Javascript Strings
JavaScript String: A string in JavaScript is a sequence of characters used to represent text. Strings are immutable, meaning once created, they cannot be changed. You can create strings using single quotes ('), double quotes ("), or backticks (`) for template literals. JS String Storage(): JavaScript strings are stored as sequences of UTF-16 code units. Each character is represented by one or two 16-bit code units. This allows JavaScript to handle a wide range of characters, including those from various languages and emoji. String() Constructor: The String constructor creates a new String object. It's generally better to use string literals for simplicity. Example: let str = new String("Hello"); console.log(str); // [String: 'Hello'] Builtin Strings in JS: JavaScript provides built-in string objects and methods for string manipulation. These methods include charAt(), concat(), includes(), indexOf(), slice(), split(), toLowerCase(), toUpperCase(), and many more. Convert String to Camel Case: To convert a string to camel case, you can use a combination of replace() and regular expressions. Example: function toCamelCase(str) { return str.replace(/(?:^\w|[A-Z]|\b\w|\s+)/g, function(match, index) { return index === 0 ? match.toLowerCase() : match.toUpperCase(); }).replace(/\s+/g, ''); } console.log(toCamelCase("hello world")); // "helloWorld" Count String Occurrences: To count the occurrences of a substring within a string, you can use the split() method and check the length of the resulting array. Example: function countOccurrences(str, subStr) { return str.split(subStr).length - 1; "hello")); // 2 String Methods: JavaScript provides various methods to manipulate strings: Sure! Here are the detailed explanations of the string methods in JavaScript, combined with their definitions and examples: charAt(index): Definition: Returns the character at the specified index in a string. Parameter: index: The position of the character you want to retrieve. It is zero-based, meaning the first character is at index 0. Example: let str = "Hello"; console.log(str.charAt(1)); // "e" concat(str1, str2, ...): Definition: Concatenates the string arguments to the calling string and returns a new string. Parameters: str1, str2, ...: One or more strings to concatenate to the calling string. Example: let str1 = "Hello"; let str2 = "World"; console.log(str1.concat(" ", str2)); // "Hello World" includes(subStr, start): Definition: Determines whether the string contains the specified substring, returning true or false. Parameters: subStr: The substring to search for within the string. start (optional): The position in the string at which to begin searching. Default is 0. Example: let str = "Hello World"; console.log(str.includes("World")); // true indexOf(subStr, start): Definition: Returns the index of the first occurrence of the specified substring, or -1 if not found. Parameters: subStr: The substring to search for within the string. start (optional): The position in the string at which to begin searching. Default is 0. Example: let str = "Hello World"; console.log(str.indexOf("World")); // 6 lastIndexOf(subStr, start): Definition: Returns the index of the last occurrence of the specified substring, or -1 if not found. Parameters: subStr: The substring to search for within the string. start (optional): The position in the string at which to begin searching backwards. Default is the length of the string. Example: let str = "Hello World World"; console.log(str.lastIndexOf("World")); // 12 slice(start, end): Definition: Extracts a section of the string and returns it as a new string, without modifying the original string. Parameters: start: The zero-based index at which to begin extraction. end (optional): The zero-based index before which to end extraction. The character at this index will not be included. If omitted, extracts to the end of the string. Example: let str = "Hello World"; console.log(str.slice(0, 5)); // "Hello" split(separator, limit): Definition: Splits the string into an array of substrings, using the specified separator. Parameters: separator: Specifies the character, or regular expression, to use for splitting the string. If omitted, the entire string is returned as a single element array. limit (optional): An integer that limits the number of splits. Example: let str = "Hello World"; let arr = str.split(" "); console.log(arr); // ["Hello", "World"] toLowerCase(): Definition: Converts the entire string to lowercase. Parameters: None. Example: let str = "Hello World"; console.log(str.toLowerCase()); // "hello world" toUpperCase(): Definition: Converts the entire string to uppercase. Parameters: None. Ex
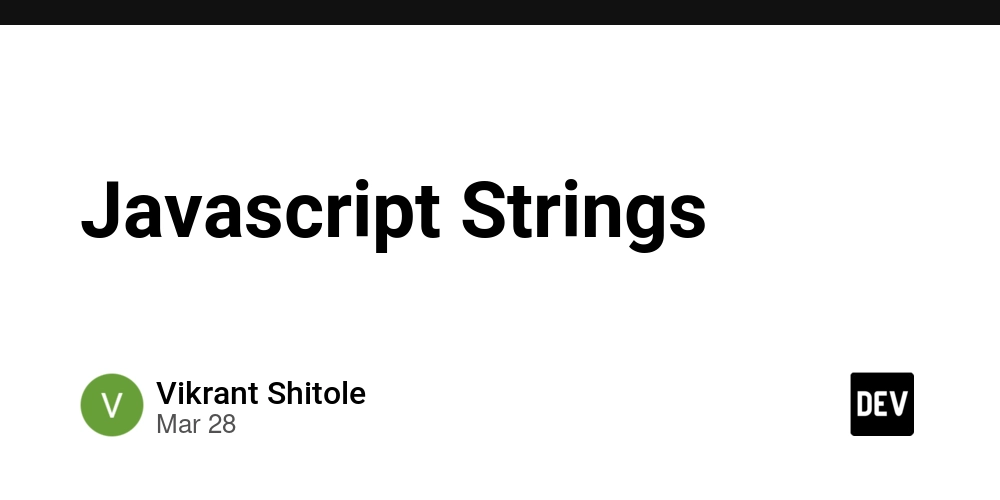
-
JavaScript String:
- A string in JavaScript is a sequence of characters used to represent text. Strings are immutable, meaning once created, they cannot be changed. You can create strings using single quotes (
'
), double quotes ("
), or backticks (`
) for template literals.
- A string in JavaScript is a sequence of characters used to represent text. Strings are immutable, meaning once created, they cannot be changed. You can create strings using single quotes (
-
JS String Storage():
- JavaScript strings are stored as sequences of UTF-16 code units. Each character is represented by one or two 16-bit code units. This allows JavaScript to handle a wide range of characters, including those from various languages and emoji.
-
String() Constructor:
- The
String
constructor creates a new String object. It's generally better to use string literals for simplicity. Example:
let str = new String("Hello"); console.log(str); // [String: 'Hello']
- The
-
Builtin Strings in JS:
- JavaScript provides built-in string objects and methods for string manipulation. These methods include
charAt()
,concat()
,includes()
,indexOf()
,slice()
,split()
,toLowerCase()
,toUpperCase()
, and many more.
- JavaScript provides built-in string objects and methods for string manipulation. These methods include
-
Convert String to Camel Case:
- To convert a string to camel case, you can use a combination of
replace()
and regular expressions. Example:
function toCamelCase(str) { return str.replace(/(?:^\w|[A-Z]|\b\w|\s+)/g, function(match, index) { return index === 0 ? match.toLowerCase() : match.toUpperCase(); }).replace(/\s+/g, ''); } console.log(toCamelCase("hello world")); // "helloWorld"
- To convert a string to camel case, you can use a combination of
-
Count String Occurrences:
- To count the occurrences of a substring within a string, you can use the
split()
method and check the length of the resulting array. Example:
function countOccurrences(str, subStr) { return str.split(subStr).length - 1; "hello")); // 2
- To count the occurrences of a substring within a string, you can use the
-
String Methods:
- JavaScript provides various methods to manipulate strings: Sure! Here are the detailed explanations of the string methods in JavaScript, combined with their definitions and examples:
-
charAt(index)
:- Definition: Returns the character at the specified index in a string.
-
Parameter:
-
index
: The position of the character you want to retrieve. It is zero-based, meaning the first character is at index 0.
-
- Example:
let str = "Hello"; console.log(str.charAt(1)); // "e"
-
concat(str1, str2, ...)
:- Definition: Concatenates the string arguments to the calling string and returns a new string.
-
Parameters:
-
str1, str2, ...
: One or more strings to concatenate to the calling string.
-
- Example:
let str1 = "Hello"; let str2 = "World"; console.log(str1.concat(" ", str2)); // "Hello World"
-
includes(subStr, start)
:-
Definition: Determines whether the string contains the specified substring, returning
true
orfalse
. -
Parameters:
-
subStr
: The substring to search for within the string. -
start
(optional): The position in the string at which to begin searching. Default is 0.
-
- Example:
let str = "Hello World"; console.log(str.includes("World")); // true
-
Definition: Determines whether the string contains the specified substring, returning
-
indexOf(subStr, start)
:-
Definition: Returns the index of the first occurrence of the specified substring, or
-1
if not found. -
Parameters:
-
subStr
: The substring to search for within the string. -
start
(optional): The position in the string at which to begin searching. Default is 0.
-
- Example:
let str = "Hello World"; console.log(str.indexOf("World")); // 6
-
Definition: Returns the index of the first occurrence of the specified substring, or
-
lastIndexOf(subStr, start)
:-
Definition: Returns the index of the last occurrence of the specified substring, or
-1
if not found. -
Parameters:
-
subStr
: The substring to search for within the string. -
start
(optional): The position in the string at which to begin searching backwards. Default is the length of the string.
-
- Example:
let str = "Hello World World"; console.log(str.lastIndexOf("World")); // 12
-
Definition: Returns the index of the last occurrence of the specified substring, or
-
slice(start, end)
:- Definition: Extracts a section of the string and returns it as a new string, without modifying the original string.
-
Parameters:
-
start
: The zero-based index at which to begin extraction. -
end
(optional): The zero-based index before which to end extraction. The character at this index will not be included. If omitted, extracts to the end of the string.
-
- Example:
let str = "Hello World"; console.log(str.slice(0, 5)); // "Hello"
-
split(separator, limit)
:- Definition: Splits the string into an array of substrings, using the specified separator.
-
Parameters:
-
separator
: Specifies the character, or regular expression, to use for splitting the string. If omitted, the entire string is returned as a single element array. -
limit
(optional): An integer that limits the number of splits.
-
- Example:
let str = "Hello World"; let arr = str.split(" "); console.log(arr); // ["Hello", "World"]
-
toLowerCase()
:- Definition: Converts the entire string to lowercase.
- Parameters: None.
- Example:
let str = "Hello World"; console.log(str.toLowerCase()); // "hello world"
-
toUpperCase()
:- Definition: Converts the entire string to uppercase.
- Parameters: None.
- Example:
let str = "Hello World"; console.log(str.toUpperCase()); // "HELLO WORLD"
-
trim()
:- Definition: Removes whitespace from both ends of the string.
- Parameters: None.
- Example:
let str = " Hello World "; console.log(str.trim()); // "Hello World"
-
replace(searchValue, newValue)
:-
Definition: Replaces occurrences of
searchValue
withnewValue
in the string. -
Parameters:
-
searchValue
: The substring or regular expression to search for. -
newValue
: The string to replace thesearchValue
with.
-
- Example:
let str = "Hello World"; let newStr = str.replace("World", "JavaScript"); console.log(newStr); // "Hello JavaScript"
-
Definition: Replaces occurrences of
-
substring(start, end)
:-
Definition: Returns a subset of the string between the
start
andend
indices. -
Parameters:
-
start
: The index at which to begin extraction. -
end
(optional): The index before which to end extraction. If omitted, extracts to the end of the string.
-
- Example:
let str = "Hello World"; console.log(str.substring(0, 5)); // "Hello"
-
Definition: Returns a subset of the string between the
-
substr(start, length)
:-
Definition: Returns a subset of the string starting at the
start
index and extending for a given number of characters (length
). -
Parameters:
-
start
: The index at which to begin extraction. -
length
(optional): The number of characters to extract. If omitted, extracts to the end of the string.
-
- Example:
let str = "Hello World"; console.log(str.substr(0, 5)); // "Hello"
-
Definition: Returns a subset of the string starting at the
-
charCodeAt(index)
:- Definition: Returns the Unicode value of the character at the specified index.
-
Parameters:
-
index
: The position of the character whose Unicode value you want to retrieve.
-
- Example:
let str = "Hello"; console.log(str.charCodeAt(0)); // 72
-
fromCharCode(...codes)
:- Definition: Static method that returns a string created from the specified sequence of Unicode values.
-
Parameters:
-
codes
: One or more Unicode values to convert to characters.
-
- Example:
console.log(String.fromCharCode(72, 101, 108, 108, 111)); // "Hello"
-
match(regex)
:- Definition: Retrieves the matches of a string against a regular expression.
-
Parameters:
-
regex
: A regular expression object to match against the string.
-
- Example:
let str = "Hello World"; let matches = str.match(/o/g); console.log(matches); // ["o", "o"]
-
search(regex)
:- Definition: Executes a search for a match between the string and a regular expression, returning the index of the match.
-
Parameters:
-
regex
: A regular expression object to search for within the string.
-
- Example:
let str = "Hello World"; console.log(str.search(/World/)); // 6
-
startsWith(subStr, start)
:- Definition: Determines whether the string starts with the specified substring.
-
Parameters:
-
subStr
: The substring to search for at the start of the string. -
start
(optional): The position in the string at which to begin searching. Default is 0.
-
- Example:
let str = "Hello World"; console.log(str.startsWith("Hello")); // true
-
endsWith(subStr, length)
:- Definition: Determines whether the string ends with the specified substring.
-
Parameters:
-
subStr
: The substring to search for at the end of the string. -
length
(optional): The length of the string to consider. Default is the full length of the string.
-
- Example:
let str = "Hello World"; // Check if the string ends with "World" console.log(str.endsWith("World")); // true // Check if the string ends with "Hello" console.log(str.endsWith("Hello")); // false // Check if the string ends with "Hello" within the first 5 characters console.log(str.endsWith("Hello", 5)); // true
-
Join Array Elements into String:
- To join array elements into a single string, use the
join()
method. Example:
let arr = ['Hello', 'World']; let str = arr.join(' '); console.log(str); // "Hello World"
- To join array elements into a single string, use the
-
Create String with Multiple Spaces:
- To create a string with multiple spaces, you can use the
repeat()
method. Example:
let spaces = ' '.repeat(5); console.log(spaces); // " "
- To create a string with multiple spaces, you can use the
-
Multi-line Strings in JavaScript:
- Use template literals (backticks) to create multi-line strings. Example:
let multiLineStr = `This is a multi-line string.`; console.log(multiLineStr);
-
Generate All Combinations of a String:
- To generate all combinations of a string, you can use recursion. Example:
function getAllCombinations(str) { let combinations = []; for (let i = 0; i < str.length; i++) { for (let j = i + 1; j < str.length + 1; j++) { combinations.push(str.slice(i, j)); } } return combinations; } console.log(getAllCombinations("abc")); // ["a", "ab", "abc", "b", "bc", "c"]
-
Create Function from String:
- You can create a function from a string using the
new Function()
constructor. Example:
let func = new Function('a', 'b', 'return a + b;'); console.log(func(2, 3)); // 5
- You can create a function from a string using the
-
String Reference:
- For comprehensive details on JavaScript strings, refer to the MDN Web Docs.
-
JS Date:
- JavaScript Date objects represent a single moment in time. You can create a Date object using the
Date
constructor. Example:
let date = new Date(); console.log(date); // Current date and time
- JavaScript Date objects represent a single moment in time. You can create a Date object using the
-
Remove a Character From String in JS:
- To remove a character from a string, use the
replace()
method. Example:
let str = 'Hello'; let newStr = str.replace('e', ''); console.log(newStr); // "Hllo"
- To remove a character from a string, use the
-
Extract a Number from a String using JS:
- To extract a number from a string, use the
match()
method with a regular expression. Example:
let str = 'There are 123 apples'; let num = str.match(/\d+/)[0]; console.log(num); // "123"
- To extract a number from a string, use the