Building a Scalable Backend with NestJS Microservices: A Blueprint for Modern Architecture
In today’s fast-evolving tech landscape, scalability, modularity, and performance are the cornerstones of a robust backend system. Whether you’re building for a startup or an enterprise-grade application, having an architecture that can grow with your needs is non-negotiable. That’s why I’m excited to share a sample project I’ve been working on: a highly scalable backend architecture built using NestJS microservices. This repository brings together some of the most powerful tools and patterns in modern development—Repository Pattern, Prisma ORM, GraphQL, and gRPC with Protobuf—to create a blueprint for adaptable, production-ready systems. You can explore the full implementation here: GitHub Repository. Let’s dive into what makes this project tick and why it might be the perfect starting point for your next backend endeavor. Why NestJS Microservices? NestJS is a progressive Node.js framework that combines the best of TypeScript, object-oriented programming, and functional paradigms. Its built-in support for microservices makes it an ideal choice for distributed systems. Unlike monolithic architectures, microservices allow you to break your application into smaller, independent services that can be developed, deployed, and scaled separately. This project leverages NestJS’s microservices capabilities to demonstrate how you can achieve modularity without sacrificing performance or maintainability. Core Features of the Architecture 1. Repository Pattern for Clean Data Access The Repository Pattern is a time-tested approach to abstracting data access logic from your business logic. In this project, I’ve implemented it to create a clean separation between the database layer and the service layer. This not only improves testability (think mocking repositories for unit tests) but also makes the codebase easier to maintain as it grows. Whether you’re swapping out databases or adding new data sources, the Repository Pattern keeps your code flexible and future-proof. 2. Prisma ORM for Database Magic Speaking of databases, this project integrates Prisma, a next-generation ORM that simplifies database interactions with a type-safe, intuitive API. Prisma handles everything from schema migrations to query generation, allowing you to focus on building features rather than wrestling with SQL. In this setup, Prisma powers the data layer, making it seamless to connect to PostgreSQL, MySQL, or any other supported database. 3. GraphQL for Flexible API Queries For the API layer, I’ve opted for GraphQL over traditional REST. Why? GraphQL’s ability to let clients request exactly the data they need eliminates over-fetching and under-fetching issues common in REST APIs. With NestJS’s built-in GraphQL module, setting up a schema-first or code-first approach is a breeze. This project showcases how GraphQL can serve as the glue between your frontend and microservices, offering a unified and efficient querying experience. 4. gRPC with Protobuf for High-Performance Communication Microservices need to talk to each other, and that’s where gRPC shines. Paired with Protocol Buffers (Protobuf), gRPC enables lightning-fast, strongly-typed communication between services. Unlike REST, which relies on JSON over HTTP, gRPC uses binary serialization and HTTP/2, making it perfect for performance-critical systems. In this repository, I’ve set up gRPC to handle cross-service communication, demonstrating how to define service contracts and implement them in a real-world scenario. What Makes This Project Scalable? Scalability isn’t just about handling more users—it’s about designing a system that can evolve. Here’s how this architecture delivers: Modular Design: Each microservice is self-contained, allowing you to scale specific components independently based on demand. Technology Agnostic: The Repository Pattern and Prisma make it easy to swap databases or add new services without rewriting core logic. Performance Optimized: gRPC ensures low-latency communication, while GraphQL reduces API overhead. Extensible: NestJS’s dependency injection and modular structure mean you can plug in new features or services with minimal friction. Who Is This For? This project is designed as a starting point for developers and teams looking to: Build a modern backend from scratch with best practices in mind. Experiment with microservices in a controlled, sample environment. Learn how to integrate cutting-edge tools like Prisma, GraphQL, and gRPC in a real-world context. Adapt a scalable architecture to their specific production needs—be it e-commerce, SaaS, or IoT. Getting Started Ready to dive in? Clone the repository and follow the setup instructions in the README to get it running locally. You’ll find detailed comments and documentation to guide you through the codebase. Play around with the GraphQL playground, tweak the gRPC services,
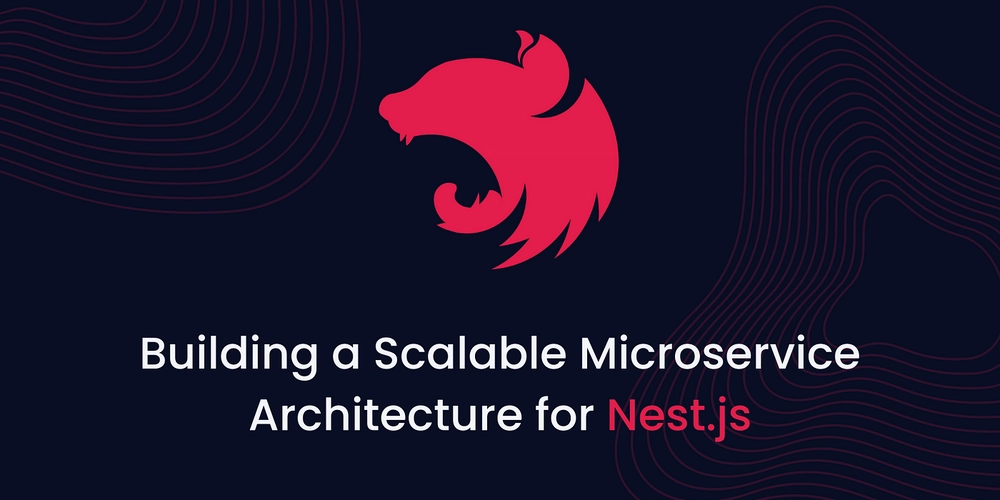
In today’s fast-evolving tech landscape, scalability, modularity, and performance are the cornerstones of a robust backend system. Whether you’re building for a startup or an enterprise-grade application, having an architecture that can grow with your needs is non-negotiable. That’s why I’m excited to share a sample project I’ve been working on: a highly scalable backend architecture built using NestJS microservices. This repository brings together some of the most powerful tools and patterns in modern development—Repository Pattern, Prisma ORM, GraphQL, and gRPC with Protobuf—to create a blueprint for adaptable, production-ready systems.
You can explore the full implementation here: GitHub Repository.
Let’s dive into what makes this project tick and why it might be the perfect starting point for your next backend endeavor.
Why NestJS Microservices?
NestJS is a progressive Node.js framework that combines the best of TypeScript, object-oriented programming, and functional paradigms. Its built-in support for microservices makes it an ideal choice for distributed systems. Unlike monolithic architectures, microservices allow you to break your application into smaller, independent services that can be developed, deployed, and scaled separately. This project leverages NestJS’s microservices capabilities to demonstrate how you can achieve modularity without sacrificing performance or maintainability.
Core Features of the Architecture
1. Repository Pattern for Clean Data Access
The Repository Pattern is a time-tested approach to abstracting data access logic from your business logic. In this project, I’ve implemented it to create a clean separation between the database layer and the service layer. This not only improves testability (think mocking repositories for unit tests) but also makes the codebase easier to maintain as it grows. Whether you’re swapping out databases or adding new data sources, the Repository Pattern keeps your code flexible and future-proof.
2. Prisma ORM for Database Magic
Speaking of databases, this project integrates Prisma, a next-generation ORM that simplifies database interactions with a type-safe, intuitive API. Prisma handles everything from schema migrations to query generation, allowing you to focus on building features rather than wrestling with SQL. In this setup, Prisma powers the data layer, making it seamless to connect to PostgreSQL, MySQL, or any other supported database.
3. GraphQL for Flexible API Queries
For the API layer, I’ve opted for GraphQL over traditional REST. Why? GraphQL’s ability to let clients request exactly the data they need eliminates over-fetching and under-fetching issues common in REST APIs. With NestJS’s built-in GraphQL module, setting up a schema-first or code-first approach is a breeze. This project showcases how GraphQL can serve as the glue between your frontend and microservices, offering a unified and efficient querying experience.
4. gRPC with Protobuf for High-Performance Communication
Microservices need to talk to each other, and that’s where gRPC shines. Paired with Protocol Buffers (Protobuf), gRPC enables lightning-fast, strongly-typed communication between services. Unlike REST, which relies on JSON over HTTP, gRPC uses binary serialization and HTTP/2, making it perfect for performance-critical systems. In this repository, I’ve set up gRPC to handle cross-service communication, demonstrating how to define service contracts and implement them in a real-world scenario.
What Makes This Project Scalable?
Scalability isn’t just about handling more users—it’s about designing a system that can evolve. Here’s how this architecture delivers:
- Modular Design: Each microservice is self-contained, allowing you to scale specific components independently based on demand.
- Technology Agnostic: The Repository Pattern and Prisma make it easy to swap databases or add new services without rewriting core logic.
- Performance Optimized: gRPC ensures low-latency communication, while GraphQL reduces API overhead.
- Extensible: NestJS’s dependency injection and modular structure mean you can plug in new features or services with minimal friction.
Who Is This For?
This project is designed as a starting point for developers and teams looking to:
- Build a modern backend from scratch with best practices in mind.
- Experiment with microservices in a controlled, sample environment.
- Learn how to integrate cutting-edge tools like Prisma, GraphQL, and gRPC in a real-world context.
- Adapt a scalable architecture to their specific production needs—be it e-commerce, SaaS, or IoT.
Getting Started
Ready to dive in? Clone the repository and follow the setup instructions in the README to get it running locally. You’ll find detailed comments and documentation to guide you through the codebase. Play around with the GraphQL playground, tweak the gRPC services, or extend the repository pattern to fit your use case.
Here’s the link again: NestJS Microservice Backend.
What’s Next?
This is just the beginning! I’m planning to add features like authentication, event-driven messaging with Kafka or RabbitMQ, and CI/CD pipelines to make this even more production-ready. Have ideas or suggestions? Feel free to open an issue or submit a PR—I’d love to collaborate!
Building scalable backends doesn’t have to be daunting. With the right tools and patterns, you can create systems that are both powerful and adaptable. I hope this project inspires you to explore the possibilities of NestJS microservices and take your backend development to the next level.
Happy coding!