Domain Driven Design(DDD) - Understanding Main Concepts
Domain Driven Design(DDD) is a form of software design that focuses on understanding and reflecting the business in code. Clean Architectures in general work really well with DDD. This happens because of layered separation, where the domain is at the center, meaning the domain layer does not depend on anything external. Main Concepts 1. Domain Is the business problem we are solving. A good domain model captures the essential business rules and behaviours while staying independent of infrastructure concerns. That's why it's important to use the same language as the domain/business team, ensuring that developers (technical) and domain experts (non technical) stay aligned. This is what its called, ubiquitous language. 2. Entities and Value Objects Entities are defined by their unique identity and not by their attributes. Even if their properties change, they are still the same entity. For example, a Customer is an entity because it has a unique identity (customer ID or email address) that distinguishes it from other customers. Even if the customer’s name, or other details change, the customer remains the same as long as the identity (ID or email) stays the same. Value objects(VO), on the other hand, are defined by their attributes and have no unique identity. Two value objects that have the same attributes are considered identical. For example, Address can be a VO, two identical addresses (same street, same city, same postal code) are interchangeable and don't hold any unique identity beyond their attributes. In an organization, the structure can be composed of multiple entities, each with its corresponding value objects. 3. Aggregates and Aggregate Roots An aggregate is a group of related domain objects that should be treated as a single unit (have a look at the image below for a better understanding). This ensures consistency and encapsulate business rules. The aggregate root is the primary entity that controls access to other objects within the aggregate. The aggregate root is always an entity and never a value object. Aggregates communicate primarily through domain events. When something happens within one aggregate that needs to affect another aggregate, the first aggregate sends an event, and other aggregates are listening and react to that event. A more real life example of aggregates. In a library system, a library can manage multiple books, each with its own attributes such as title, author, and location within the library. Library (Aggregate Root) The Library is an entity because it has a unique identity (libraryId) and it represents the collection of books within the system. It is also the aggregate root, meaning it controls access to the other entities within the aggregate (the Book entities in this case). Book (Entity) The Book is an entity because it also has a unique identity (the isbn) and it represents a specific book in the library collection. It is part of the Library aggregate, and its properties are managed through the Library. BookDetails and Location (VO) They are value objects because they don’t have an identity and are defined only by their attributes. 4. Domain Services Sometimes, business logic does not naturally belong to an entity or value object. In such cases, we use domain services to encapsulate that logic. 5. Domain Events Domain events capture important occurrences within the business domain. For example, when a payment is completed, an event like PaymentCompleted can be published, triggering related actions such as sending an email notification. 6. Bounded Contexts This concept is a bit abstract. Bounded contexts, represents subdomains with its own models and logic. It's like a "boundary" that separates one part of a system from others. For example, in an online store, you might have one bounded context for order management and another for payment processing, each with its own models and logic. Don't mix aggregates and bounded contexts, aggregates are smaller groups and exist inside a bounded context, but bounded contexts help separate different business areas within the whole system Conclusion DDD is not just about structuring code, it’s a mindset shift that emphasizes deep collaboration with domain experts and models business logic in a way that is both clear and maintainable.
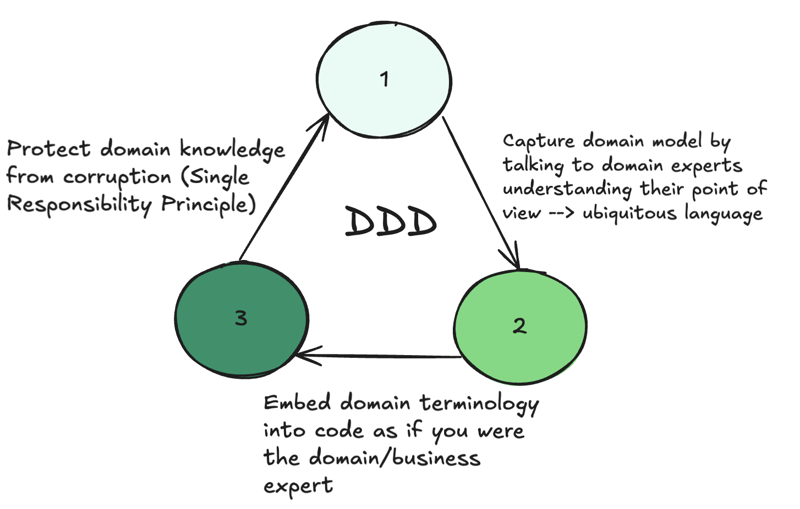
Domain Driven Design(DDD) is a form of software design that focuses on understanding and reflecting the business in code. Clean Architectures in general work really well with DDD. This happens because of layered separation, where the domain is at the center, meaning the domain layer does not depend on anything external.
Main Concepts
1. Domain
Is the business problem we are solving. A good domain model captures the essential business rules and behaviours while staying independent of infrastructure concerns. That's why it's important to use the same language as the domain/business team, ensuring that developers (technical) and domain experts (non technical) stay aligned. This is what its called, ubiquitous language.
2. Entities and Value Objects
Entities are defined by their unique identity and not by their attributes. Even if their properties change, they are still the same entity.
For example, aCustomer
is an entity because it has a unique identity (customer ID or email address) that distinguishes it from other customers. Even if the customer’s name, or other details change, the customer remains the same as long as the identity (ID or email) stays the same.Value objects(VO), on the other hand, are defined by their attributes and have no unique identity. Two value objects that have the same attributes are considered identical.
For example,Address
can be a VO, two identical addresses (same street, same city, same postal code) are interchangeable and don't hold any unique identity beyond their attributes.
In an organization, the structure can be composed of multiple entities, each with its corresponding value objects.
3. Aggregates and Aggregate Roots
- An aggregate is a group of related domain objects that should be treated as a single unit (have a look at the image below for a better understanding). This ensures consistency and encapsulate business rules.
- The aggregate root is the primary entity that controls access to other objects within the aggregate. The aggregate root is always an entity and never a value object.
Aggregates communicate primarily through domain events. When something happens within one aggregate that needs to affect another aggregate, the first aggregate sends an event, and other aggregates are listening and react to that event.
A more real life example of aggregates. In a library system, a library can manage multiple books, each with its own attributes such as title, author, and location within the library.
- Library (Aggregate Root)
The Library is an entity because it has a unique identity (libraryId) and it represents the collection of books within the system.
It is also the aggregate root, meaning it controls access to the other entities within the aggregate (the Book entities in this case).
- Book (Entity)
The Book is an entity because it also has a unique identity (the isbn) and it represents a specific book in the library collection.
It is part of the Library aggregate, and its properties are managed through the Library.
- BookDetails and Location (VO)
They are value objects because they don’t have an identity and are defined only by their attributes.
4. Domain Services
Sometimes, business logic does not naturally belong to an entity or value object. In such cases, we use domain services to encapsulate that logic.
5. Domain Events
Domain events capture important occurrences within the business domain. For example, when a payment is completed, an event like PaymentCompleted can be published, triggering related actions such as sending an email notification.
6. Bounded Contexts
This concept is a bit abstract. Bounded contexts, represents subdomains with its own models and logic. It's like a "boundary" that separates one part of a system from others. For example, in an online store, you might have one bounded context for order management and another for payment processing, each with its own models and logic.
Don't mix aggregates and bounded contexts, aggregates are smaller groups and exist inside a bounded context, but bounded contexts help separate different business areas within the whole system
Conclusion
DDD is not just about structuring code, it’s a mindset shift that emphasizes deep collaboration with domain experts and models business logic in a way that is both clear and maintainable.