Learn AbortController with Examples - Never to look back again
In modern JavaScript development, managing asynchronous tasks such as data fetching, animations, timeouts, or user interactions can be challenging. One elegant solution introduced for managing these tasks, particularly for graceful cancellation, is the AbortController API. Although introduced in 2017, many developers are still unfamiliar with its full capabilities. This article provides a thorough understanding of AbortController—what it is, how it functions, and appropriate use cases. What is AbortController? AbortController is a built-in JavaScript API that lets you cancel asynchronous operations like fetch() before they complete. It works in tandem with an AbortSignal, which is passed to async functions like fetch(). When AbortController.abort() is called, the linked signal is marked as aborted, triggering any listeners or checks on that signal. Core concept You create an AbortController instance, pass its signal to the task, and invoke .abort() whenever cancellation is required. Basic Usage with fetch() const controller = new AbortController(); const signal = controller.signal; fetch('https://api.example.com/data', { signal }) .then(response => response.json()) .then(data => console.log(data)) .catch(err => { if (err.name === 'AbortError') { console.log('Fetch aborted'); } else { console.error('Fetch error:', err); } }); // Abort the fetch after 2 seconds setTimeout(() => controller.abort(), 2000); Understanding the Components 1. AbortController: An object created to control cancellation. Provides the method abort(). 2. AbortSignal: Accessible as controller.signal. Passed to asynchronous operations. Emits an abort event when abort() is called. Real-World Use Cases 1. 1. Search Suggestions (Live Typing) let controller; function search(query) { if (controller) controller.abort(); controller = new AbortController(); fetch(`/api/search?q=${query}`, { signal: controller.signal }) .then(res => res.json()) .then(showResults) .catch(err => { if (err.name !== 'AbortError') console.error(err); }); } 2. React Cleanup in useEffect() useEffect(() => { const controller = new AbortController(); fetch('/api/user', { signal: controller.signal }) .then(res => res.json()) .then(setUserData); return () => controller.abort(); // Clean up }, []); 3. Cancelable Timeout function delay(ms, signal) { return new Promise((resolve, reject) => { const timer = setTimeout(resolve, ms); signal.addEventListener('abort', () => { clearTimeout(timer); reject(new Error('Aborted')); }); }); } const controller = new AbortController(); delay(5000, controller.signal) .then(() => console.log('Done')) .catch(console.error); controller.abort(); // Cancel the delay Browser & Library Support All modern browsers (Chrome, Firefox, Safari, Edge). Node.js (version 15 and above or through polyfills). Libraries such as Axios (version 1.1 and above) support AbortController via the signal property. Pro Tips Always verify signal.aborted before initiating long-running tasks. Use signal.addEventListener('abort', ...) for customized asynchronous logic. Aborting a signal only impacts operations that actively listen for it. Summary The AbortController API provides an effective method for cancelling asynchronous tasks in JavaScript. Whether managing fetch() requests, timeouts, or complex application logic, incorporating AbortController results in cleaner, more efficient, and responsive code. If you have not yet adopted this API, consider integrating it into your development practices today. Thank you very for reading this article through the end. Please leave a like, if it helped you and do checkout my other articles in Never to look back series.
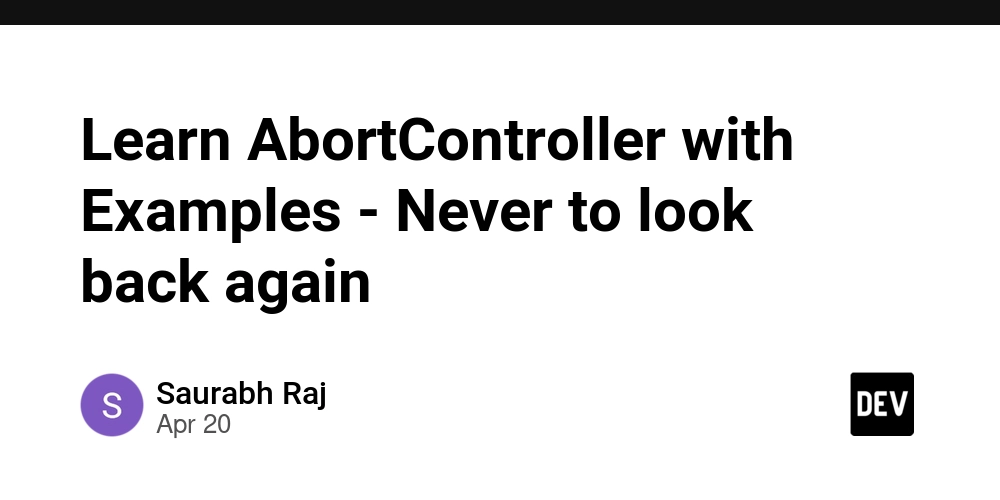
In modern JavaScript development, managing asynchronous tasks such as data fetching, animations, timeouts, or user interactions can be challenging. One elegant solution introduced for managing these tasks, particularly for graceful cancellation, is the AbortController API. Although introduced in 2017, many developers are still unfamiliar with its full capabilities. This article provides a thorough understanding of AbortController—what it is, how it functions, and appropriate use cases.
What is AbortController?
AbortController is a built-in JavaScript API that lets you cancel asynchronous operations like fetch() before they complete.
It works in tandem with an AbortSignal
, which is passed to async functions like fetch(). When AbortController.abort()
is called, the linked signal is marked as aborted, triggering any listeners or checks on that signal.
Core concept
You create an AbortController instance, pass its signal to the task, and invoke .abort()
whenever cancellation is required.
Basic Usage with fetch()
const controller = new AbortController();
const signal = controller.signal;
fetch('https://api.example.com/data', { signal })
.then(response => response.json())
.then(data => console.log(data))
.catch(err => {
if (err.name === 'AbortError') {
console.log('Fetch aborted');
} else {
console.error('Fetch error:', err);
}
});
// Abort the fetch after 2 seconds
setTimeout(() => controller.abort(), 2000);
Understanding the Components
1. AbortController:
- An object created to control cancellation.
- Provides the method
abort()
.
2. AbortSignal:
- Accessible as
controller.signal
. - Passed to
asynchronous operations
. - Emits an
abort event
whenabort()
is called.
Real-World Use Cases
1. 1. Search Suggestions (Live Typing)
let controller;
function search(query) {
if (controller) controller.abort();
controller = new AbortController();
fetch(`/api/search?q=${query}`, { signal: controller.signal })
.then(res => res.json())
.then(showResults)
.catch(err => {
if (err.name !== 'AbortError') console.error(err);
});
}
2. React Cleanup in useEffect()
useEffect(() => {
const controller = new AbortController();
fetch('/api/user', { signal: controller.signal })
.then(res => res.json())
.then(setUserData);
return () => controller.abort(); // Clean up
}, []);
3. Cancelable Timeout
function delay(ms, signal) {
return new Promise((resolve, reject) => {
const timer = setTimeout(resolve, ms);
signal.addEventListener('abort', () => {
clearTimeout(timer);
reject(new Error('Aborted'));
});
});
}
const controller = new AbortController();
delay(5000, controller.signal)
.then(() => console.log('Done'))
.catch(console.error);
controller.abort(); // Cancel the delay
Browser & Library Support
- All modern browsers (Chrome, Firefox, Safari, Edge).
- Node.js (version 15 and above or through polyfills).
- Libraries such as Axios (version 1.1 and above) support AbortController via the signal property.
Pro Tips
- Always verify
signal.aborted
before initiating long-running tasks. - Use
signal.addEventListener('abort', ...)
for customized asynchronous logic. - Aborting a signal only impacts operations that actively listen for it.
Summary
The AbortController API provides an effective method for cancelling asynchronous tasks in JavaScript. Whether managing fetch() requests, timeouts, or complex application logic, incorporating AbortController results in cleaner, more efficient, and responsive code. If you have not yet adopted this API, consider integrating it into your development practices today.
Thank you very for reading this article through the end. Please leave a like, if it helped you and do checkout my other articles in Never to look back series.