How to find ip address of the FPGA board connected through MATLAB?
To find the IP address of an FPGA board connected through MATLAB, you typically need to establish communication between MATLAB and the FPGA board using a supported interface (e.g., Ethernet, JTAG, or PCIe). The exact steps depend on the FPGA board, the communication protocol, and the tools you're using. Below is a general guide for finding the IP address of an FPGA board connected to MATLAB: 1. Ensure the FPGA Board is Connected Connect the FPGA board to your computer via Ethernet, JTAG, or another supported interface. Power on the FPGA board and ensure it is properly configured for network communication (if using Ethernet). 2. Use MATLAB Support Packages MATLAB provides support packages for FPGA boards like Xilinx and Intel (Altera). These packages often include functions to detect and communicate with the FPGA board. A. Install FPGA Support Package Open MATLAB. Go to the Add-Ons menu and search for the FPGA support package for your board (e.g., "Xilinx FPGA Support Package" or "Intel FPGA Support Package"). Install the support package. B. Detect the FPGA Board Use MATLAB commands to detect the FPGA board and its IP address. Example for Xilinx boards: matlab % List all FPGA boards connected to the host hw = xilinx.fpga.Board.listAvailableBoards(); % Display the IP address of the first detected board if ~isempty(hw) disp(hw(1).IPAddress); else disp('No FPGA board detected.'); end 3. Use System Commands to Find the IP Address If the FPGA board is connected via Ethernet, you can use system commands to find its IP address. A. Ping the FPGA Board Use the ping command to identify the FPGA board's IP address. Example: matlab % Ping the FPGA board (replace 'fpga_hostname' with the board's hostname) [status, result] = system('ping fpga_hostname'); % Display the result disp(result); B. Scan the Network Use tools like arp or nmap to scan the network for connected devices. Example: matlab % Run arp command to list devices on the network [status, result] = system('arp -a'); % Display the result disp(result); 4. Use FPGA Vendor Tools Most FPGA vendors provide tools to detect and configure FPGA boards. These tools can often display the IP address of the connected board. A. Xilinx Vivado Open Vivado and connect to the FPGA board. Use the Hardware Manager to view the board's IP address. B. Intel Quartus Open Quartus and connect to the FPGA board. Use the System Console or JTAG Chain Debugger to view the board's IP address. 5. Use MATLAB TCP/IP Functions If the FPGA board is configured as a TCP/IP server, you can use MATLAB's TCP/IP functions to establish a connection and retrieve the IP address. Example: matlab % Create a TCP/IP object tcpipObj = tcpip('fpga_hostname', 7); % Replace 'fpga_hostname' with the board's hostname % Open the connection fopen(tcpipObj); % Get the remote IP address ipAddress = tcpipObj.RemoteHost; % Display the IP address disp(ipAddress); % Close the connection fclose(tcpipObj); 6. Check the FPGA Board's Documentation Refer to the FPGA board's user manual or documentation for specific instructions on finding its IP address. Some boards have a default IP address or provide a way to configure it via a web interface or serial connection. 7. Example: Xilinx Zynq Board with MATLAB If you're using a Xilinx Zynq board with MATLAB: Connect the board to your computer via Ethernet. Use the xilinx.fpga.Board.listAvailableBoards function to detect the board and retrieve its IP address. Alternatively, use the Xilinx Vivado Hardware Manager to find the IP address. By following these steps, you can find the IP address of an FPGA board connected through MATLAB and establish communication for further development and testing.
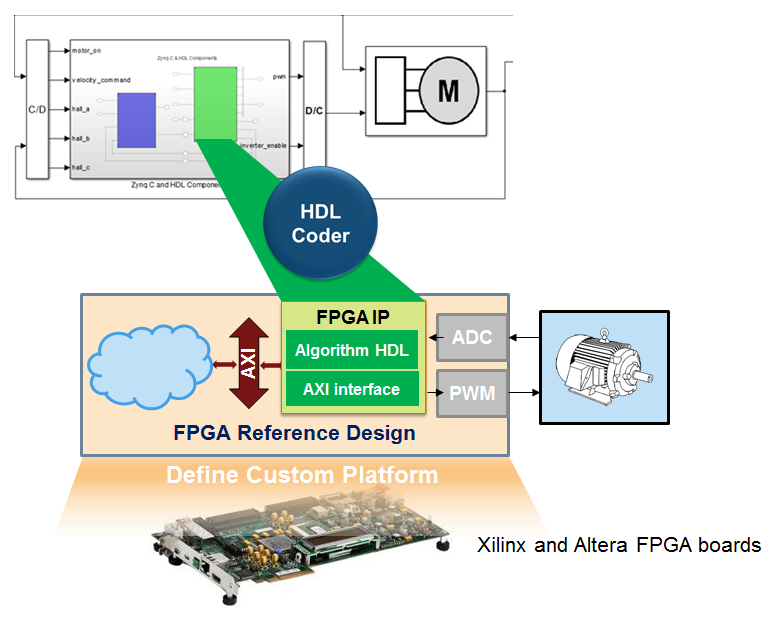
To find the IP address of an FPGA board connected through MATLAB, you typically need to establish communication between MATLAB and the FPGA board using a supported interface (e.g., Ethernet, JTAG, or PCIe). The exact steps depend on the FPGA board, the communication protocol, and the tools you're using. Below is a general guide for finding the IP address of an FPGA board connected to MATLAB:
1. Ensure the FPGA Board is Connected
- Connect the FPGA board to your computer via Ethernet, JTAG, or another supported interface.
- Power on the FPGA board and ensure it is properly configured for network communication (if using Ethernet).
2. Use MATLAB Support Packages
MATLAB provides support packages for FPGA boards like Xilinx and Intel (Altera). These packages often include functions to detect and communicate with the FPGA board.
A. Install FPGA Support Package
- Open MATLAB.
- Go to the Add-Ons menu and search for the FPGA support package for your board (e.g., "Xilinx FPGA Support Package" or "Intel FPGA Support Package").
- Install the support package.
B. Detect the FPGA Board
Use MATLAB commands to detect the FPGA board and its IP address.
Example for Xilinx boards:
matlab
% List all FPGA boards connected to the host
hw = xilinx.fpga.Board.listAvailableBoards();
% Display the IP address of the first detected board
if ~isempty(hw)
disp(hw(1).IPAddress);
else
disp('No FPGA board detected.');
end
3. Use System Commands to Find the IP Address
If the FPGA board is connected via Ethernet, you can use system commands to find its IP address.
A. Ping the FPGA Board
Use the ping command to identify the FPGA board's IP address.
Example:
matlab
% Ping the FPGA board (replace 'fpga_hostname' with the board's hostname)
[status, result] = system('ping fpga_hostname');
% Display the result
disp(result);
B. Scan the Network
Use tools like arp or nmap to scan the network for connected devices.
Example:
matlab
% Run arp command to list devices on the network
[status, result] = system('arp -a');
% Display the result
disp(result);
4. Use FPGA Vendor Tools
Most FPGA vendors provide tools to detect and configure FPGA boards. These tools can often display the IP address of the connected board.
A. Xilinx Vivado
- Open Vivado and connect to the FPGA board.
- Use the Hardware Manager to view the board's IP address.
B. Intel Quartus
- Open Quartus and connect to the FPGA board.
- Use the System Console or JTAG Chain Debugger to view the board's IP address.
5. Use MATLAB TCP/IP Functions
If the FPGA board is configured as a TCP/IP server, you can use MATLAB's TCP/IP functions to establish a connection and retrieve the IP address.
Example:
matlab
% Create a TCP/IP object
tcpipObj = tcpip('fpga_hostname', 7); % Replace 'fpga_hostname' with the board's hostname
% Open the connection
fopen(tcpipObj);
% Get the remote IP address
ipAddress = tcpipObj.RemoteHost;
% Display the IP address
disp(ipAddress);
% Close the connection
fclose(tcpipObj);
6. Check the FPGA Board's Documentation
Refer to the FPGA board's user manual or documentation for specific instructions on finding its IP address. Some boards have a default IP address or provide a way to configure it via a web interface or serial connection.
7. Example: Xilinx Zynq Board with MATLAB
If you're using a Xilinx Zynq board with MATLAB:
Connect the board to your computer via Ethernet.
Use the xilinx.fpga.Board.listAvailableBoards function to detect the board and retrieve its IP address.
Alternatively, use the Xilinx Vivado Hardware Manager to find the IP address.
By following these steps, you can find the IP address of an FPGA board connected through MATLAB and establish communication for further development and testing.