Beyond Blueprints: Seeing the Soul of Java's Classes and Objects
We've all heard the simplistic analogy: "A class is a blueprint, and an object is a house." While it offers a rudimentary starting point, it barely scratches the surface of the profound relationship between classes and objects in object-oriented programming, especially within the robust ecosystem of Java. Let's discard the oversimplifications and embark on a technical and philosophical exploration to truly grasp their significance in crafting resilient and elegant software. 1. The Technical Foundation: A Java Perspective Within the context of Java, classes and objects have particular meaning.They are not mere abstract concepts,they are fundamental building blocks with distinct roles at different stages of their lifecycle. Class: The template of Behavior and Structure A class in Java is a compile-time construct, a meticulous definition etched in your source code. It acts as a comprehensive template, dictating the very essence of the entities your program will manipulate. This template encompasses, State (variables):: These are the variables declared within a class (e.g., int age, String name). They represent the data that an object of this class will hold, defining its characteristics. Think of them as the attributes that describe an entity.instance variables define the precise memory footprint of each object. When a class is loaded, the JVM calculates field offsets based on type signatures in the class file - primitive types like int (4 bytes) and long (8 bytes) get fixed-width slots, while object references consume either 4 or 8 bytes depending on JVM configuration.Each object header (typically 12 bytes) contains a pointer to this layout metadata in the class's klass structure. Notably, static fields are treated differently,they stored in the class's mirror object in Metaspace, not within individual instances. Behavior (Methods):: These are the functions defined within a class (e.g., calculateSalary(), login()). They encapsulate the actions that an object of this class can perform, defining its capabilities and interactions in controlled manner.When a Java class is loaded, the JVM stores its bytecode and metadata, including the structure of fields and methods, within the Method Area. For classes with overridable instance methods, the JVM creates a vtable in the Method Area, which is an ordered array of pointers to the actual method implementations (either bytecode in the Method Area or compiled native code in the Code Cache). Similarly, classes implementing interfaces may have itables in the Method Area to facilitate interface method dispatch. Each object created on the heap contains a pointer to its class's metadata in the Method Area, allowing the JVM to access the appropriate vtable or itables. During method invocation on an object, the JVM uses the object's class pointer to locate the vtable or itable and then uses a precomputed index to find the memory address of the method's executable code, enabling dynamic dispatch and polymorphism. Static methods and constructors, not subject to dynamic dispatch, are also stored in the Method Area and are invoked directly using the class metadata. Instance fields reside within the object on the heap, while static fields are stored in the class's metadata in the Method Area. Identity (Constructors): These special methods dictate how a new object of the class is initialized. They set the initial state of an object when it is created, giving it its initial identity.At a raw, low-level, when new is executed, the JVM first allocates a contiguous block of raw bytes in the heap, the size determined by the class's field layout. This memory is initialized to zero. Then, the appropriate constructor's bytecode is executed. This bytecode directly manipulates the memory at specific byte offsets corresponding to the instance fields, writing the initial values provided in the constructor call or default values. The constructor might also call other methods, including the constructor of the superclass, which similarly manipulates the raw byte representation of the object's inherited fields. Finally, the new operation returns the memory address of this now-initialized block of bytes as a reference to the newly created object. Relationships (Inheritance, Interfaces): Java's extends and implements keywords within a class definition establish how this class relates to other classes and interfaces, enabling code reuse and polymorphism.At the JVM level, inheritance via extends creates a hierarchical vtable structure where method calls are resolved through virtual method table lookups - each class maintains a table of method slots, with subclasses copying and overriding parent entries, while invokevirtual opcodes use this table for dynamic dispatch. Interface implementation (implements) generates separate itables (interface method tables) that map interface methods to concrete implementations, resolved through invokeinterface which performs additional overhead
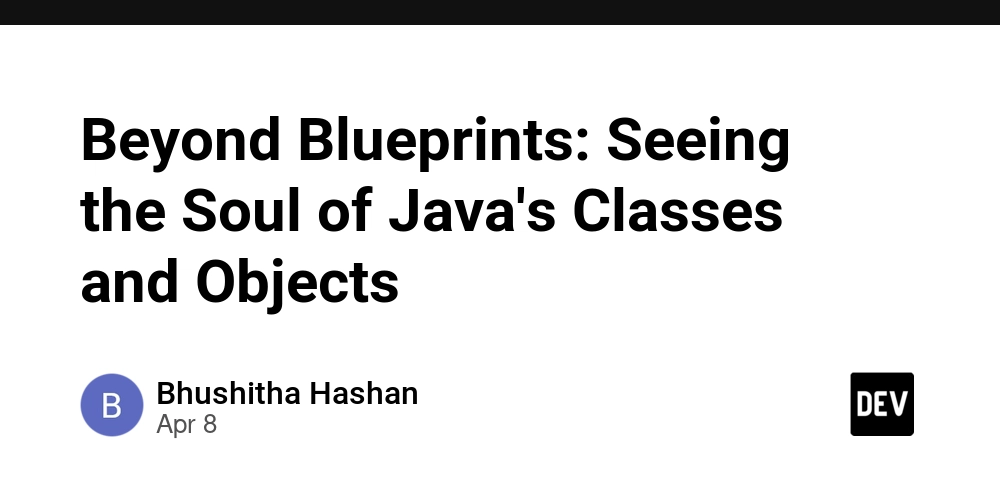
We've all heard the simplistic analogy: "A class is a blueprint, and an object is a house." While it offers a rudimentary starting point, it barely scratches the surface of the profound relationship between classes and objects in object-oriented programming, especially within the robust ecosystem of Java. Let's discard the oversimplifications and embark on a technical and philosophical exploration to truly grasp their significance in crafting resilient and elegant software.
1. The Technical Foundation: A Java Perspective
Within the context of Java, classes and objects have particular meaning.They are not mere abstract concepts,they are fundamental building blocks with distinct roles at different stages of their lifecycle.
Class: The template of Behavior and Structure
A class in Java is a compile-time construct, a meticulous definition etched in your source code. It acts as a comprehensive template, dictating the very essence of the entities your program will manipulate. This template encompasses,
State (variables):: These are the variables declared within a class (e.g.,
int age
,String name
). They represent the data that an object of this class will hold, defining its characteristics. Think of them as the attributes that describe an entity.instance variables define the precise memory footprint of each object. When a class is loaded, the JVM calculates field offsets based on type signatures in the class file - primitive types like int (4 bytes) and long (8 bytes) get fixed-width slots, while object references consume either 4 or 8 bytes depending on JVM configuration.Each object header (typically 12 bytes) contains a pointer to this layout metadata in the class's klass structure. Notably, static fields are treated differently,they stored in the class's mirror object in Metaspace, not within individual instances.Behavior (Methods):: These are the functions defined within a class (e.g.,
calculateSalary()
,login()
). They encapsulate the actions that an object of this class can perform, defining its capabilities and interactions in controlled manner.When a Java class is loaded, the JVM stores its bytecode and metadata, including the structure of fields and methods, within the Method Area. For classes with overridable instance methods, the JVM creates a vtable in the Method Area, which is an ordered array of pointers to the actual method implementations (either bytecode in the Method Area or compiled native code in the Code Cache). Similarly, classes implementing interfaces may have itables in the Method Area to facilitate interface method dispatch. Each object created on the heap contains a pointer to its class's metadata in the Method Area, allowing the JVM to access the appropriate vtable or itables. During method invocation on an object, the JVM uses the object's class pointer to locate the vtable or itable and then uses a precomputed index to find the memory address of the method's executable code, enabling dynamic dispatch and polymorphism. Static methods and constructors, not subject to dynamic dispatch, are also stored in the Method Area and are invoked directly using the class metadata. Instance fields reside within the object on the heap, while static fields are stored in the class's metadata in the Method Area.Identity (Constructors): These special methods dictate how a new object of the class is initialized. They set the initial state of an object when it is created, giving it its initial identity.At a raw, low-level, when
new
is executed, the JVM first allocates a contiguous block of raw bytes in the heap, the size determined by the class's field layout. This memory is initialized to zero. Then, the appropriate constructor's bytecode is executed. This bytecode directly manipulates the memory at specific byte offsets corresponding to the instance fields, writing the initial values provided in the constructor call or default values. The constructor might also call other methods, including the constructor of the superclass, which similarly manipulates the raw byte representation of the object's inherited fields. Finally, thenew
operation returns the memory address of this now-initialized block of bytes as a reference to the newly created object.Relationships (Inheritance, Interfaces): Java's
extends
andimplements
keywords within a class definition establish how this class relates to other classes and interfaces, enabling code reuse and polymorphism.At the JVM level, inheritance via extends creates a hierarchical vtable structure where method calls are resolved through virtual method table lookups - each class maintains a table of method slots, with subclasses copying and overriding parent entries, while invokevirtual opcodes use this table for dynamic dispatch. Interface implementation (implements) generates separate itables (interface method tables) that map interface methods to concrete implementations, resolved through invokeinterface which performs additional overhead for interface method resolution including checking all implemented interfaces.
Finally A class exists solely in your source code. It is the input for the Java compiler, which then translates this human readable definition into bytecode the language understood by the Java Virtual Machine (JVM).the moment your program runs and touches that class, the JVM brings it to life in memory. It builds this whole shadow version of your class in Metaspace.Method tables, reflection junk,Constant Pool Resolution,Class Hierarchy Info ,JIT Optimization Hooks which the Just-In-Time compiler uses to aggressively optimize hot code paths etc are few.
Object: The Living Instance
An object, on the other hand, is a runtime instance of a class. It is the tangible manifestation of the blueprint defined by the class. When your Java program runs, objects come to life, occupying precious memory within the heap.
When a new object comes into being within the JVM's heap, it carves out a specific chunk of raw bytes from the available memory. The size of this chunk isn't a simple tally of its declared fields; it also includes a bit of extra baggage known as the object header. This header is like an internal ID tag, holding crucial low-level information. One part, the "mark word," carries bits that track things like the object's unique hash code, its status for garbage collection, and any active locking information. Another vital part is the "klass pointer," which is essentially a direct memory address acting like a signpost, pointing the JVM back to the detailed blueprint of the object's class stored in the Metaspace.
Right after this header, the actual data fields of the object reside. For basic data types like integers and long numbers, their raw binary values are laid out directly in the allocated memory, starting at specific positions (offsets). The JVM decides these positions based on a careful arrangement process that considers the order in which the fields are declared and also any alignment rules that the computer's processor might require for optimal performance.
When you tell an object to do something (invoke a method), the special this
keyword you often see is, at its heart, just the raw memory address where that object lives in the heap. The JVM takes this address, along with the name of the method you called, and uses the "klass pointer" from the object's header to find the class's instructions. Within these instructions, it locates the vtable (for regular methods) or itable (for interface methods). These tables are like internal address books, containing the raw memory locations of the actual machine code that makes the method run. Finally, the object's unique "identity" at the most fundamental level is deeply connected to its unique starting address within the vast landscape of the heap, and the hashCode()
method often cleverly uses this address or some derived value to give you a more human-friendly integer representation of that uniqueness.
2. The Philosophical and Design Significance: Beyond the Code
Stepping aside the technical specifications,Now let's delve into the philosophical and design implications of classes and objects, understanding their true meaning in crafting robust and maintainable software.
Class as a "Rulebook": The Abstract Definition of an Idea
Think of a Java class as a strict and detailed rulebook. It lays out exactly what kind of data its objects will hold.like numbers, strings, or lists — and what actions they’ll be able to perform,like depositing money or printing details. This isn’t a gentle suggestion; it’s a contract. Every object created from that class must follow these rules without exception. The Java Virtual Machine (JVM) enforces this behind the scenes, ensuring that your objects behave predictably and consistently.
Let’s take a real-world example: a BankAccount class.
This class doesn’t just suggest that a bank account should have a balance and an accountNumber. It defines them as essential parts of what makes a bank account a bank account. It also outlines specific operations, like deposit(), withdraw(), and getBalance(). These aren't optional features — they are the core capabilities every BankAccount object must have.
But there’s more. A good class often comes with invariants — unbreakable rules that must always hold true. For instance, a BankAccount might require that the balance can never drop below zero, and a withdrawal can only happen if there’s enough money in the account. These built-in checks act like safety rails, preventing your objects from getting into illogical or invalid states.
Object as a "Tangible Entity": The Concrete Realization
Now here’s where it gets more interesting.
If a class is the rulebook, then an object is a living, breathing copy created by following those rules. When your program runs and creates a new BankAccount, that’s no longer an idea — it’s a real instance in memory, with its own data and its own life. It might have a balance of $500 and an account number like 12345. You can interact with it: deposit money, withdraw cash, or check its balance.
And here’s the cool part,if you also have a bank account in the program, it’s built from the same blueprint but lives a completely separate life. Your account might have $2,000, and mine $500. Even though both are BankAccount objects, each one has its own memory, its own values, and its own identity.
Just like two houses built from the same blueprint can have different paint colors and furniture, two objects of the same class can have different data, but still obey the same structural rules. That’s the power of object-oriented programming.
Finally,A well-designed class becomes a promise. It says, “Every object built from me will behave in a certain way and hold certain data and no surprises.” That kind of consistency is what allows large, complex programs to stay manageable.
So the next time you define a class, remember, you're not just typing out some syntax. You're crafting a set of rules that will shape real, living things in your program's world.Each one with its own identity, yet all bound by the same laws you set.
And when you create an object, you’re not just allocating memory,you're giving life to an idea.