Complete Guide to Building Apps with Jamstack: Speed and Scalability Guaranteed
The Jamstack architecture (JavaScript, APIs, and Markup) has become one of the most popular solutions for developing modern web applications. This guide will provide you with a deep understanding of what Jamstack is, why it is relevant in today's landscape, and how you can implement it in your projects. What is Jamstack? Jamstack is an approach to building sites and applications that provide a fast and secure user experience. The basic premise is to separate the markup layer from application logic (JavaScript) and API access. This allows for greater flexibility and resource optimization, resulting in superior performance. Advantages of Using Jamstack Performance: Jamstack applications are fast because static files are served, which minimizes server workloads. Security: By eliminating the need for complex backend functionality, the number of attack points is reduced. Scalability: By using a CDN to serve static content, applications can easily scale to handle high traffic. Simplified development: Separating front-end and back-end makes it easier for development teams to collaborate. Key Qualities of Jamstack Jamstack is built on three fundamental pillars: JavaScript: Programming language that provides interactivity and functionality through the use of frameworks such as React, Vue or Angular. APIs: APIs are used for server functionality and data management, and can be third-party or custom. Markup: Markdown and static site generators (SSG) like Gatsby or Next.js are typically used for content creation. Building Your First Jamstack App Next, I'll show you how you can set up a basic application using Next.js as a static site generator and Contentful as a CMS. Set up a Project in Next.js npx create-next-app nombre-del-proyecto Install the Contentful SDK npm install contentful Set up Contentful Head over to Contentful and create an account. Set up a new space and define the content templates you need. Code to Get Data from Contentful import { createClient } from 'contentful'; const client = createClient({ space: process.env.CONTENTFUL_SPACE_ID, accessToken: process.env.CONTENTFUL_ACCESS_TOKEN }); export async function getStaticProps() { const res = await client.getEntries(); return { props: { entries: res.items, }, }; } Render the Content export default function Home({ entries }) { return ( Mis entradas {entries.map(entry => ( {entry.fields.title} {entry.fields.description} ))} ); } Conclusions Jamstack offers a robust methodology for developing web applications that prioritizes speed, security, and scalability. If you haven't tried this architecture yet, consider implementing it in your next project. Start exploring the potential of Jamstack in your web applications today!
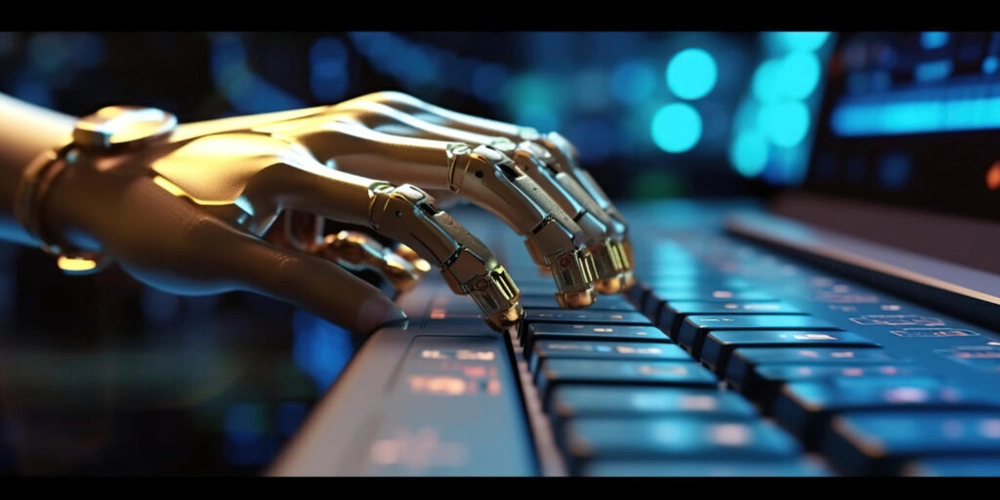
The Jamstack architecture (JavaScript, APIs, and Markup) has become one of the most popular solutions for developing modern web applications. This guide will provide you with a deep understanding of what Jamstack is, why it is relevant in today's landscape, and how you can implement it in your projects.
What is Jamstack?
Jamstack is an approach to building sites and applications that provide a fast and secure user experience. The basic premise is to separate the markup layer from application logic (JavaScript) and API access. This allows for greater flexibility and resource optimization, resulting in superior performance.
Advantages of Using Jamstack
Performance: Jamstack applications are fast because static files are served, which minimizes server workloads.
Security: By eliminating the need for complex backend functionality, the number of attack points is reduced.
Scalability: By using a CDN to serve static content, applications can easily scale to handle high traffic.
Simplified development: Separating front-end and back-end makes it easier for development teams to collaborate.
Key Qualities of Jamstack
Jamstack is built on three fundamental pillars:
JavaScript: Programming language that provides interactivity and functionality through the use of frameworks such as React, Vue or Angular.
APIs: APIs are used for server functionality and data management, and can be third-party or custom.
Markup: Markdown and static site generators (SSG) like Gatsby or Next.js are typically used for content creation.
Building Your First Jamstack App
Next, I'll show you how you can set up a basic application using Next.js as a static site generator and Contentful as a CMS.
- Set up a Project in Next.js npx create-next-app nombre-del-proyecto
- Install the Contentful SDK npm install contentful
Set up Contentful
Head over to Contentful and create an account. Set up a new space and define the content templates you need.Code to Get Data from Contentful
import { createClient } from 'contentful';
const client = createClient({
space: process.env.CONTENTFUL_SPACE_ID,
accessToken: process.env.CONTENTFUL_ACCESS_TOKEN
});
export async function getStaticProps() {
const res = await client.getEntries();
return {
props: {
entries: res.items,
},
};
}
- Render the Content export default function Home({ entries }) { return ( Mis entradas {entries.map(entry => ( {entry.fields.title} {entry.fields.description} ))} ); } Conclusions Jamstack offers a robust methodology for developing web applications that prioritizes speed, security, and scalability. If you haven't tried this architecture yet, consider implementing it in your next project.
Start exploring the potential of Jamstack in your web applications today!