Comparing AI SDKs for React: Vercel, LangChain, Hugging Face
Comparing AI SDKs for React: Vercel, LangChain, Hugging Face Introduction In the rapidly evolving landscape of artificial intelligence, developers are increasingly integrating AI capabilities into their applications. For React developers, several AI SDKs are available, each offering unique features and functionalities. In this post, we will compare some of the most popular AI SDKs for React applications, including Vercel AI SDK, LangChain, and Hugging Face. We will explore their features, limitations, and practical use cases, along with code examples to illustrate their implementation. Vercel AI SDK Features Seamless Integration: Vercel AI SDK is designed to work effortlessly with Vercel's deployment platform. Serverless Functions: It allows developers to create serverless functions that can handle AI requests efficiently. Real-time Capabilities: Supports real-time data processing, making it suitable for chatbots and interactive applications. Limitations Vendor Lock-in: Tightly coupled with Vercel's ecosystem, which may not be ideal for all projects. Limited Documentation: Some users report that the documentation could be more comprehensive. Code Example import { createAI } from 'vercel-ai-sdk'; const ai = createAI({ apiKey: 'YOUR_API_KEY', }); const response = await ai.query('What is the capital of France?'); console.log(response); LangChain Features Chainable Components: LangChain allows developers to create complex AI workflows by chaining components together. Multi-Model Support: It supports various AI models, enabling flexibility in choosing the right model for the task. Extensive Community: A growing community that contributes to its development and provides support. Limitations Steeper Learning Curve: The flexibility comes with complexity, which may be overwhelming for beginners. Performance Overhead: Chaining multiple components can introduce latency in response times. Code Example import { LangChain } from 'langchain'; const chain = new LangChain(); chain.addModel('gpt-3.5-turbo'); const result = await chain.run('Tell me a joke.'); console.log(result); Hugging Face Features Pre-trained Models: Offers a wide range of pre-trained models for various NLP tasks, including text generation and sentiment analysis. Transformers Library: The Transformers library provides easy access to state-of-the-art models. Community and Resources: A large community and extensive resources for learning and troubleshooting. Limitations Resource Intensive: Running large models can be resource-intensive, requiring significant computational power. Complex Setup: Initial setup and configuration can be complex for new users. Code Example import { HuggingFace } from 'huggingface-sdk'; const hf = new HuggingFace({ model: 'gpt-2', }); const output = await hf.generate('Once upon a time...'); console.log(output); Conclusion Choosing the right AI SDK for your React application depends on your specific needs and project requirements. Vercel AI SDK is excellent for those already using Vercel's platform, while LangChain offers flexibility for complex workflows. Hugging Face is ideal for developers looking for powerful pre-trained models. By understanding the features, limitations, and use cases of each SDK, you can make an informed decision that best suits your development goals. Tags react ai sdk vercel huggingface Description Explore a detailed comparison of popular AI SDKs for React applications, including Vercel AI SDK, LangChain, and Hugging Face, with code examples and use cases.
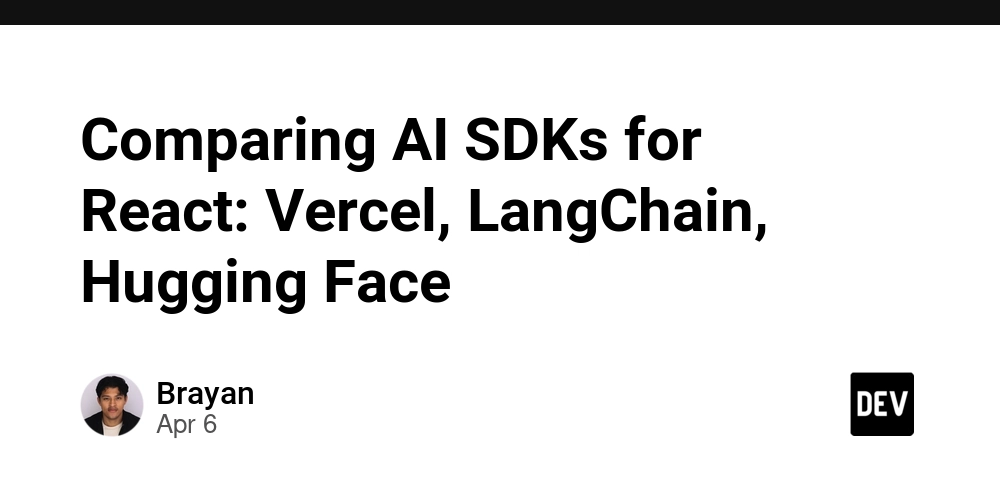
Comparing AI SDKs for React: Vercel, LangChain, Hugging Face
Introduction
In the rapidly evolving landscape of artificial intelligence, developers are increasingly integrating AI capabilities into their applications. For React developers, several AI SDKs are available, each offering unique features and functionalities. In this post, we will compare some of the most popular AI SDKs for React applications, including Vercel AI SDK, LangChain, and Hugging Face. We will explore their features, limitations, and practical use cases, along with code examples to illustrate their implementation.
Vercel AI SDK
Features
- Seamless Integration: Vercel AI SDK is designed to work effortlessly with Vercel's deployment platform.
- Serverless Functions: It allows developers to create serverless functions that can handle AI requests efficiently.
- Real-time Capabilities: Supports real-time data processing, making it suitable for chatbots and interactive applications.
Limitations
- Vendor Lock-in: Tightly coupled with Vercel's ecosystem, which may not be ideal for all projects.
- Limited Documentation: Some users report that the documentation could be more comprehensive.
Code Example
import { createAI } from 'vercel-ai-sdk';
const ai = createAI({
apiKey: 'YOUR_API_KEY',
});
const response = await ai.query('What is the capital of France?');
console.log(response);
LangChain
Features
- Chainable Components: LangChain allows developers to create complex AI workflows by chaining components together.
- Multi-Model Support: It supports various AI models, enabling flexibility in choosing the right model for the task.
- Extensive Community: A growing community that contributes to its development and provides support.
Limitations
- Steeper Learning Curve: The flexibility comes with complexity, which may be overwhelming for beginners.
- Performance Overhead: Chaining multiple components can introduce latency in response times.
Code Example
import { LangChain } from 'langchain';
const chain = new LangChain();
chain.addModel('gpt-3.5-turbo');
const result = await chain.run('Tell me a joke.');
console.log(result);
Hugging Face
Features
- Pre-trained Models: Offers a wide range of pre-trained models for various NLP tasks, including text generation and sentiment analysis.
- Transformers Library: The Transformers library provides easy access to state-of-the-art models.
- Community and Resources: A large community and extensive resources for learning and troubleshooting.
Limitations
- Resource Intensive: Running large models can be resource-intensive, requiring significant computational power.
- Complex Setup: Initial setup and configuration can be complex for new users.
Code Example
import { HuggingFace } from 'huggingface-sdk';
const hf = new HuggingFace({
model: 'gpt-2',
});
const output = await hf.generate('Once upon a time...');
console.log(output);
Conclusion
Choosing the right AI SDK for your React application depends on your specific needs and project requirements. Vercel AI SDK is excellent for those already using Vercel's platform, while LangChain offers flexibility for complex workflows. Hugging Face is ideal for developers looking for powerful pre-trained models. By understanding the features, limitations, and use cases of each SDK, you can make an informed decision that best suits your development goals.
Tags
- react
- ai
- sdk
- vercel
- huggingface
Description
Explore a detailed comparison of popular AI SDKs for React applications, including Vercel AI SDK, LangChain, and Hugging Face, with code examples and use cases.