How to Build an AI-Powered Website Generator Like Lovable.ai
In today's fast-moving digital landscape, AI-powered website builders like Lovable.ai are revolutionizing how we create web experiences. These platforms allow users to generate complete websites just by describing what they want in natural language. In this post, I'll walk through how you could build your own AI website generator. Understanding the Architecture An AI website generator typically has three core components: Prompt Processing Engine - Interprets user input AI Generation Layer - Creates content and design Website Compiler - Turns AI output into functional code Here's a high-level architecture: User Prompt → AI Processing → Content Generation → Design Generation → Code Compilation → Deployable Website Building the Prompt Processing Engine The first step is understanding user intent from their natural language prompts. You'll want to: # Example using OpenAI's API for prompt understanding import openai def analyze_prompt(user_prompt): response = openai.ChatCompletion.create( model="gpt-4", messages=[ {"role": "system", "content": "You are a website design assistant. Analyze this prompt and extract: business type, desired features, style preferences, and any specific content mentioned."}, {"role": "user", "content": user_prompt} ] ) return response.choices[0].message.content This helps break down vague requests like "I need a website for my bakery" into structured data your system can work with. Generating Website Content With the analyzed prompt, you can now generate appropriate content: def generate_website_content(business_details): response = openai.ChatCompletion.create( model="gpt-4", messages=[ {"role": "system", "content": f"Generate website content for a {business_details['type']} business. Include homepage text, about page, and services/products."}, {"role": "user", "content": business_details} ] ) return parse_content(response.choices[0].message.content) Creating the Design For design generation, you have several options: Template Selection: Use AI to pick from pre-made templates CSS Generation: Have AI write custom CSS Visual Generation: Use models like DALL·E for design mockups // Example using AI to select a template function selectTemplate(businessType, stylePreferences) { // This would analyze your template library // and return the best match based on AI analysis } Compiling the Website Now combine everything into actual code. You could: Generate HTML/CSS directly Use a framework like React/Vue Output to a site builder format (Webflow, WordPress) def compile_to_html(content, design_spec): # This would take the generated content and design # and assemble it into proper HTML/CSS return f""" {design_spec['css']} {content['homepage']} """ Deployment Options Finally, automate deployment to: Vercel/Netlify for static sites AWS/GCP for more complex apps Custom hosting solutions // Example using Netlify's API const deployToNetlify = async (siteFiles) => { const response = await fetch('https://api.netlify.com/api/v1/sites', { method: 'POST', headers: { 'Authorization': `Bearer ${process.env.NETLIFY_TOKEN}`, 'Content-Type': 'application/zip' }, body: siteFiles }); return response.json(); }; Enhancing Your Generator To make your platform stand out like Lovable.ai, consider adding: Multi-prompt refinement: Let users iteratively improve their site AI-powered editing: "Make it more modern" should regenerate accordingly Component library: Allow adding specific elements (contact forms, galleries) Personalization: Use AI to tailor content to target audience Challenges to Consider Building an AI website generator isn't without hurdles: Quality Control: AI can produce inconsistent results Specificity: Generic prompts lead to generic websites Technical Limitations: Complex functionality still requires manual work Cost: Running AI at scale can be expensive Tech Stack Suggestions Frontend: Next.js, Vue, or Svelte AI Services: OpenAI API, Anthropic, or self-hosted LLMs Design: Tailwind CSS, AI-generated CSS, or template libraries Backend: Node.js, Python, or Go Hosting: Vercel, AWS, or specialized AI platforms Getting Started If you want to experiment with building your own version: Start with a simple single-page generator Use OpenAI's API for content creation Build a small library of templates Gradually add more complex features The key is iterative development - start small and expand based on what works.
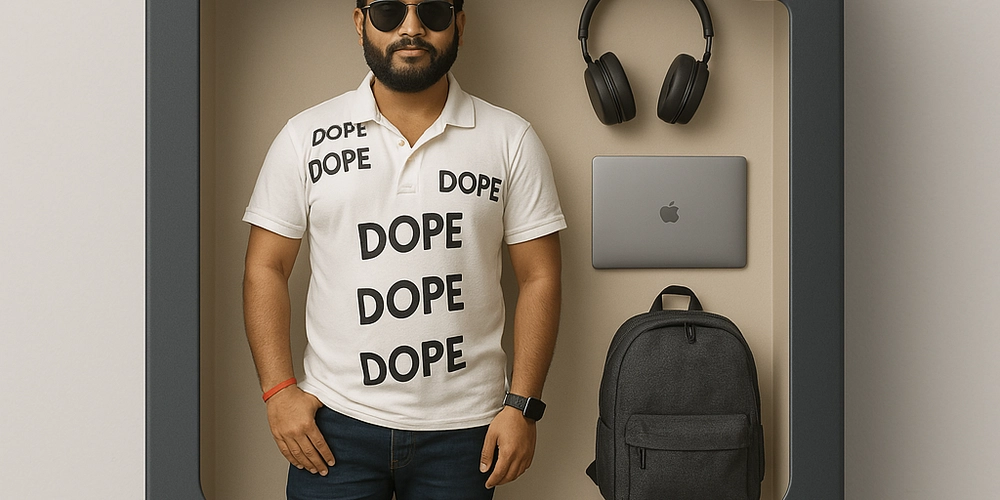
In today's fast-moving digital landscape, AI-powered website builders like Lovable.ai are revolutionizing how we create web experiences. These platforms allow users to generate complete websites just by describing what they want in natural language. In this post, I'll walk through how you could build your own AI website generator.
Understanding the Architecture
An AI website generator typically has three core components:
- Prompt Processing Engine - Interprets user input
- AI Generation Layer - Creates content and design
- Website Compiler - Turns AI output into functional code
Here's a high-level architecture:
User Prompt → AI Processing → Content Generation → Design Generation → Code Compilation → Deployable Website
Building the Prompt Processing Engine
The first step is understanding user intent from their natural language prompts. You'll want to:
# Example using OpenAI's API for prompt understanding
import openai
def analyze_prompt(user_prompt):
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[
{"role": "system", "content": "You are a website design assistant. Analyze this prompt and extract: business type, desired features, style preferences, and any specific content mentioned."},
{"role": "user", "content": user_prompt}
]
)
return response.choices[0].message.content
This helps break down vague requests like "I need a website for my bakery" into structured data your system can work with.
Generating Website Content
With the analyzed prompt, you can now generate appropriate content:
def generate_website_content(business_details):
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[
{"role": "system", "content": f"Generate website content for a {business_details['type']} business. Include homepage text, about page, and services/products."},
{"role": "user", "content": business_details}
]
)
return parse_content(response.choices[0].message.content)
Creating the Design
For design generation, you have several options:
- Template Selection: Use AI to pick from pre-made templates
- CSS Generation: Have AI write custom CSS
- Visual Generation: Use models like DALL·E for design mockups
// Example using AI to select a template
function selectTemplate(businessType, stylePreferences) {
// This would analyze your template library
// and return the best match based on AI analysis
}
Compiling the Website
Now combine everything into actual code. You could:
- Generate HTML/CSS directly
- Use a framework like React/Vue
- Output to a site builder format (Webflow, WordPress)
def compile_to_html(content, design_spec):
# This would take the generated content and design
# and assemble it into proper HTML/CSS
return f"""
{design_spec['css']}
{content['homepage']}
"""
Deployment Options
Finally, automate deployment to:
- Vercel/Netlify for static sites
- AWS/GCP for more complex apps
- Custom hosting solutions
// Example using Netlify's API
const deployToNetlify = async (siteFiles) => {
const response = await fetch('https://api.netlify.com/api/v1/sites', {
method: 'POST',
headers: {
'Authorization': `Bearer ${process.env.NETLIFY_TOKEN}`,
'Content-Type': 'application/zip'
},
body: siteFiles
});
return response.json();
};
Enhancing Your Generator
To make your platform stand out like Lovable.ai, consider adding:
- Multi-prompt refinement: Let users iteratively improve their site
- AI-powered editing: "Make it more modern" should regenerate accordingly
- Component library: Allow adding specific elements (contact forms, galleries)
- Personalization: Use AI to tailor content to target audience
Challenges to Consider
Building an AI website generator isn't without hurdles:
- Quality Control: AI can produce inconsistent results
- Specificity: Generic prompts lead to generic websites
- Technical Limitations: Complex functionality still requires manual work
- Cost: Running AI at scale can be expensive
Tech Stack Suggestions
- Frontend: Next.js, Vue, or Svelte
- AI Services: OpenAI API, Anthropic, or self-hosted LLMs
- Design: Tailwind CSS, AI-generated CSS, or template libraries
- Backend: Node.js, Python, or Go
- Hosting: Vercel, AWS, or specialized AI platforms
Getting Started
If you want to experiment with building your own version:
- Start with a simple single-page generator
- Use OpenAI's API for content creation
- Build a small library of templates
- Gradually add more complex features
The key is iterative development - start small and expand based on what works.