Beginner’s Guide to Writing Clean Java Code
When you first start learning Java, it’s easy to focus solely on getting things to work. If a program runs, it’s considered a success. However, as you grow in your programming journey, you’ll quickly realize that how your Java code is written matters just as much as whether it works. Writing clean Java code is an essential skill that will set you apart and make your work more maintainable, readable, and professional. Why Clean Code Matters Clean Java code is not just about making things look pretty. It’s about making your code easier to read, understand, and modify. As a beginner, you might be the only one working on your projects for now, but in any real-world software development environment, you’ll likely collaborate with others. Clean code ensures that your logic can be followed and your programs can be extended or debugged without frustration. Think of your code as a form of communication, not just with machines, but with other developers—and your future self. When you revisit a project after a few weeks or months, clean and well-structured Java code will help you pick up where you left off without confusion. Start with Meaningful Naming One of the simplest but most powerful ways to clean up your Java code is by using meaningful names. This includes naming your classes, methods, and variables clearly to describe their purpose. Avoid short and cryptic names; instead, opt for descriptive terms that make the code self-explanatory. For example, a variable that stores a user’s age should be named something like userAge, not just a or x. This simple practice significantly enhances the readability of your code and makes it easier to understand at a glance. Stay Organized with Consistent Formatting Consistency is key in writing clean Java code. Follow a clear and consistent formatting style. This includes proper indentation, spacing, and organizing code blocks logically. Using a consistent style not only helps your code look better but also allows you and others to understand its structure more easily. Modern IDEs (Integrated Development Environments) like IntelliJ IDEA or Eclipse often come with formatting tools that help you stick to standard practices. These tools are especially useful for beginners who are still getting used to writing neatly structured code. Embrace Comments — But Use Them Wisely Comments are a helpful way to explain why something is done a certain way in your Java code, especially when the logic isn't immediately obvious. However, over-commenting or adding obvious explanations can clutter the file. Aim to write your Java code so clearly that it barely needs comments. When you do use them, focus on explaining the reasoning behind complex decisions, rather than what the code is doing line by line. Keep Methods Focused and Short Clean Java code is often made up of small, focused methods that do one thing well. Long and overly complex methods are harder to test, debug, and understand. By keeping each method dedicated to a single task, you increase modularity and improve the overall design of your program. Additionally, short methods are easier to reuse and reduce duplication, which is another sign of clean and efficient code. Avoid Hard-Coding Values Using hard-coded values (sometimes called "magic numbers") throughout your Java code makes it harder to change and understand. Instead, define constants or configuration parameters that can be easily updated and reused. This practice not only improves readability but also makes your code more flexible and maintainable in the long run. Stick to Java JDK Standards The Java JDK (Java Development Kit) provides standard libraries and conventions that serve as the foundation of all Java development. As a beginner, familiarize yourself with the standard practices included in the Java JDK. Using features and tools provided by the Java JDK ensures compatibility and helps you write code that aligns with widely accepted patterns. Relying on well-documented Java JDK components can also reduce the chances of introducing bugs or unnecessary complexity into your programs. Refactor Often Refactoring means restructuring existing Java code without changing its external behavior. This could involve renaming variables for clarity, splitting up large methods, or removing unnecessary code. Refactoring regularly ensures that your code stays clean and organized as the project evolves. It’s a good habit to pause occasionally during development to review your code and make small improvements. Don’t wait until your program becomes too large and messy—cleaning up as you go saves time and frustration later. Conclusion Writing clean Java code is a mindset as much as it is a skill. It’s about being thoughtful, consistent, and considerate—not just about getting a program to run. As a beginner, developing good habits early on will make you a stronger and more rel
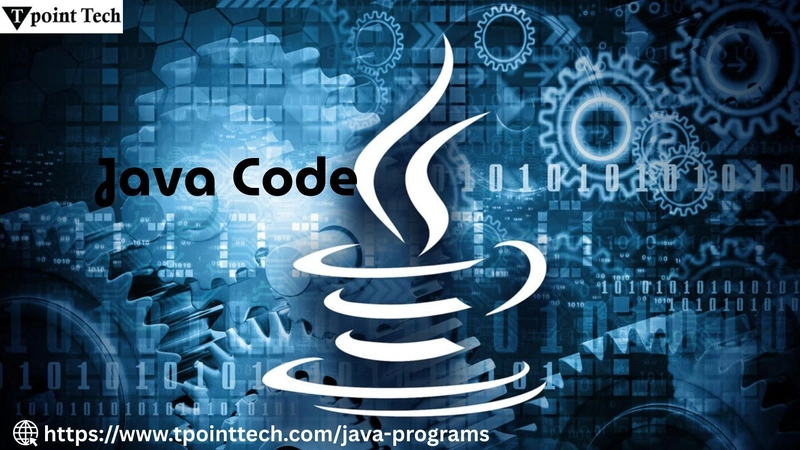
When you first start learning Java, it’s easy to focus solely on getting things to work. If a program runs, it’s considered a success. However, as you grow in your programming journey, you’ll quickly realize that how your Java code is written matters just as much as whether it works. Writing clean Java code is an essential skill that will set you apart and make your work more maintainable, readable, and professional.
Why Clean Code Matters
Clean Java code is not just about making things look pretty. It’s about making your code easier to read, understand, and modify. As a beginner, you might be the only one working on your projects for now, but in any real-world software development environment, you’ll likely collaborate with others. Clean code ensures that your logic can be followed and your programs can be extended or debugged without frustration.
Think of your code as a form of communication, not just with machines, but with other developers—and your future self. When you revisit a project after a few weeks or months, clean and well-structured Java code will help you pick up where you left off without confusion.
Start with Meaningful Naming
One of the simplest but most powerful ways to clean up your Java code is by using meaningful names. This includes naming your classes, methods, and variables clearly to describe their purpose. Avoid short and cryptic names; instead, opt for descriptive terms that make the code self-explanatory.
For example, a variable that stores a user’s age should be named something like userAge, not just a or x. This simple practice significantly enhances the readability of your code and makes it easier to understand at a glance.
Stay Organized with Consistent Formatting
Consistency is key in writing clean Java code. Follow a clear and consistent formatting style. This includes proper indentation, spacing, and organizing code blocks logically. Using a consistent style not only helps your code look better but also allows you and others to understand its structure more easily.
Modern IDEs (Integrated Development Environments) like IntelliJ IDEA or Eclipse often come with formatting tools that help you stick to standard practices. These tools are especially useful for beginners who are still getting used to writing neatly structured code.
Embrace Comments — But Use Them Wisely
Comments are a helpful way to explain why something is done a certain way in your Java code, especially when the logic isn't immediately obvious. However, over-commenting or adding obvious explanations can clutter the file.
Aim to write your Java code so clearly that it barely needs comments. When you do use them, focus on explaining the reasoning behind complex decisions, rather than what the code is doing line by line.
Keep Methods Focused and Short
Clean Java code is often made up of small, focused methods that do one thing well. Long and overly complex methods are harder to test, debug, and understand. By keeping each method dedicated to a single task, you increase modularity and improve the overall design of your program.
Additionally, short methods are easier to reuse and reduce duplication, which is another sign of clean and efficient code.
Avoid Hard-Coding Values
Using hard-coded values (sometimes called "magic numbers") throughout your Java code makes it harder to change and understand. Instead, define constants or configuration parameters that can be easily updated and reused. This practice not only improves readability but also makes your code more flexible and maintainable in the long run.
Stick to Java JDK Standards
The Java JDK (Java Development Kit) provides standard libraries and conventions that serve as the foundation of all Java development. As a beginner, familiarize yourself with the standard practices included in the Java JDK.
Using features and tools provided by the Java JDK ensures compatibility and helps you write code that aligns with widely accepted patterns. Relying on well-documented Java JDK components can also reduce the chances of introducing bugs or unnecessary complexity into your programs.
Refactor Often
Refactoring means restructuring existing Java code without changing its external behavior. This could involve renaming variables for clarity, splitting up large methods, or removing unnecessary code. Refactoring regularly ensures that your code stays clean and organized as the project evolves.
It’s a good habit to pause occasionally during development to review your code and make small improvements. Don’t wait until your program becomes too large and messy—cleaning up as you go saves time and frustration later.
Conclusion
Writing clean Java code is a mindset as much as it is a skill. It’s about being thoughtful, consistent, and considerate—not just about getting a program to run. As a beginner, developing good habits early on will make you a stronger and more reliable developer over time.
Clean code leads to better collaboration, fewer bugs, and easier updates. By focusing on clarity, simplicity, and standard practices like those offered by the Java JDK, you’ll be well on your way to writing Java code that not only works but stands the test of time.