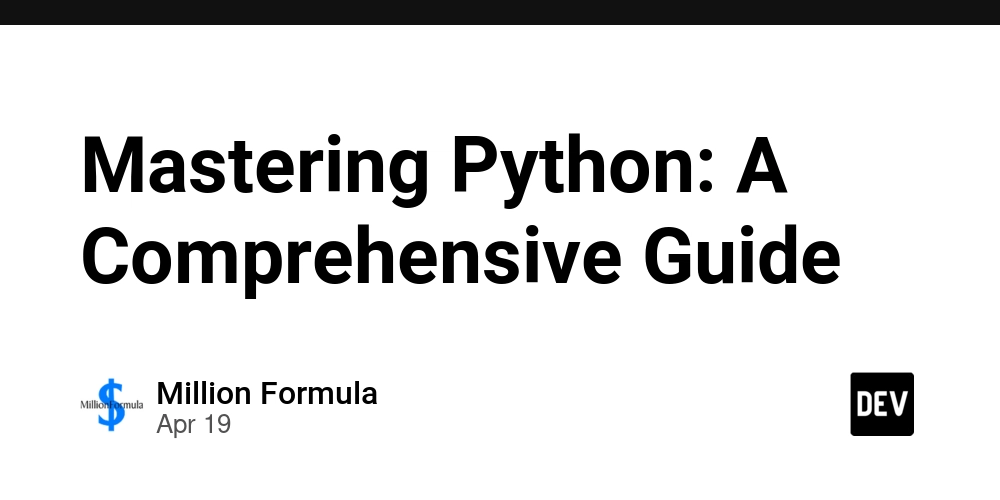
Mastering Python: A Comprehensive Guide
Python is one of the most versatile and widely used programming languages today. Whether you're a beginner or an experienced developer, mastering Python can open doors to numerous opportunities in web development, data science, automation, and more. In this guide, we'll explore Python's core concepts, best practices, and advanced techniques to help you become proficient.
And if you're looking to monetize your web programming skills, check out MillionFormula—a great platform to turn your expertise into income.
Why Learn Python?
Python's popularity stems from its simplicity, readability, and vast ecosystem. Here’s why it’s a must-learn language:
Easy to Learn: Python’s syntax is intuitive, making it beginner-friendly.
Versatile: Used in web development (Django, Flask), data science (Pandas, NumPy), AI (TensorFlow, PyTorch), and automation.
Strong Community Support: Extensive documentation and active communities like Stack Overflow and Python’s Official Docs.
Setting Up Python
Before diving in, ensure you have Python installed:
Download Python from the official website.
-
Verify Installation:
bashCopyDownload
python --version
-
Use a Virtual Environment (Best Practice):
bashCopyDownload
python -m venv myenv
source myenv/bin/activate # Linux/Mac
.myenvScriptsactivate # Windows
Python Basics You Must Know
1. Variables and Data Types
Python is dynamically typed, meaning you don’t need to declare variable types explicitly. pythonCopyDownload
name = "Alice" # String
age = 25 # Integer
height = 5.9 # Float
is_programmer = True # Boolean
2. Control Structures
Python uses indentation (no braces!) for blocks. pythonCopyDownload
# If-Else
if age >= 18:
print("Adult")
else:
print("Minor")# Loops
for i in range(5): # 0 to 4
print(i)while age < 30:
age += 1
3. Functions
Functions are defined using def
.
pythonCopyDownload
def greet(name):
return f"Hello, {name}!"print(greet("Bob")) # Output: Hello, Bob!
4. Lists, Tuples, and Dictionaries
Lists are mutable.
Tuples are immutable.
Dictionaries store key-value pairs.
fruits = ["apple", "banana", "cherry"] # List
coordinates = (10.0, 20.0) # Tuple
person = {"name": "Alice", "age": 25} # Dictionary
Intermediate Python Concepts
1. List Comprehensions
A concise way to create lists. pythonCopyDownload
squares = [x**2 for x in range(10)]
2. Error Handling with Try-Except
Prevent crashes by handling exceptions. pythonCopyDownload
try:
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero!")
3. Working with Files
Read and write files easily. pythonCopyDownload
with open("file.txt", "r") as file:
content = file.read()
4. Object-Oriented Programming (OOP)
Python supports classes and inheritance. pythonCopyDownload
class Dog:
def init(self, name):
self.name = namedef bark(self): return "Woof!"
dog = Dog("Buddy")
print(dog.bark()) # Output: Woof!
Advanced Python Techniques
1. Decorators
Modify functions dynamically. pythonCopyDownload
def logger(func):
def wrapper(args, kwargs):
print(f"Calling {func.name}")
return func(args, **kwargs)
return wrapper@logger
def say_hello():
print("Hello!")say_hello() # Logs before execution
2. Generators
Efficiently handle large datasets. pythonCopyDownload
def count_up_to(n):
i = 1
while i <= n:
yield i
i += 1for num in count_up_to(5):
print(num) # Output: 1, 2, 3, 4, 5
3. Multithreading
Run tasks concurrently. pythonCopyDownload
import threadingdef print_numbers():
for i in range(5):
print(i)thread = threading.Thread(target=print_numbers)
thread.start()
Python for Web Development
Python powers major web frameworks:
Here’s a simple Flask app: pythonCopyDownload
from flask import Flask
app = Flask(name)@app.route("/")
def home():
return "Hello, Flask!"if name == "main":
app.run()
Run it with: bashCopyDownload
flask run
Python for Data Science
Libraries like Pandas and NumPy make Python a data powerhouse. pythonCopyDownload
import pandas as pddata = {"Name": ["Alice", "Bob"], "Age": [25, 30]}
df = pd.DataFrame(data)
print(df)
Where to Go Next?
Contribute to open-source projects on GitHub.
Solve coding challenges on LeetCode.
Build real-world projects to solidify skills.
And if you're eager to monetize your web development expertise, MillionFormula offers fantastic opportunities.
Final Thoughts
Mastering Python takes practice, but its rewards are immense. Whether you're automating tasks, building web apps, or analyzing data, Python’s flexibility makes it indispensable.
Start coding today, and unlock endless possibilities!