Java Tutorial-What is Java
Java is a high-level, object-oriented programming language used to build applications across platforms—from web and mobile apps to enterprise software. It is known for its Write Once, Run Anywhere capability, meaning code written in Java can run on any device that supports the Java Virtual Machine (JVM). Keywords: high-level,object-oriented,across platforms,write once run everywhere, JVM. 1. What is Java Used For? Develop mobile apps, desktop apps, web apps, web servers, games, and enterprise-level systems. Java's syntax is similar to C/C++. 1.1 Java File Naming Conventions The file name must match with the public class name. Java is case sensitive, so Geeks.java is not equal to geeks.java. If a file has no "public" class, the file name can be anything but it should be matched with the primary class name. 2. Java Basic Java basics form the foundation of your programming journey, covering essential concepts like syntax, data types, variables, loops, and conditionals. 3. Java Methods Java methods are reusable blocks of code that perform specific tasks and help organize your program. 4. Java Arrays Java arrays are containers that store multiple values of the same data type in a single variable. They provide an efficient way to** manage and access collections of data** using index-based positions: 5. Java Strings represent sequences of characters and are widely used in text processing. They are immutable, meaning once created, their values cannot be changed: 6. Java OOPs Concepts Java follows the Object-Oriented Programming (OOP) paradigm, which organizes code into classes and objects. Core OOP principles like inheritance, encapsulation, polymorphism, and abstraction make Java modular and scalable: 7. Java Interfaces Java interfaces define a contract that classes must follow, specifying method signatures without implementations. They enable abstraction and support multiple inheritance in Java through a clean, structured approach: 8. Java Collections Java Collections provide a framework for storing and manipulating groups of objects efficiently. It includes interfaces like List, Set, and Map, along with classes like ArrayList, HashSet, and HashMap: 9. Java Exception Handling Java Exception Handling is a mechanism to handle runtime errors, ensuring the program runs smoothly without crashing. It uses keywords like try, catch, throw, throws, and finally to manage exceptions: 10. Java Multithreading Java Multithreading allows concurrent execution of two or more threads, enabling efficient CPU utilization and faster program performance. It is commonly used for tasks like parallel processing and responsive applications: 11. Java File Handling Java File Handling enables programs to create, read, write, and manipulate files stored on the system. It uses classes from the java.io and java.nio packages for efficient file operations: 12. Java Streams and Lambda Expressions Java Streams and Lambda Expressions simplify data processing by enabling functional-style operations on collections. Lambdas provide concise syntax for anonymous functions, while Streams allow efficient filtering, mapping, and reduction of data: 13. Java IO Java IO (Input/Output) provides a set of classes and streams to read and write data from various sources like files, consoles, and network connections. It is part of the java.io package and supports both byte and character streams: 14. Java Synchronization Java Synchronization is used to control access to shared resources in multithreaded environments. It ensures that only one thread can access a critical section at a time, preventing data inconsistency: 15. Java Regex Java Regex (Regular Expressions) allows **pattern matching **and text manipulation using the java.util.regex package. It is powerful for validating, searching, and replacing strings based on specific patterns: 16. Java Networking Java Networking enables communication between devices over a network using classes from the java.net package. It supports protocols like TCP and UDP for building client-server applications and data exchange: 17. Java Memory Allocation Java Memory Allocation refers to how memory is assigned to variables, objects, and classes during program execution. It involves stack and heap memory, with the JVM managing allocation and garbage collection automatically:
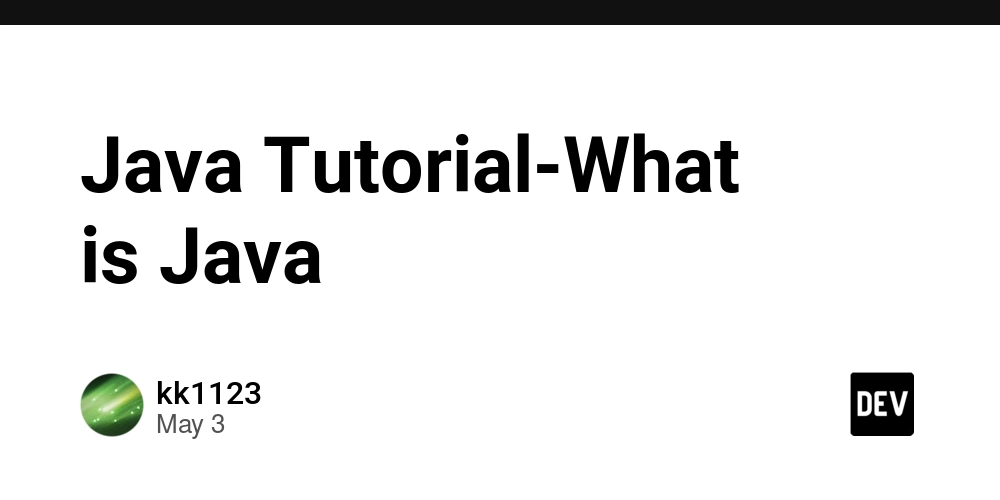
Java is a high-level, object-oriented programming language used to build applications across platforms—from web and mobile apps to enterprise software. It is known for its Write Once, Run Anywhere capability, meaning code written in Java can run on any device that supports the Java Virtual Machine (JVM).
Keywords: high-level,object-oriented,across platforms,write once run everywhere, JVM.
1. What is Java Used For?
- Develop mobile apps, desktop apps, web apps, web servers, games, and enterprise-level systems.
- Java's syntax is similar to C/C++.
1.1 Java File Naming Conventions
- The file name must match with the public class name.
- Java is case sensitive, so Geeks.java is not equal to geeks.java.
- If a file has no "public" class, the file name can be anything but it should be matched with the primary class name.
2. Java Basic
Java basics form the foundation of your programming journey, covering essential concepts like syntax, data types, variables, loops, and conditionals.
3. Java Methods
Java methods are reusable blocks of code that perform specific tasks and help organize your program.
4. Java Arrays
Java arrays are containers that store multiple values of the same data type in a single variable. They provide an efficient way to** manage and access collections of data** using index-based positions:
5. Java Strings
represent sequences of characters and are widely used in text processing. They are immutable, meaning once created, their values cannot be changed:
6. Java OOPs Concepts
Java follows the Object-Oriented Programming (OOP) paradigm, which organizes code into classes and objects. Core OOP principles like inheritance, encapsulation, polymorphism, and abstraction make Java modular and scalable:
7. Java Interfaces
Java interfaces define a contract that classes must follow, specifying method signatures without implementations. They enable abstraction and support multiple inheritance in Java through a clean, structured approach:
8. Java Collections
Java Collections provide a framework for storing and manipulating groups of objects efficiently. It includes interfaces like List, Set, and Map, along with classes like ArrayList, HashSet, and HashMap:
9. Java Exception Handling
Java Exception Handling is a mechanism to handle runtime errors, ensuring the program runs smoothly without crashing. It uses keywords like try, catch, throw, throws, and finally to manage exceptions:
10. Java Multithreading
Java Multithreading allows concurrent execution of two or more threads, enabling efficient CPU utilization and faster program performance. It is commonly used for tasks like parallel processing and responsive applications:
11. Java File Handling
Java File Handling enables programs to create, read, write, and manipulate files stored on the system. It uses classes from the java.io and java.nio packages for efficient file operations:
12. Java Streams and Lambda Expressions
Java Streams and Lambda Expressions simplify data processing by enabling functional-style operations on collections. Lambdas provide concise syntax for anonymous functions, while Streams allow efficient filtering, mapping, and reduction of data:
13. Java IO
Java IO (Input/Output) provides a set of classes and streams to read and write data from various sources like files, consoles, and network connections. It is part of the java.io package and supports both byte and character streams:
14. Java Synchronization
Java Synchronization is used to control access to shared resources in multithreaded environments. It ensures that only one thread can access a critical section at a time, preventing data inconsistency:
15. Java Regex
Java Regex (Regular Expressions) allows **pattern matching **and text manipulation using the java.util.regex package. It is powerful for validating, searching, and replacing strings based on specific patterns:
16. Java Networking
Java Networking enables communication between devices over a network using classes from the java.net package. It supports protocols like TCP and UDP for building client-server applications and data exchange:
17. Java Memory Allocation
Java Memory Allocation refers to how memory is assigned to variables, objects, and classes during program execution. It involves stack and heap memory, with the JVM managing allocation and garbage collection automatically: