Angular 16–19: Understanding `input.required<T>()` vs `input.required<T>().signal`
In Angular 16+ and 19, the new functional input API using input.required() is a powerful way to declare inputs in standalone and signal-based components. But many developers encounter this error: Property 'signal' does not exist on type 'InputSignal' So what’s the difference between: characters = input.required(); and characters = input.required().signal; Let’s break it down. ✅ input.required() This is the official API introduced in Angular 16 and fully stabilized in Angular 19. What it does: Declares a required input. Returns an InputSignal. Can be used like a Signal, but is a distinct type. Example: characters = input.required(); ngOnInit() { console.log(this.characters()); // works like a signal } ✔️ This works in Angular 16, 17, 18, and 19. ❌ input.required().signal (Invalid) In some blog posts or GitHub examples, you might see .signal added: characters = input.required().signal; This throws: Error: Property 'signal' does not exist on type 'InputSignal' Why? The .signal accessor was considered for future versions of Angular but: ❌ It is not implemented as of Angular 19. ❌ InputSignal is already reactive — no need to convert it. ✅ How to Use InputSignal Properly characters = input.required(); const characterCount = computed(() => this.characters().length); Or inside the template: { '{' } c.name { '}' } Angular tracks changes reactively — no .signal needed! Summary Syntax Description Works in Angular 16–19? input.required() Returns a reactive InputSignal ✅ Yes input.required().signal ❌ Invalid: .signal not implemented ❌ No Final Thoughts Angular’s input.required() is already reactive. Treat it like a signal: Call .() to get its current value. Use it in computed signals or templates. No need for .signal. If you see .signal, you're likely reading about an experimental or unofficial version. Stick to input.required() — it’s stable, supported, and reactive by design. Happy coding with Signals and Functional Inputs! ⚡
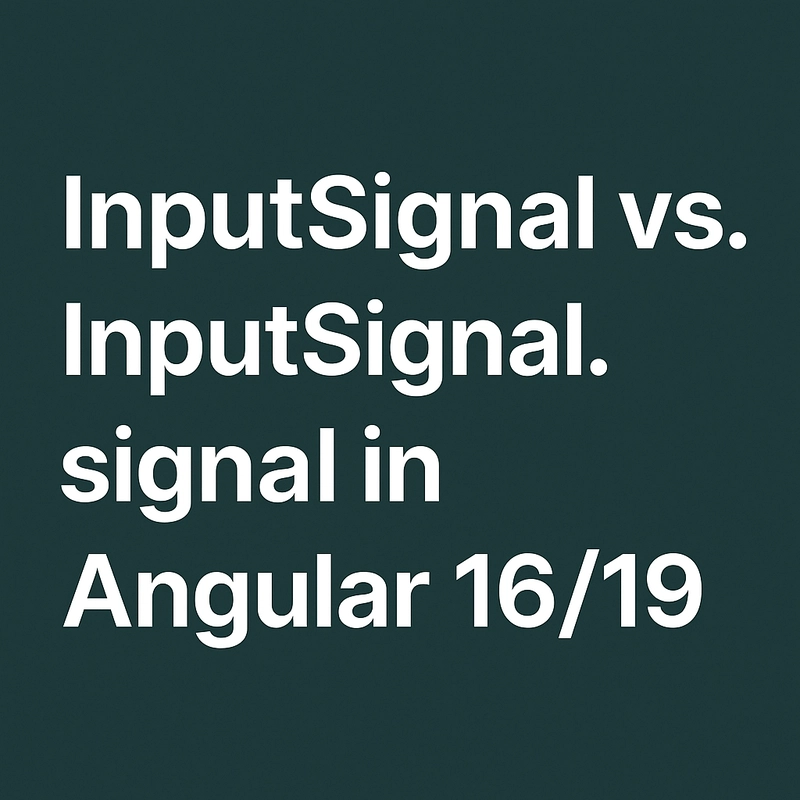
In Angular 16+ and 19, the new functional input API using input.required
is a powerful way to declare inputs in standalone and signal-based components. But many developers encounter this error:
Property 'signal' does not exist on type 'InputSignal'
So what’s the difference between:
characters = input.required<Character[]>();
and
characters = input.required<Character[]>().signal;
Let’s break it down.
✅ input.required()
This is the official API introduced in Angular 16 and fully stabilized in Angular 19.
What it does:
- Declares a required input.
- Returns an
InputSignal
. -
Can be used like a
Signal
, but is a distinct type.
Example:
characters = input.required<Character[]>();
ngOnInit() {
console.log(this.characters()); // works like a signal
}
✔️ This works in Angular 16, 17, 18, and 19.
❌ input.required().signal
(Invalid)
In some blog posts or GitHub examples, you might see .signal
added:
characters = input.required<Character[]>().signal;
This throws:
Error: Property 'signal' does not exist on type 'InputSignal'
Why?
The .signal
accessor was considered for future versions of Angular but:
- ❌ It is not implemented as of Angular 19.
- ❌
InputSignal
is already reactive — no need to convert it.
✅ How to Use InputSignal
Properly
characters = input.required<Character[]>();
const characterCount = computed(() => this.characters().length);
Or inside the template:
*ngFor="let c of characters()">{ '{' } c.name { '}' }
Angular tracks changes reactively — no .signal
needed!
Summary
Syntax | Description | Works in Angular 16–19? |
---|---|---|
input.required |
Returns a reactive InputSignal
|
✅ Yes |
input.required |
❌ Invalid: .signal not implemented |
❌ No |
Final Thoughts
Angular’s input.required
is already reactive. Treat it like a signal:
- Call
.()
to get its current value. - Use it in computed signals or templates.
- No need for
.signal
.
If you see
.signal
, you're likely reading about an experimental or unofficial version. Stick toinput.required
— it’s stable, supported, and reactive by design.()
Happy coding with Signals and Functional Inputs! ⚡