Exploring Next.js 15 and Server Actions: Features and Best Practices
Exploring Next.js 15 and Server Actions: Features and Best Practices Introduction Next.js 15 has introduced a plethora of new features that enhance the developer experience and improve application performance. One of the standout features is Server Actions, which allows developers to handle server-side logic more efficiently. In this blog post, we will dive into the new features of Next.js 15, particularly focusing on Server Actions, and provide practical implementation examples and best practices. What are Server Actions? Server Actions are a new way to handle server-side logic directly within your components. This feature simplifies data fetching and mutation processes, allowing developers to write cleaner and more maintainable code. With Server Actions, you can define functions that run on the server and can be called directly from your client-side components. Key Features of Next.js 15 Improved Performance: Next.js 15 optimizes rendering and data fetching, leading to faster load times. Server Actions: Simplifies server-side logic and enhances the developer experience. Enhanced Routing: New routing capabilities allow for more dynamic and flexible applications. Automatic React Server Components: Streamlines the use of React Server Components, improving performance and usability. Implementing Server Actions To illustrate how to implement Server Actions, let’s create a simple example where we fetch user data from an API and display it in a component. Step 1: Setting Up Your Next.js Project First, ensure you have Next.js 15 installed. You can create a new project using the following command: npx create-next-app@latest my-next-app cd my-next-app Step 2: Creating a Server Action Next, let’s create a server action to fetch user data. Create a new file named actions.js in the app directory: // app/actions.js export async function fetchUserData(userId) { const response = await fetch(`https://jsonplaceholder.typicode.com/users/${userId}`); if (!response.ok) { throw new Error('Failed to fetch user data'); } return response.json(); } Step 3: Using the Server Action in a Component Now, let’s use this server action in a component. Create a new file named UserProfile.js in the app directory: // app/UserProfile.js import { fetchUserData } from './actions'; export default async function UserProfile({ userId }) { const user = await fetchUserData(userId); return ( {user.name} {user.email} ); } Step 4: Rendering the Component Finally, render the UserProfile component in your main page. Update page.js as follows: // app/page.js import UserProfile from './UserProfile'; export default function Home() { return ( User Profile ); } Best Practices for Using Server Actions Keep Actions Focused: Each server action should handle a single responsibility to maintain clarity and reusability. Error Handling: Implement robust error handling within your server actions to manage API failures gracefully. Optimize Data Fetching: Use caching strategies where applicable to reduce unnecessary API calls and improve performance. Security Considerations: Be mindful of sensitive data and ensure that server actions are secure and validate inputs properly. Real-World Use Cases User Authentication: Server Actions can be used to handle user login and registration processes securely. Data Management: Applications that require CRUD operations can leverage Server Actions for efficient data handling. Dynamic Content Loading: Use Server Actions to fetch and display dynamic content based on user interactions or preferences. Conclusion Next.js 15 and its Server Actions feature provide developers with powerful tools to build efficient and maintainable applications. By simplifying server-side logic and enhancing performance, Next.js 15 is a game-changer for modern web development. Embrace these new features to elevate your projects and improve your development workflow.
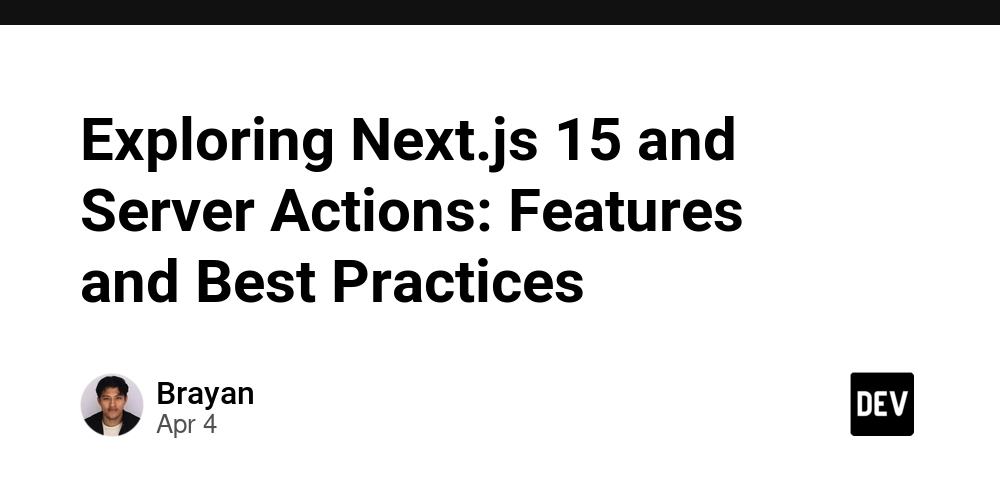
Exploring Next.js 15 and Server Actions: Features and Best Practices
Introduction
Next.js 15 has introduced a plethora of new features that enhance the developer experience and improve application performance. One of the standout features is Server Actions, which allows developers to handle server-side logic more efficiently. In this blog post, we will dive into the new features of Next.js 15, particularly focusing on Server Actions, and provide practical implementation examples and best practices.
What are Server Actions?
Server Actions are a new way to handle server-side logic directly within your components. This feature simplifies data fetching and mutation processes, allowing developers to write cleaner and more maintainable code. With Server Actions, you can define functions that run on the server and can be called directly from your client-side components.
Key Features of Next.js 15
- Improved Performance: Next.js 15 optimizes rendering and data fetching, leading to faster load times.
- Server Actions: Simplifies server-side logic and enhances the developer experience.
- Enhanced Routing: New routing capabilities allow for more dynamic and flexible applications.
- Automatic React Server Components: Streamlines the use of React Server Components, improving performance and usability.
Implementing Server Actions
To illustrate how to implement Server Actions, let’s create a simple example where we fetch user data from an API and display it in a component.
Step 1: Setting Up Your Next.js Project
First, ensure you have Next.js 15 installed. You can create a new project using the following command:
npx create-next-app@latest my-next-app
cd my-next-app
Step 2: Creating a Server Action
Next, let’s create a server action to fetch user data. Create a new file named actions.js
in the app
directory:
// app/actions.js
export async function fetchUserData(userId) {
const response = await fetch(`https://jsonplaceholder.typicode.com/users/${userId}`);
if (!response.ok) {
throw new Error('Failed to fetch user data');
}
return response.json();
}
Step 3: Using the Server Action in a Component
Now, let’s use this server action in a component. Create a new file named UserProfile.js
in the app
directory:
// app/UserProfile.js
import { fetchUserData } from './actions';
export default async function UserProfile({ userId }) {
const user = await fetchUserData(userId);
return (
<div>
<h1>{user.name}</h1>
<p>{user.email}</p>
</div>
);
}
Step 4: Rendering the Component
Finally, render the UserProfile
component in your main page. Update page.js
as follows:
// app/page.js
import UserProfile from './UserProfile';
export default function Home() {
return (
<div>
<h1>User Profile</h1>
<UserProfile userId={1} />
</div>
);
}
Best Practices for Using Server Actions
- Keep Actions Focused: Each server action should handle a single responsibility to maintain clarity and reusability.
- Error Handling: Implement robust error handling within your server actions to manage API failures gracefully.
- Optimize Data Fetching: Use caching strategies where applicable to reduce unnecessary API calls and improve performance.
- Security Considerations: Be mindful of sensitive data and ensure that server actions are secure and validate inputs properly.
Real-World Use Cases
- User Authentication: Server Actions can be used to handle user login and registration processes securely.
- Data Management: Applications that require CRUD operations can leverage Server Actions for efficient data handling.
- Dynamic Content Loading: Use Server Actions to fetch and display dynamic content based on user interactions or preferences.
Conclusion
Next.js 15 and its Server Actions feature provide developers with powerful tools to build efficient and maintainable applications. By simplifying server-side logic and enhancing performance, Next.js 15 is a game-changer for modern web development. Embrace these new features to elevate your projects and improve your development workflow.