How to Use a Local Gradle Project in Another Gradle Project?
When working with multiple Gradle projects, especially in a scenario where one project depends on another, you might encounter the need to test and develop features across both projects simultaneously. In this guide, I will explain how to use a local Gradle project (Project B) within another Gradle project (Project A) without the need to push changes to remote and release a new version each time. Understanding the Problem In multirepo architectures, the standard approach would be to update dependencies in Project A after releasing a new version of Project B. This process can slow down development, as it involves pushes to remote repositories and CI/CD workflows. Instead, being able to reference a local version of Project B while you are still developing features allows for quicker feedback and iteration without committing intermediate changes. Setting Up Local Dependency with Gradle Step 1: Include Project B as a Composite Build One of the most effective ways to include a local version of Project B into Project A is via composite builds. This involves referencing the local project directly in the settings of Project A. In your Project A directory, create or modify the settings.gradle file to include Project B: includeBuild '../path/to/project-b' // specify the relative path to Project B year inline Block each bean import statement This small addition tells Gradle that you want to include Project B as part of the build process for Project A. Step 2: Modify build.gradle in Project A In your build.gradle file for Project A, modify the dependency definition to reference Project B without specifying a version. Instead of: implementation 'com.something.project-b:project-b:1.0.0' You should change it to: implementation 'com.something.project-b:project-b' This change means that Gradle will now look for the local composite build version of Project B instead of a specific version from a remote repository. Step 3: Verify Dependency Management Run the following command to ensure that your configuration is successful: ./gradlew dependencies This command lists all the dependencies resolved for your project, including transitive dependencies from Project B. Make sure that all required dependencies are included, confirming they are correctly picked up from the local version. Step 4: Implement and Test Changes Now that your local dependency is set up, you can make changes in Project B. With Project A configured to use the local version of Project B, any changes you make will take effect immediately when you build or run Project A. This allows you to test features across both projects seamlessly. Advanced Considerations Testing Changes Without Pushing This setup allows you to work on features across both projects without needing to deploy changes. Once you are satisfied with the functionality, you can publish to the remote repository and follow the standard CI/CD process. Upgrading Gradle Version It is important to keep in mind that you mentioned using Gradle 7.5. Ensure that your Gradle version is up to date, as newer versions provide better support and more features that can be leveraged in multi-project builds. Check with: gradle --version Multiple Modules Dependency Resolution Should Project B have multiple modules with their own dependencies, this composite build setup will respect that. Gradle's dependency resolution will handle all transitive dependencies correctly, so there’s no need to worry about missing libraries in your local development environment. Frequently Asked Questions Can I use this method for production builds? Yes, composite builds are typically used for local development. For production builds, you would revert back to using the versions from your remote repository. What happens to existing consumers of Project B when I switch to local? When you switch to local development, only your instance of Project A will use the local Project B. Other consumers will still reference the remote version unless they also change their dependency configuration. How does this affect build performance? There might be slight changes in performance depending on how you structure your projects, but overall, this method is efficient for local development and testing. In conclusion, using composite builds in Gradle provides a powerful way to manage local dependencies without the hassle of publishing changes to remote repositories. This setup is ideal for reducing development friction and increasing productivity when multiple repositories are involved.
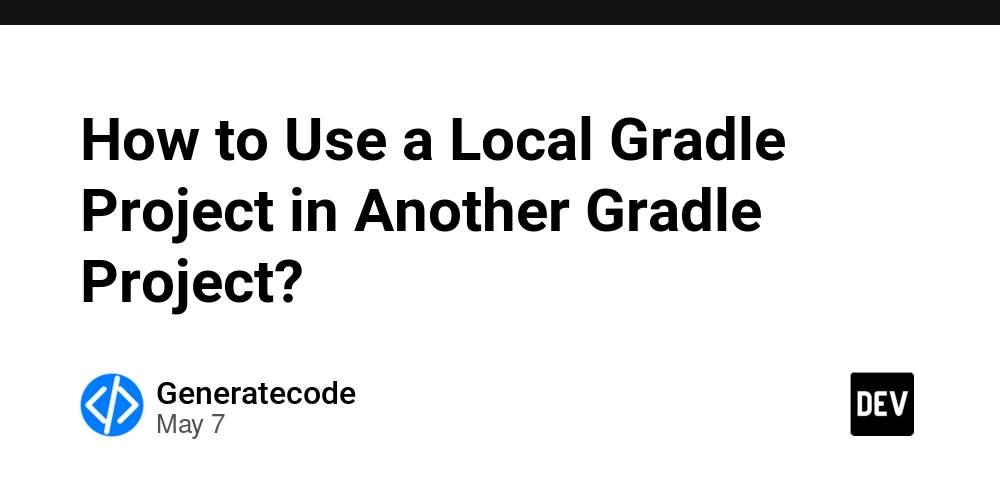
When working with multiple Gradle projects, especially in a scenario where one project depends on another, you might encounter the need to test and develop features across both projects simultaneously. In this guide, I will explain how to use a local Gradle project (Project B) within another Gradle project (Project A) without the need to push changes to remote and release a new version each time.
Understanding the Problem
In multirepo architectures, the standard approach would be to update dependencies in Project A after releasing a new version of Project B. This process can slow down development, as it involves pushes to remote repositories and CI/CD workflows. Instead, being able to reference a local version of Project B while you are still developing features allows for quicker feedback and iteration without committing intermediate changes.
Setting Up Local Dependency with Gradle
Step 1: Include Project B as a Composite Build
One of the most effective ways to include a local version of Project B into Project A is via composite builds. This involves referencing the local project directly in the settings of Project A.
- In your Project A directory, create or modify the
settings.gradle
file to include Project B:
includeBuild '../path/to/project-b' // specify the relative path to Project B
year inline Block each bean import statement
This small addition tells Gradle that you want to include Project B as part of the build process for Project A.
Step 2: Modify build.gradle
in Project A
In your build.gradle
file for Project A, modify the dependency definition to reference Project B without specifying a version. Instead of:
implementation 'com.something.project-b:project-b:1.0.0'
You should change it to:
implementation 'com.something.project-b:project-b'
This change means that Gradle will now look for the local composite build version of Project B instead of a specific version from a remote repository.
Step 3: Verify Dependency Management
Run the following command to ensure that your configuration is successful:
./gradlew dependencies
This command lists all the dependencies resolved for your project, including transitive dependencies from Project B. Make sure that all required dependencies are included, confirming they are correctly picked up from the local version.
Step 4: Implement and Test Changes
Now that your local dependency is set up, you can make changes in Project B. With Project A configured to use the local version of Project B, any changes you make will take effect immediately when you build or run Project A. This allows you to test features across both projects seamlessly.
Advanced Considerations
Testing Changes Without Pushing
This setup allows you to work on features across both projects without needing to deploy changes. Once you are satisfied with the functionality, you can publish to the remote repository and follow the standard CI/CD process.
Upgrading Gradle Version
It is important to keep in mind that you mentioned using Gradle 7.5. Ensure that your Gradle version is up to date, as newer versions provide better support and more features that can be leveraged in multi-project builds. Check with:
gradle --version
Multiple Modules Dependency Resolution
Should Project B have multiple modules with their own dependencies, this composite build setup will respect that. Gradle's dependency resolution will handle all transitive dependencies correctly, so there’s no need to worry about missing libraries in your local development environment.
Frequently Asked Questions
Can I use this method for production builds?
Yes, composite builds are typically used for local development. For production builds, you would revert back to using the versions from your remote repository.
What happens to existing consumers of Project B when I switch to local?
When you switch to local development, only your instance of Project A will use the local Project B. Other consumers will still reference the remote version unless they also change their dependency configuration.
How does this affect build performance?
There might be slight changes in performance depending on how you structure your projects, but overall, this method is efficient for local development and testing.
In conclusion, using composite builds in Gradle provides a powerful way to manage local dependencies without the hassle of publishing changes to remote repositories. This setup is ideal for reducing development friction and increasing productivity when multiple repositories are involved.