gRPC-Spring-Boot-Starter: Authentication Methods
Introduction The grpc-spring-boot-starter provides a seamless way to integrate gRPC services into Spring Boot applications. However, securing gRPC endpoints requires implementing authentication mechanisms. In this blog, we’ll explore different authentication strategies for gRPC with Spring Boot, including token-based authentication, TLS, and OAuth2. Why Secure gRPC Services? Since gRPC communicates over HTTP/2 and supports high-performance remote procedure calls, it is crucial to protect services from unauthorized access. Common threats include: Unauthorized access to sensitive data. Man-in-the-middle attacks. Service abuse due to unrestricted access. To prevent these issues, we can enforce authentication at the transport and application levels. Authentication Methods for gRPC in Spring Boot Here are some common authentication strategies for securing gRPC services: 1. Basic Authentication with Metadata A simple way to authenticate gRPC requests is by passing credentials in the metadata. Server-side Implementation @GrpcService public class AuthenticatedService extends AuthServiceGrpc.AuthServiceImplBase { @Override public void secureMethod(HelloRequest request, StreamObserver responseObserver) { // Extract metadata from the request Context ctx = Context.current(); String token = ctx.get(Metadata.Key.of("authorization", Metadata.ASCII_STRING_MARSHALLER)); if (!"valid-token".equals(token)) { responseObserver.onError(Status.UNAUTHENTICATED.withDescription("Invalid token").asRuntimeException()); return; } HelloResponse response = HelloResponse.newBuilder().setMessage("Hello, authenticated user!").build(); responseObserver.onNext(response); responseObserver.onCompleted(); } } Client-side Implementation ManagedChannel channel = ManagedChannelBuilder.forAddress("localhost", 9090).usePlaintext().build(); Metadata metadata = new Metadata(); metadata.put(Metadata.Key.of("authorization", Metadata.ASCII_STRING_MARSHALLER), "valid-token"); AuthServiceGrpc.AuthServiceBlockingStub stub = AuthServiceGrpc.newBlockingStub(channel) .withCallCredentials(new MetadataUtils(metadata)); HelloRequest request = HelloRequest.newBuilder().setName("John").build(); HelloResponse response = stub.secureMethod(request); System.out.println(response.getMessage()); 2. Secure gRPC with TLS Transport Layer Security (TLS) encrypts communication between the client and server, preventing data interception. Configuring TLS in Spring Boot gRPC Server In application.yml: grpc: server: security: enabled: true certificate-chain: classpath:certs/server-cert.pem private-key: classpath:certs/server-key.pem This ensures that all incoming gRPC requests must be encrypted. Configuring TLS in gRPC Client ManagedChannel channel = ManagedChannelBuilder .forAddress("localhost", 9090) .useTransportSecurity() .build(); With this setup, only TLS-secured connections are allowed. 3. OAuth2 Authentication with JWT For enterprise-grade security, gRPC can leverage OAuth2 and JWTs to authenticate requests. Server-side Configuration @GrpcService public class JwtAuthenticatedService extends AuthServiceGrpc.AuthServiceImplBase { @Override public void secureMethod(HelloRequest request, StreamObserver responseObserver) { try { String jwt = extractJwtFromMetadata(); DecodedJWT decodedJWT = JWT.require(Algorithm.HMAC256("secret-key")) .build() .verify(jwt); HelloResponse response = HelloResponse.newBuilder().setMessage("Welcome " + decodedJWT.getSubject()).build(); responseObserver.onNext(response); responseObserver.onCompleted(); } catch (JWTVerificationException e) { responseObserver.onError(Status.UNAUTHENTICATED.withDescription("Invalid JWT").asRuntimeException()); } } } Client-side Implementation String jwtToken = generateJwtToken(); Metadata metadata = new Metadata(); metadata.put(Metadata.Key.of("authorization", Metadata.ASCII_STRING_MARSHALLER), "Bearer " + jwtToken); With this setup, only valid JWTs are accepted for authentication. Conclusion gRPC services in Spring Boot can be secured using various authentication mechanisms, such as metadata-based authentication, TLS encryption, and OAuth2 with JWT. Depending on the security requirements, you can choose the method that best suits your needs. Would you like a deep dive into implementing role-based access control (RBAC) for gRPC? Let me know in the comments!
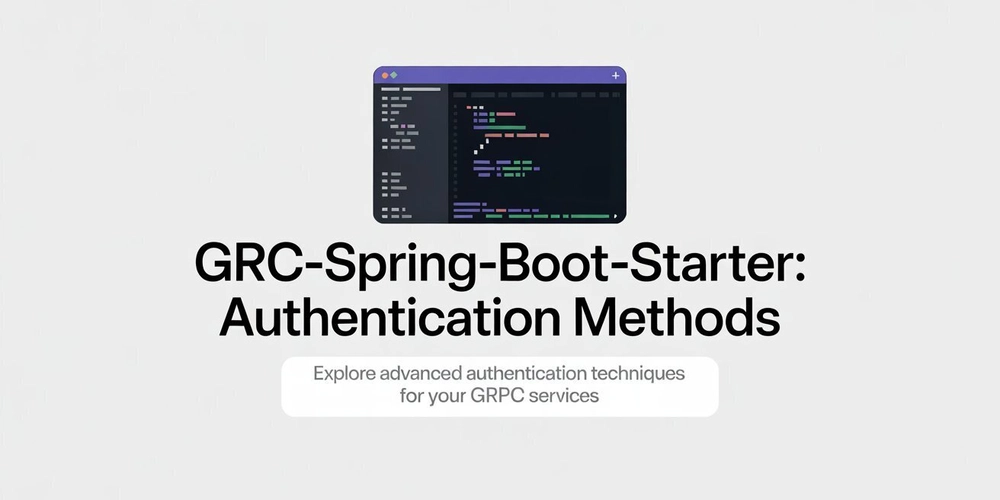
Introduction
The grpc-spring-boot-starter
provides a seamless way to integrate gRPC services into Spring Boot applications. However, securing gRPC endpoints requires implementing authentication mechanisms. In this blog, we’ll explore different authentication strategies for gRPC with Spring Boot, including token-based authentication, TLS, and OAuth2.
Why Secure gRPC Services?
Since gRPC communicates over HTTP/2 and supports high-performance remote procedure calls, it is crucial to protect services from unauthorized access. Common threats include:
- Unauthorized access to sensitive data.
- Man-in-the-middle attacks.
- Service abuse due to unrestricted access.
To prevent these issues, we can enforce authentication at the transport and application levels.
Authentication Methods for gRPC in Spring Boot
Here are some common authentication strategies for securing gRPC services:
1. Basic Authentication with Metadata
A simple way to authenticate gRPC requests is by passing credentials in the metadata.
Server-side Implementation
@GrpcService
public class AuthenticatedService extends AuthServiceGrpc.AuthServiceImplBase {
@Override
public void secureMethod(HelloRequest request, StreamObserver<HelloResponse> responseObserver) {
// Extract metadata from the request
Context ctx = Context.current();
String token = ctx.get(Metadata.Key.of("authorization", Metadata.ASCII_STRING_MARSHALLER));
if (!"valid-token".equals(token)) {
responseObserver.onError(Status.UNAUTHENTICATED.withDescription("Invalid token").asRuntimeException());
return;
}
HelloResponse response = HelloResponse.newBuilder().setMessage("Hello, authenticated user!").build();
responseObserver.onNext(response);
responseObserver.onCompleted();
}
}
Client-side Implementation
ManagedChannel channel = ManagedChannelBuilder.forAddress("localhost", 9090).usePlaintext().build();
Metadata metadata = new Metadata();
metadata.put(Metadata.Key.of("authorization", Metadata.ASCII_STRING_MARSHALLER), "valid-token");
AuthServiceGrpc.AuthServiceBlockingStub stub = AuthServiceGrpc.newBlockingStub(channel)
.withCallCredentials(new MetadataUtils(metadata));
HelloRequest request = HelloRequest.newBuilder().setName("John").build();
HelloResponse response = stub.secureMethod(request);
System.out.println(response.getMessage());
2. Secure gRPC with TLS
Transport Layer Security (TLS) encrypts communication between the client and server, preventing data interception.
Configuring TLS in Spring Boot gRPC Server
In application.yml
:
grpc:
server:
security:
enabled: true
certificate-chain: classpath:certs/server-cert.pem
private-key: classpath:certs/server-key.pem
This ensures that all incoming gRPC requests must be encrypted.
Configuring TLS in gRPC Client
ManagedChannel channel = ManagedChannelBuilder
.forAddress("localhost", 9090)
.useTransportSecurity()
.build();
With this setup, only TLS-secured connections are allowed.
3. OAuth2 Authentication with JWT
For enterprise-grade security, gRPC can leverage OAuth2 and JWTs to authenticate requests.
Server-side Configuration
@GrpcService
public class JwtAuthenticatedService extends AuthServiceGrpc.AuthServiceImplBase {
@Override
public void secureMethod(HelloRequest request, StreamObserver<HelloResponse> responseObserver) {
try {
String jwt = extractJwtFromMetadata();
DecodedJWT decodedJWT = JWT.require(Algorithm.HMAC256("secret-key"))
.build()
.verify(jwt);
HelloResponse response = HelloResponse.newBuilder().setMessage("Welcome " + decodedJWT.getSubject()).build();
responseObserver.onNext(response);
responseObserver.onCompleted();
} catch (JWTVerificationException e) {
responseObserver.onError(Status.UNAUTHENTICATED.withDescription("Invalid JWT").asRuntimeException());
}
}
}
Client-side Implementation
String jwtToken = generateJwtToken();
Metadata metadata = new Metadata();
metadata.put(Metadata.Key.of("authorization", Metadata.ASCII_STRING_MARSHALLER), "Bearer " + jwtToken);
With this setup, only valid JWTs are accepted for authentication.
Conclusion
gRPC services in Spring Boot can be secured using various authentication mechanisms, such as metadata-based authentication, TLS encryption, and OAuth2 with JWT. Depending on the security requirements, you can choose the method that best suits your needs.
Would you like a deep dive into implementing role-based access control (RBAC) for gRPC? Let me know in the comments!