JavaScript Roadmap: 60 Days to Mastery in 2025
Learn JavaScript the Easy, Enjoyable, and Effective Way with support from this practical eBook: JavaScript Mastery eBook JavaScript is not hard. It’s just rarely taught the right way—until now. If you’ve ever felt stuck, overwhelmed, or unsure where to start, this article is your new best friend. This is a complete 60-day learning roadmap designed to take you from beginner to confident JavaScript developer—step by step—with daily goals, code exercises, and mini-projects. No fluff. No guesswork. Just structured, progressive learning backed by the JavaScript Mastery eBook that keeps you consistent and motivated. Why Learn JavaScript in 2025? JavaScript powers nearly every modern website. It’s used for: Frontend frameworks like React, Vue, and Svelte Backend platforms like Node.js Desktop and mobile apps Game development Automation and more It’s the one language you’ll use everywhere. And the best part? It’s learnable. By committing 60 days, you can go from curious beginner to confident creator. The 60-Day JavaScript Roadmap Here’s exactly what you’ll learn each day — broken into 8 clear stages. Week 1: Foundations (Days 1–7) Build your confidence. Lay the groundwork. Day 1: What is JavaScript? Understand how it powers the web. Write your first console.log. Day 2: Setup & Console Choose a code editor. Learn how to run JavaScript in the browser. Day 3: Variables Understand let, const, and var. Know when and why to use each. Day 4: Data Types Dive into strings, numbers, booleans, undefined, and null. Day 5: Operators Learn math, assignment, and comparison operators. Day 6: Conditionals Write if, else, switch statements to make decisions. Day 7: Loops Automate with for, while, and do...while loops. Week 2: Functions, Arrays & Objects (Days 8–14) Write modular, reusable, and logical code. Day 8: Functions Learn function declarations, expressions, and return values. Day 9: Scope & Hoisting Understand how variables behave inside and outside functions. Day 10: Mini Project — Calculator App Apply logic to build your first tool. Day 11: Arrays Store and loop through multiple values. Day 12: Array Methods Master .map(), .filter(), .reduce(), and .forEach(). Day 13: Objects Create structured data using key-value pairs. Day 14: Mini Project — Product List Build a dynamic app using arrays and objects. Week 3: DOM & Interactivity (Days 15–21) Make your websites interactive and responsive. Day 15: DOM Basics Learn how to select and manipulate HTML elements. Day 16: Events Respond to user actions like clicks and inputs. Day 17: DOM Manipulation Add, remove, and change elements dynamically. Day 18: Forms & Validations Capture and clean user input using JavaScript. Day 19: Mini Project — To-Do List Create a task manager with DOM and event handling. Days 20–21: Practice Challenges Reinforce your DOM and interaction knowledge with mini tasks. Week 4: Modern JavaScript (Days 22–28) Learn how professional developers write code today. Day 22: ES6+ Features Arrow functions, let/const, template literals. Day 23: Destructuring & Spread Make your code cleaner and easier to read. Day 24: JavaScript Classes Learn the basics of object-oriented JavaScript. Day 25: Prototypes & this Understand the deeper mechanics of JS. Day 26: Mini Project — Notes App Use classes and OOP to organize your code. Days 27–28: Code Refactoring Practice writing clean, readable, maintainable code. Week 5: APIs & Async (Days 29–35) Fetch and display real-world data from the web. Day 29: Callbacks & Promises Understand async JavaScript the simple way. Day 30: Async/Await Write cleaner asynchronous code. Day 31: Fetch API Make GET requests and handle JSON responses. Days 32–33: Project — Weather App Use a public API to display real-time weather. Days 34–35: Project — News App Build a news reader that fetches live data. Weeks 6–8: Real Projects & Deployment (Days 36–60) Apply everything and build a portfolio. Days 36–37: LocalStorage Store user data in the browser persistently. Day 38: JSON Deep Dive Learn how to send, receive, and store JSON data. Days 39–40: Project — Expense Tracker Build an app with form input, math, and storage. Days 41–43: Mini Projects Quote Generator Clock + Alarm Bookmark Manager Days 44–48: Debugging Mastery Use DevTools, trace errors, and solve problems fast. Days 49–53: Final Projects Movie Search with OMDB API Portfolio Page Pomodoro Productivity Timer Days 54–59: Git & Deployment Learn Git basics. Push to GitHub. Host on Netlify or Vercel. Day 60: Wrap Up & What’s Next Review your projects. Plan for React, TypeScript, or backend (Node.js). You’re Not Alone on This Journey Each of these 60 days is supported by the JavaScript Mastery eBook. This book is your roadmap, your mentor, and your accountability partner. Inside the eB
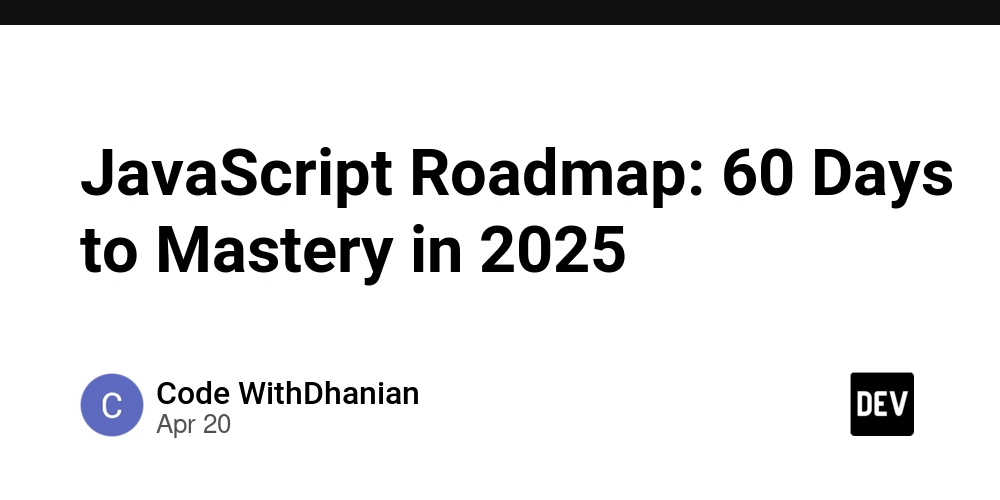
Learn JavaScript the Easy, Enjoyable, and Effective Way
with support from this practical eBook:
JavaScript Mastery eBook
JavaScript is not hard.
It’s just rarely taught the right way—until now.
If you’ve ever felt stuck, overwhelmed, or unsure where to start, this article is your new best friend. This is a complete 60-day learning roadmap designed to take you from beginner to confident JavaScript developer—step by step—with daily goals, code exercises, and mini-projects.
No fluff. No guesswork. Just structured, progressive learning backed by the JavaScript Mastery eBook that keeps you consistent and motivated.
Why Learn JavaScript in 2025?
JavaScript powers nearly every modern website. It’s used for:
- Frontend frameworks like React, Vue, and Svelte
- Backend platforms like Node.js
- Desktop and mobile apps
- Game development
- Automation and more
It’s the one language you’ll use everywhere. And the best part? It’s learnable. By committing 60 days, you can go from curious beginner to confident creator.
The 60-Day JavaScript Roadmap
Here’s exactly what you’ll learn each day — broken into 8 clear stages.
Week 1: Foundations (Days 1–7)
Build your confidence. Lay the groundwork.
Day 1: What is JavaScript?
Understand how it powers the web. Write your firstconsole.log
.Day 2: Setup & Console
Choose a code editor. Learn how to run JavaScript in the browser.Day 3: Variables
Understandlet
,const
, andvar
. Know when and why to use each.Day 4: Data Types
Dive into strings, numbers, booleans, undefined, and null.Day 5: Operators
Learn math, assignment, and comparison operators.Day 6: Conditionals
Writeif
,else
,switch
statements to make decisions.Day 7: Loops
Automate withfor
,while
, anddo...while
loops.
Week 2: Functions, Arrays & Objects (Days 8–14)
Write modular, reusable, and logical code.
Day 8: Functions
Learn function declarations, expressions, and return values.Day 9: Scope & Hoisting
Understand how variables behave inside and outside functions.Day 10: Mini Project — Calculator App
Apply logic to build your first tool.Day 11: Arrays
Store and loop through multiple values.Day 12: Array Methods
Master.map()
,.filter()
,.reduce()
, and.forEach()
.Day 13: Objects
Create structured data using key-value pairs.Day 14: Mini Project — Product List
Build a dynamic app using arrays and objects.
Week 3: DOM & Interactivity (Days 15–21)
Make your websites interactive and responsive.
Day 15: DOM Basics
Learn how to select and manipulate HTML elements.Day 16: Events
Respond to user actions like clicks and inputs.Day 17: DOM Manipulation
Add, remove, and change elements dynamically.Day 18: Forms & Validations
Capture and clean user input using JavaScript.Day 19: Mini Project — To-Do List
Create a task manager with DOM and event handling.Days 20–21: Practice Challenges
Reinforce your DOM and interaction knowledge with mini tasks.
Week 4: Modern JavaScript (Days 22–28)
Learn how professional developers write code today.
Day 22: ES6+ Features
Arrow functions,let
/const
, template literals.Day 23: Destructuring & Spread
Make your code cleaner and easier to read.Day 24: JavaScript Classes
Learn the basics of object-oriented JavaScript.Day 25: Prototypes &
this
Understand the deeper mechanics of JS.Day 26: Mini Project — Notes App
Use classes and OOP to organize your code.Days 27–28: Code Refactoring
Practice writing clean, readable, maintainable code.
Week 5: APIs & Async (Days 29–35)
Fetch and display real-world data from the web.
Day 29: Callbacks & Promises
Understand async JavaScript the simple way.Day 30: Async/Await
Write cleaner asynchronous code.Day 31: Fetch API
Make GET requests and handle JSON responses.Days 32–33: Project — Weather App
Use a public API to display real-time weather.Days 34–35: Project — News App
Build a news reader that fetches live data.
Weeks 6–8: Real Projects & Deployment (Days 36–60)
Apply everything and build a portfolio.
Days 36–37: LocalStorage
Store user data in the browser persistently.Day 38: JSON Deep Dive
Learn how to send, receive, and store JSON data.Days 39–40: Project — Expense Tracker
Build an app with form input, math, and storage.-
Days 41–43: Mini Projects
- Quote Generator
- Clock + Alarm
- Bookmark Manager
Days 44–48: Debugging Mastery
Use DevTools, trace errors, and solve problems fast.-
Days 49–53: Final Projects
- Movie Search with OMDB API
- Portfolio Page
- Pomodoro Productivity Timer
Days 54–59: Git & Deployment
Learn Git basics. Push to GitHub. Host on Netlify or Vercel.Day 60: Wrap Up & What’s Next
Review your projects. Plan for React, TypeScript, or backend (Node.js).
You’re Not Alone on This Journey
Each of these 60 days is supported by the JavaScript Mastery eBook. This book is your roadmap, your mentor, and your accountability partner.
Inside the eBook:
- 60+ topics explained clearly and concisely
- Copy-paste runnable code snippets
- Mini projects, real-world apps, and exercises
- Clean code examples and developer best practices
- Structured for daily progress
Ready to Build Real Projects and Master JavaScript?
In 60 days, you’ll go from:
- “I’m not sure how JavaScript works” to
- “I just built a full-featured web app from scratch.”
So take that first step.
Start learning JavaScript the right way.
Grab the eBook:
codewithdhanian.gumroad.com/l/jjpifd
Make 2025 the year you master JavaScript — one day at a time.