How to Create a Simple Key Management System in Lua
Managing keys for your Lua scripts can be crucial for ensuring security and functionality, especially if you're looking to control access without relying on expensive or complex services. In this article, we'll guide you through creating a simple key management system in Lua that enables you to generate, manage, and validate keys easily. Understanding Key Management in Lua Key management in programming is essential for controlling who has access to your application or script's features. As a beginner, you might struggle with various third-party solutions that can be confusing or costly. By developing your own key management system in Lua, you can tailor it to your needs without unnecessary complexity. Why You Need a Custom Key Management System Cost-Effective: Instead of paying for services like Luarmor or Panda Auth, a custom Lua solution allows you to manage keys for free. Simplicity: Many existing systems have steep learning curves. A simple system provides ease of use. Security: Managing your keys yourself allows for better control over their security and access. Now that we understand the need for a key management system, let’s dive into how to build one. Step 1: Setting Up Your Environment Before writing your Lua script, ensure you have the correct development environment set up. Make sure Lua is installed on your machine and that you have an appropriate IDE or text editor. Step 2: Basic Structure for Key Management Here’s a simple Lua script that introduces key generation and management: local KeyManager = {} KeyManager.keys = {} -- Function to generate a new key function KeyManager:generateKey() local key = "key_" .. tostring(math.random(1000, 9999)) self.keys[key] = true -- Store key in the table d return key end -- Function to validate a key function KeyManager:validateKey(key) return self.keys[key] == true -- Return if the key exists end -- Function to revoke a key function KeyManager:revokeKey(key) self.keys[key] = nil -- Remove the key from the table end -- Example Usage local newKey = KeyManager:generateKey() print("Generated Key: " .. newKey) print("Is Key Valid: " .. tostring(KeyManager:validateKey(newKey))) KeyManager:revokeKey(newKey) print("Is Key Valid after revocation: " .. tostring(KeyManager:validateKey(newKey))) Explanation of the Code Generate Key: This function creates a unique key using a random number and stores it in a table. Validate Key: Check if the given key exists in the keys table. Revoke Key: Remove the key from the management system, protecting your script against unauthorized access. Step 3: Enhancements for Better Management Storing Generated Keys To improve your key management, consider using a more persistent method to store keys, like saving them to a file. This way, keys will remain available even after your script stops running. Here’s an example of how to save keys to a file: function KeyManager:saveKeysToFile(filename) local file = io.open(filename, "w") for key in pairs(self.keys) do file:write(key .. '\n') end file:close() end function KeyManager:loadKeysFromFile(filename) local file = io.open(filename, "r") if file then for line in file:lines() do self.keys[line] = true end file:close() end end Example Usage KeyManager:loadKeysFromFile("keys.txt") -- Load existing keys from a file print("Is Key Valid: " .. tostring(KeyManager:validateKey(newKey))) KeyManager:saveKeysToFile("keys.txt") -- Save current keys to a file Frequently Asked Questions What is a key management system? A key management system is a way to generate and validate keys that control access to applications or functionalities. Why use Lua for key management? Lua is lightweight, easy to learn, and offers flexibility for scripting, making it suitable for custom solutions like key management. Can I extend this system? Yes, you can add features like key expiration, user assignment, and logging to enhance your key management system. Conclusion By following this guide, you can create a straightforward key management system in Lua that meets your needs without relying on third-party services. As you continue to learn Lua and development, you can expand the system’s capabilities, ensuring it remains secure and functional. Feel free to play around with the code and customize it as per your requirements. Happy coding!
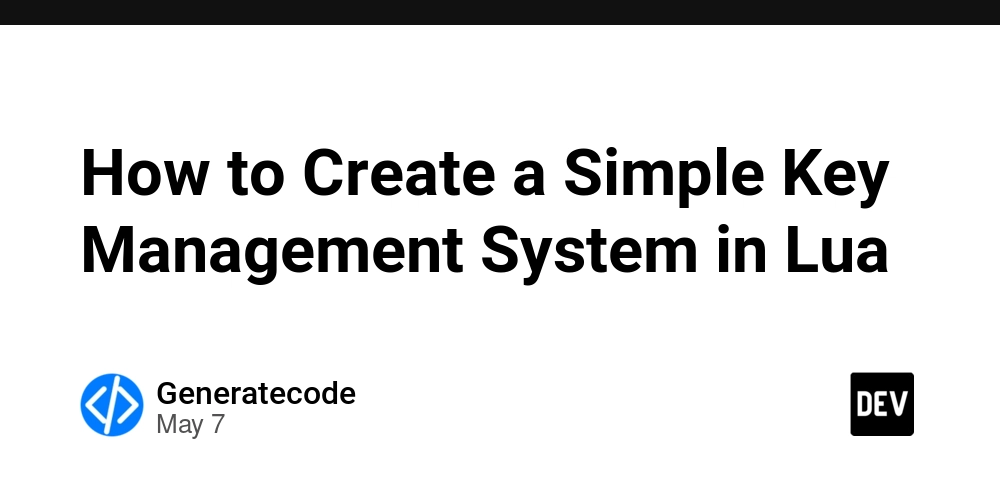
Managing keys for your Lua scripts can be crucial for ensuring security and functionality, especially if you're looking to control access without relying on expensive or complex services. In this article, we'll guide you through creating a simple key management system in Lua that enables you to generate, manage, and validate keys easily.
Understanding Key Management in Lua
Key management in programming is essential for controlling who has access to your application or script's features. As a beginner, you might struggle with various third-party solutions that can be confusing or costly. By developing your own key management system in Lua, you can tailor it to your needs without unnecessary complexity.
Why You Need a Custom Key Management System
- Cost-Effective: Instead of paying for services like Luarmor or Panda Auth, a custom Lua solution allows you to manage keys for free.
- Simplicity: Many existing systems have steep learning curves. A simple system provides ease of use.
- Security: Managing your keys yourself allows for better control over their security and access.
Now that we understand the need for a key management system, let’s dive into how to build one.
Step 1: Setting Up Your Environment
Before writing your Lua script, ensure you have the correct development environment set up. Make sure Lua is installed on your machine and that you have an appropriate IDE or text editor.
Step 2: Basic Structure for Key Management
Here’s a simple Lua script that introduces key generation and management:
local KeyManager = {}
KeyManager.keys = {}
-- Function to generate a new key
function KeyManager:generateKey()
local key = "key_" .. tostring(math.random(1000, 9999))
self.keys[key] = true -- Store key in the table
d return key
end
-- Function to validate a key
function KeyManager:validateKey(key)
return self.keys[key] == true -- Return if the key exists
end
-- Function to revoke a key
function KeyManager:revokeKey(key)
self.keys[key] = nil -- Remove the key from the table
end
-- Example Usage
local newKey = KeyManager:generateKey()
print("Generated Key: " .. newKey)
print("Is Key Valid: " .. tostring(KeyManager:validateKey(newKey)))
KeyManager:revokeKey(newKey)
print("Is Key Valid after revocation: " .. tostring(KeyManager:validateKey(newKey)))
Explanation of the Code
- Generate Key: This function creates a unique key using a random number and stores it in a table.
- Validate Key: Check if the given key exists in the keys table.
- Revoke Key: Remove the key from the management system, protecting your script against unauthorized access.
Step 3: Enhancements for Better Management
Storing Generated Keys
To improve your key management, consider using a more persistent method to store keys, like saving them to a file. This way, keys will remain available even after your script stops running.
Here’s an example of how to save keys to a file:
function KeyManager:saveKeysToFile(filename)
local file = io.open(filename, "w")
for key in pairs(self.keys) do
file:write(key .. '\n')
end
file:close()
end
function KeyManager:loadKeysFromFile(filename)
local file = io.open(filename, "r")
if file then
for line in file:lines() do
self.keys[line] = true
end
file:close()
end
end
Example Usage
KeyManager:loadKeysFromFile("keys.txt") -- Load existing keys from a file
print("Is Key Valid: " .. tostring(KeyManager:validateKey(newKey)))
KeyManager:saveKeysToFile("keys.txt") -- Save current keys to a file
Frequently Asked Questions
What is a key management system?
A key management system is a way to generate and validate keys that control access to applications or functionalities.
Why use Lua for key management?
Lua is lightweight, easy to learn, and offers flexibility for scripting, making it suitable for custom solutions like key management.
Can I extend this system?
Yes, you can add features like key expiration, user assignment, and logging to enhance your key management system.
Conclusion
By following this guide, you can create a straightforward key management system in Lua that meets your needs without relying on third-party services. As you continue to learn Lua and development, you can expand the system’s capabilities, ensuring it remains secure and functional.
Feel free to play around with the code and customize it as per your requirements. Happy coding!