C# vs Angular: Universal Principles of Dependency Injection
Introduction Dependency Injection (DI) is a concept so deeply ingrained in everyday programming practices that ignoring it could almost be considered a cardinal sin, on par with neglecting version control. But why has DI become so crucial? DI is one of the key principles enabling the creation of flexible and maintainable applications. The philosophy behind it revolves around freeing code from unnecessary details that tightly couple logical components. Components no longer depend on specific implementations of other parts of the system—they simply declare their needs, and DI provides the required dependencies. The goal here is not just to master a trendy technology but to explore a universal architectural tool whose concepts transcend different ecosystems. Studying DI across multiple languages and environments not only deepens your understanding of the concept itself but also broadens your perspective on system design. You’ll come to realize that, despite syntactic differences, fundamental ideas converge toward the same architectural goals. Who will benefit from this article? If you’re already familiar with .NET’s IServiceCollection but have always wanted to understand Angular’s Injectors, you’re in the right place. Conversely, if you write TypeScript code but the term "Transient" leaves you puzzled—welcome aboard. We’ll explore how similar concepts adapt to two different worlds and why studying both ecosystems will help you design better applications. The aim of this piece is to demonstrate that the fundamental principles of DI remain consistent regardless of framework popularity or language-specific syntax. In short, you’ll see how elegant ideas underpin technologies as diverse as C# and Angular. What Is DI? A Unified Philosophy Dependency Injection may seem complex at first glance, but its "magic" boils down to a simple idea: let a specialized mechanism handle the creation and management of dependencies in your application. This relieves the code of unnecessary coupling, making the application significantly more flexible and testable. Why Reduce Coupling? A primary goal of DI is decoupling. Application components no longer rigidly depend on one another. Instead, they work with abstractions, and concrete implementations are dynamically supplied. Remember the golden rule of good architecture: depend on interfaces, not concrete classes. Reduced coupling means components are easier to test (dependencies can be replaced with mocks). It also simplifies extending functionality: you can swap one implementation for another without rewriting old code—just register the new dependency. This is especially important in large applications where changes require careful validation and minimal side effects. Similarities in C# and Angular Here’s the interesting part. At first glance, C# and Angular seem like entirely different technologies: strict, OOP-oriented C# versus Angular’s modular, declarative approach. Yet both systems understand and support DI in the same way, proving the universality of this philosophy. At the core of DI in Angular and C# lies the same key idea: inversion of control. The architectural magic in both revolves around a "dependency container"—a mechanism that handles object creation, configuration, and management. Angular uses a powerful system of "injectors," while C# relies on IoC containers (Inversion of Control). Additionally, both approaches clearly recognize dependency lifecycles, offering flexibility: from objects created anew each time to Singleton implementations that persist for the application’s entire lifespan. DI in C#: Runtime Injection Flexibility In the .NET ecosystem, DI centers on the IServiceCollection interface for registering dependencies and the DependencyResolver mechanism for retrieving them. Registered dependencies become automatically available, eliminating the need to manually specify how each object is created. Injection can occur via constructors, methods, or properties—giving you full control over how and where dependencies integrate. Why is this useful? Constructors suit mandatory dependencies, methods work for optional ones, and properties enable dependencies relevant only at specific times. All you need to do is configure the DI container correctly. IoC Containers and Service Lifetime A major strength of DI in .NET is lifecycle management (Service Lifetime). Using Transient, Scoped, and Singleton, you define how long an object exists and how often it’s created. Transient: A new object is created each time it’s requested. Ideal for lightweight, "disposable" dependencies. Scoped: An object is created once per request or application scope. Useful for database contexts or related data within a single request. Singleton: An object is created once and persists for the application’s lifetime. Configuring DI in ASP.NET Core The beauty of DI in .NET lies in its simplicity. The Confi
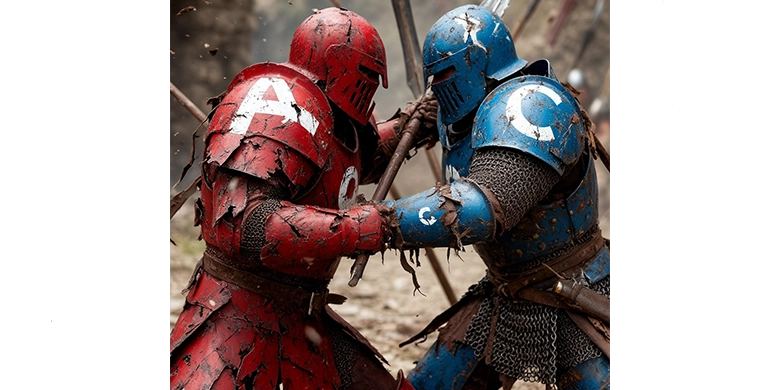
Introduction
Dependency Injection (DI) is a concept so deeply ingrained in everyday programming practices that ignoring it could almost be considered a cardinal sin, on par with neglecting version control. But why has DI become so crucial?
DI is one of the key principles enabling the creation of flexible and maintainable applications. The philosophy behind it revolves around freeing code from unnecessary details that tightly couple logical components. Components no longer depend on specific implementations of other parts of the system—they simply declare their needs, and DI provides the required dependencies.
The goal here is not just to master a trendy technology but to explore a universal architectural tool whose concepts transcend different ecosystems. Studying DI across multiple languages and environments not only deepens your understanding of the concept itself but also broadens your perspective on system design. You’ll come to realize that, despite syntactic differences, fundamental ideas converge toward the same architectural goals.
Who will benefit from this article? If you’re already familiar with .NET’s IServiceCollection
but have always wanted to understand Angular’s Injectors, you’re in the right place. Conversely, if you write TypeScript code but the term "Transient" leaves you puzzled—welcome aboard. We’ll explore how similar concepts adapt to two different worlds and why studying both ecosystems will help you design better applications.
The aim of this piece is to demonstrate that the fundamental principles of DI remain consistent regardless of framework popularity or language-specific syntax. In short, you’ll see how elegant ideas underpin technologies as diverse as C# and Angular.
What Is DI? A Unified Philosophy
Dependency Injection may seem complex at first glance, but its "magic" boils down to a simple idea: let a specialized mechanism handle the creation and management of dependencies in your application. This relieves the code of unnecessary coupling, making the application significantly more flexible and testable.
Why Reduce Coupling?
A primary goal of DI is decoupling. Application components no longer rigidly depend on one another. Instead, they work with abstractions, and concrete implementations are dynamically supplied. Remember the golden rule of good architecture: depend on interfaces, not concrete classes.
Reduced coupling means components are easier to test (dependencies can be replaced with mocks). It also simplifies extending functionality: you can swap one implementation for another without rewriting old code—just register the new dependency. This is especially important in large applications where changes require careful validation and minimal side effects.
Similarities in C# and Angular
Here’s the interesting part. At first glance, C# and Angular seem like entirely different technologies: strict, OOP-oriented C# versus Angular’s modular, declarative approach. Yet both systems understand and support DI in the same way, proving the universality of this philosophy.
At the core of DI in Angular and C# lies the same key idea: inversion of control. The architectural magic in both revolves around a "dependency container"—a mechanism that handles object creation, configuration, and management.
Angular uses a powerful system of "injectors," while C# relies on IoC containers (Inversion of Control).
Additionally, both approaches clearly recognize dependency lifecycles, offering flexibility: from objects created anew each time to Singleton implementations that persist for the application’s entire lifespan.
DI in C#: Runtime Injection Flexibility
In the .NET ecosystem, DI centers on the IServiceCollection
interface for registering dependencies and the DependencyResolver
mechanism for retrieving them. Registered dependencies become automatically available, eliminating the need to manually specify how each object is created. Injection can occur via constructors, methods, or properties—giving you full control over how and where dependencies integrate.
Why is this useful? Constructors suit mandatory dependencies, methods work for optional ones, and properties enable dependencies relevant only at specific times. All you need to do is configure the DI container correctly.
IoC Containers and Service Lifetime
A major strength of DI in .NET is lifecycle management (Service Lifetime). Using Transient
, Scoped
, and Singleton
, you define how long an object exists and how often it’s created.
- Transient: A new object is created each time it’s requested. Ideal for lightweight, "disposable" dependencies.
- Scoped: An object is created once per request or application scope. Useful for database contexts or related data within a single request.
- Singleton: An object is created once and persists for the application’s lifetime.
Configuring DI in ASP.NET Core
The beauty of DI in .NET lies in its simplicity. The ConfigureServices
method registers dependencies in the container, making them available throughout your application.
public void ConfigureServices(IServiceCollection services) {
// Register dependencies with different lifetimes
services.AddTransient<IMyTransientService, MyTransientService>();
services.AddScoped<IMyScopedService, MyScopedService>();
services.AddSingleton<IMySingletonService, MySingletonService>();
}
Demonstration of Usage
As a mini-example, let's demonstrate the use of some already registered dependency in a controller.
public class MyController {
private readonly IMyService _myService;
public MyController(IMyService myService)
{
_myService = myService;
}
public string GetMessage()
{
return _myService.GetGreeting();
}
}
The controller here focuses only on its task, while the creation and management of IMyService
remains the responsibility of the DI container.
DI in Angular: Declarative Approach with Metadata Binding
Dependency Injection (DI) in Angular is a fundamental part of its architecture, making applications modular, flexible, and easily extensible. If you work with Angular, DI will be your constant companion—it ensures that components and services interact simply, clearly, and without surprises.
Basics of DI Mechanism in Angular
Angular uses a powerful and declarative DI system based on three main pillars: Injectors
, Providers
, and Injection Tokens
.
- Injectors — the foundation of the entire system, a repository that manages the creation and provision of dependencies. It handles all the "heavy lifting," leaving you only with configuration.
- Providers — a way to instruct Angular on how to create or provide a dependency. Here, you declare: "for this interface or token, use this class or value."
- Injection Tokens — a solution for cases where no existing interface or class is available. They allow the use of arbitrary identifiers for dependencies.
Providers: Root, Module, and Component-Level Scopes
The flexibility of DI in Angular is achieved through different provider visibility levels. Depending on the scope, you can specify:
- root — the provider becomes available application-wide. Ideal for global services used everywhere.
- module — the provider is limited to a specific module. Useful for services required within a particular feature or application section.
- component-level — creates a unique dependency instance for each component. This enables data and state isolation between components.
For example, you can register a provider globally via @Injectable({ providedIn: 'root' })
or bind it to a component using the providers
array in @Component
.
Using Decorators and Metadata
Angular ensures dependency configuration remains declarative and intuitive. Decorators like @Injectable
or @Inject
explicitly "mark" what Angular needs to operate.
The @Injectable
decorator indicates that a class can be used as a dependency and specifies its registration scope. For greater flexibility, @Inject
allows explicit token or provider specification at injection points.
Dependency injection in a class is straightforward:
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
providers: [ExampleService]
})
export class ExampleComponent {
constructor(private exampleService: ExampleService) {
console.log(this.exampleService.getData());
}
}
IoC Containers, C# in Angular
The DI mechanism took care of initialization and passed the ready-made dependency to the component.
Unified Principles
When it comes to Dependency Injection, it's surprising how similar the approaches are in seemingly different technology stacks like Angular and C#. Despite the differences in ecosystems and languages, both tools use unified architectural principles for building applications. Let's examine the key points.
IoC (Inversion of Control)
The core idea of DI is the inversion of control (IoC). The traditional approach assumes that a class itself creates instances of its dependencies. DI changes the rules: control is now delegated to the container.
-
In Angular, this task is handled by the
Injector
. It determines what, when, and where to create, ensuring components or services receive only ready-made dependencies. The code remains simple and isolated from infrastructure details. -
In C#, the IoC container plays a similar role. Through it, you declare dependencies (e.g., using
IServiceCollection
), and the system handles their creation.
This transfer of control simplifies both code writing and architectural changes: classes become independent of implementation details.
Scopes (Lifespan)
Both Angular and C# DI provide flexible mechanisms for defining object "lifespans," helping manage state and limit dependency scopes.
-
Angular offers three main scopes:
root
(global),module
/component
, andfactory
(local). The system automatically creates new instances or reuses existing ones based on the specified level. -
C# uses
Transient
,Scoped
, andSingleton
lifetimes. These determine whether a new object is created each time, preserved within a request, or maintained as a single application-wide instance.
Angular Scope | C# Lifetime |
---|---|
root |
Singleton |
component /module
|
Scoped |
factory |
Transient |
Motivation and Testability
Both approaches agree on another point: Dependency Injection is key to testability. Simplifying dependency substitution allows testing classes or components in isolation.
- Angular and npm libraries provide tools for mocking objects, replacing real dependencies with mocks to speed up testing and ensure determinism.
- C# leverages mock objects and interfaces replaceable via the DI container in unit tests. Test implementations or libraries simulate any behavior.
Thus, DI in both technologies minimizes component coupling, enhances modularity, and accelerates the creation of quality tests.
Key Implementation Differences
Despite shared principles, DI implementations in Angular and C# differ in approach, tools, and philosophy. Each solution reflects its context: Angular focuses on client apps, while .NET targets web, desktop, and more. Let’s explore the details.
Resolvers and Dependency Types
A key difference lies in how dependencies are defined and registered.
-
Angular uses
Injection Tokens
extensively. These unique keys explicitly identify dependencies, crucial for architectures with multiple implementations of the same interface or when default types fall short.
Example:
const MY_TOKEN = new InjectionToken<string>('myToken');
providers: [
{ provide: MY_TOKEN, useValue: 'Angular DI' }
]
This strict, flexible approach prevents runtime errors.
C# relies on interfaces and their implementations. Dependencies are registered as interface-to-class mappings:
services.AddTransient<IMyService, MyService>();
The process is linear, without tokens or additional layers.
Areas of DI Application
At the level of DI application, it becomes evident how Angular and C# differ in their approaches.
In Angular, DI is deeply integrated into the architecture. Developers cannot bypass it during development. Services, components, and even directives interact through injections, making DI an inseparable part of the ecosystem. The framework is fundamentally structured around this mechanism.
In C#, DI is more of a recommended practice than a built-in language or platform feature. Developers can build projects without DI containers. However, most modern .NET frameworks (e.g., ASP.NET Core) include native DI support.
This distinction determines how systematically DI is adopted: Angular enforces a universal model, while C# offers flexibility.
Dependency on Build/Runtime Execution
Another conceptual difference lies in how dependencies are handled during compilation versus runtime.
In C#, dependencies are resolved dynamically at runtime. Developers define dependencies in the IoC container, and the system instantiates objects on the fly. This can lead to runtime errors (e.g., misconfigured or missing dependencies). While flexible, this approach demands vigilance.
In Angular, DI is strictly controlled at compile time due to strong typing. Tools like
Injection Tokens
, decorators, and Angular’s compilation process detect DI issues before the app runs. For example, omitting a provider for a token will prevent the app from compiling.
This makes Angular more rigorous, reducing runtime error risks. In contrast, C#’s runtime flexibility is advantageous for dynamic or complex scenarios.
While Angular and C# share DI’s core architectural principles, their implementations diverge contextually. Angular emphasizes systematicity and safety through tokens, strong typing, and compile-time checks. C# prioritizes flexibility and dynamism, typical of a general-purpose platform. Recognizing these differences helps select the right tool for specific tasks.
Benefits of Learning DI in Both Ecosystems
Mastering diverse approaches and tools builds confidence in designing and implementing complex systems. Beyond technologies, it hones critical skills.
Expanding Professional Horizons and Architectural Skills
Understanding DI across ecosystems reveals multiple solutions to the same problem. It deepens knowledge of patterns like IoC (Inversion of Control), modularity, and loose coupling. Working with Angular and C# immerses developers in two domains: client-side apps with rich UIs and server-side systems with intricate logic.
This knowledge extends beyond DI:
- Recognizing when strict typing limits design freedom versus preventing errors.
- Evaluating trade-offs between runtime and compile-time solutions.
- Selecting optimal architectures for specific projects.
Designing Cross-Platform Architectures
In an era where client-server boundaries blur, DI expertise in Angular and C# enables seamless cross-platform solutions.
Examples include:
- A .NET backend with REST API paired with an Angular frontend, ensuring clear separation of concerns and unified dependency handling.
- Complex apps (e.g., backend serving web, desktop, and mobile) that avoid redundancy through combined approaches.
The result is loosely coupled, testable, and extensible architectures. Such systems are easier to maintain, scale, and hand over to teams.
Advancing Testing and Design Practices
Testability is a cornerstone of modern development. DI simplifies testing by decoupling components. Learning DI logic is pivotal for designing testable systems and writing effective tests.
Mastering DI in Angular and C# transcends library or tool proficiency. It cultivates professional skills: architectural design, testing, and adapting best practices across platforms. Developers gain a holistic perspective, enabling them to combine strategies and solve real-world challenges effectively.
Conclusion
Dependency Injection (DI) is more than just a tool—it’s a development philosophy that remains consistent whether you’re working with Angular or C#. Principles like separation of concerns, inversion of control, and testability create flexible architectures and maintainable code.
When working with both ecosystems, it becomes clear that DI universally strives for the same goals but achieves them differently. Angular impresses with its strict typing and deep integration, while C# excels in flexibility and architectural freedom. It’s in these nuances that the magic of working across platforms truly lies.
Final Thoughts
There’s a simple truth: the more we study and compare technologies, the better we understand their strengths and weaknesses. DI teaches us to embrace complex systems and experiment with new approaches. There’s no one-size-fits-all formula here—everything depends on your tasks, vision, and the ecosystem you operate in.
How does your experience with DI in C# differ from Angular? Where do you feel more freedom, and where do you encounter rigidity? Your insights are invaluable to the discussion.
If you have ideas or topics you’d like to explore, don’t hesitate to share them. Dialogue strengthens us, and your experience could spark new discoveries.
Thank you for reading! I’d love to hear your thoughts, questions, or suggestions—not just about DI, but about architecture in general. Let’s move forward together, advancing our knowledge and professional skills.