How to handle nested reactive properties in reactive programming?
When building an application or program using the concepts of reactive programming, how does this work for nested reactive properties? I have an example using Swift, ReactiveKit, and Bond, however the concepts apply to any reactive programming setup. class User { var firstName: Observable init(firstName: String) { self.firstName = Observable(firstName) } } class ViewController: UIViewController { var user: Observable = Observable(User.init(firstName: "Bob")) @IBOutlet let label: UILabel! override func viewDidLoad() { user.firstName.bind(to: label) } @IBAction func updateUser() { WebService.retrieveUserFromServer { [weak self] user in self?.user.value = user } } } So in the example above, I want the label to be updated whenever the user or user.firstName is updated. But currently, if updateUser gets called, and a new user instance is passed in, the bind to the label doesn't work anymore. So in this example, the firstName can be updated directly, or the entire user instance can be updated. Of course there is a way to maybe use some combine functions to add a listener for when user gets updated or user.firstName gets updated and handle it then. But that gets extremely messy very quickly, especially as you add more properties to User. How are you supposed to handle these types of cases in reactive programming? Where you have a parent property that is reactive, and properties that are reactive within that class, and you want to react based on either the parent being updated, or any of the children properties being updated.
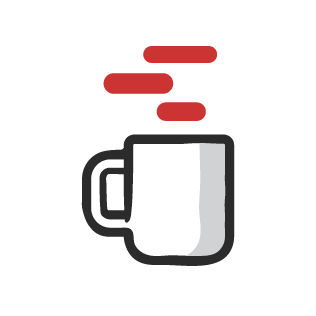
When building an application or program using the concepts of reactive programming, how does this work for nested reactive properties?
I have an example using Swift, ReactiveKit, and Bond, however the concepts apply to any reactive programming setup.
class User {
var firstName: Observable
init(firstName: String) {
self.firstName = Observable(firstName)
}
}
class ViewController: UIViewController {
var user: Observable = Observable(User.init(firstName: "Bob"))
@IBOutlet let label: UILabel!
override func viewDidLoad() {
user.firstName.bind(to: label)
}
@IBAction func updateUser() {
WebService.retrieveUserFromServer { [weak self] user in
self?.user.value = user
}
}
}
So in the example above, I want the label to be updated whenever the user
or user.firstName
is updated. But currently, if updateUser
gets called, and a new user
instance is passed in, the bind
to the label doesn't work anymore.
So in this example, the firstName
can be updated directly, or the entire user
instance can be updated. Of course there is a way to maybe use some combine functions to add a listener for when user
gets updated or user.firstName
gets updated and handle it then. But that gets extremely messy very quickly, especially as you add more properties to User
.
How are you supposed to handle these types of cases in reactive programming? Where you have a parent property that is reactive, and properties that are reactive within that class, and you want to react based on either the parent being updated, or any of the children properties being updated.