Building a House Price Prediction Model with FastAPI and Deployment
In the previous post, we built a Random Forest model to predict house prices using the Toronto housing dataset. Now, let’s move forward by creating a FastAPI web application to serve this model and deploy it so others can interact with it. Below, I’ll guide you through the process of creating the FastAPI application, setting up Nginx as a reverse proxy, and deploying the model on AWS EC2. Setting Up the FastAPI Application First, we need to set up the FastAPI app that will serve our machine learning model. We’ll structure the app as follows: Install FastAPI and Uvicorn: pip install fastapi uvicorn Create the FastAPI App: In the app.py file, we will create a POST endpoint /predict that accepts the input features of the house and returns the predicted price using our trained model. from fastapi import FastAPI from pydantic import BaseModel import joblib import pandas as pd import numpy as np import logging # Load the trained model model_path = "models/random_forest_model.pkl" model = joblib.load(model_path) app = FastAPI() # Define the input data model class HouseFeatures(BaseModel): sqft: int bedrooms: int bathrooms: int parking: int mean_district_income: int @app.post("/predict") async def predict_house_price(data: HouseFeatures): try: input_data = data.dict() logging.info(f"
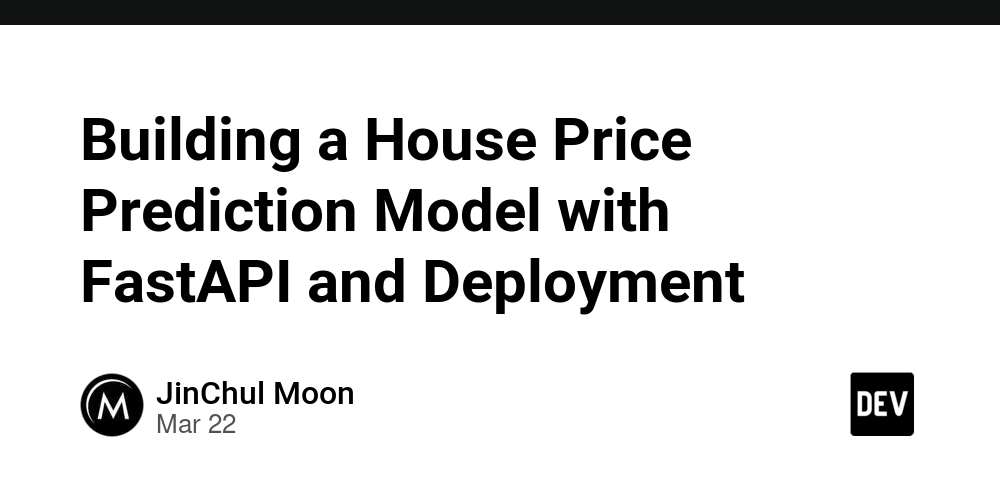
In the previous post, we built a Random Forest model to predict house prices using the Toronto housing dataset. Now, let’s move forward by creating a FastAPI web application to serve this model and deploy it so others can interact with it. Below, I’ll guide you through the process of creating the FastAPI application, setting up Nginx as a reverse proxy, and deploying the model on AWS EC2.
- Setting Up the FastAPI Application
First, we need to set up the FastAPI app that will serve our machine learning model. We’ll structure the app as follows:
- Install FastAPI and Uvicorn:
pip install fastapi uvicorn
- Create the FastAPI App:
In the app.py file, we will create a POST endpoint /predict that accepts the input features of the house and returns the predicted price using our trained model.
from fastapi import FastAPI
from pydantic import BaseModel
import joblib
import pandas as pd
import numpy as np
import logging
# Load the trained model
model_path = "models/random_forest_model.pkl"
model = joblib.load(model_path)
app = FastAPI()
# Define the input data model
class HouseFeatures(BaseModel):
sqft: int
bedrooms: int
bathrooms: int
parking: int
mean_district_income: int
@app.post("/predict")
async def predict_house_price(data: HouseFeatures):
try:
input_data = data.dict()
logging.info(f"