A Deep Dive into JavaScript Classes: Syntax, Constructors, Fields, and More
In the previous blog post, we discussed the difference between constructors and classes in JavaScript. In this blog and throughout the series, I'll delve deeper into classes. I realized that understanding classes is crucial, and instead of just mentioning syntax, I should explore each feature in detail. In this blog, I'll cover the following topics: Class Syntax Types of Constructors Class Fields Getters and Setters Review of Methods According to MDN, Classes are a template for creating objects. They encapsulate data with code to work on that data. Classes in JS are built on prototypes but also have some syntax and semantics that are unique to classes. MDN Class Syntax class MyClass { // class methods constructor() { ... } method1() { ... } method2() { ... } method3() { ... } ... } The Modern JavaScript Tutorial We use the new operator to create an object from the class: let user = new User("John"); When using the new operator, the constructor implicitly creates the this object and returns it. Types of Constructors Like other functions, classes can be declared in two ways: class declarations and class expressions. // Declaration class Rectangle { constructor(height, width) { this.height = height; this.width = width; } } // Expression; the class is anonymous but assigned to a variable const Rectangle = class { constructor(height, width) { this.height = height; this.width = width; } }; // Expression; the class has its own name const Rectangle = class Rectangle2 { constructor(height, width) { this.height = height; this.width = width; } }; MDN Class Fields The class syntax introduces a new feature: fields. A class field is a property that belongs to the instance of the class, not to its prototype. class User { name = "John"; // Field sayHi() { console.log(`Hi, ${this.name}!`); // Method } } let user = new User(); console.log(user.name); // John console.log(User.prototype.name); //undefined Getters and Setters In classes, we can define get and set accessors, which are shared by all instances. These allow controlled access to a property while maintaining encapsulation. class User { constructor (name) { this._name = name; } get name () { return this._name; } set name (value) { if (value.length < 4) { console.log("Name is too short."); return; } this._name = value; } } The example above is modified by adding "_" to private fields, which is a common convention. The Modern JavaScript Tutorial Review of Methods There are several ways to declare methods. Below is a summary of each syntax and its usage. Arrow Function: foo: () => "bar"; This is a property of an object, and this is inherited from its lexical scope. However, this is not a class method because it does not belong to a class prototype. Object Property: foo: function () { return "bar"; } This defines a method as a property of an object. this is bound to the calling object. However, this is a property on the instance, not on the prototype. Class Method Syntax: foo () { return "bar"; } This is the standard syntax for class methods. Class methods are shared across instances of the class. Additionally, this is automatically bound to the instance when the method is called. Getter and setter properties are defined on the prototype, so they are shared by instances of the class.
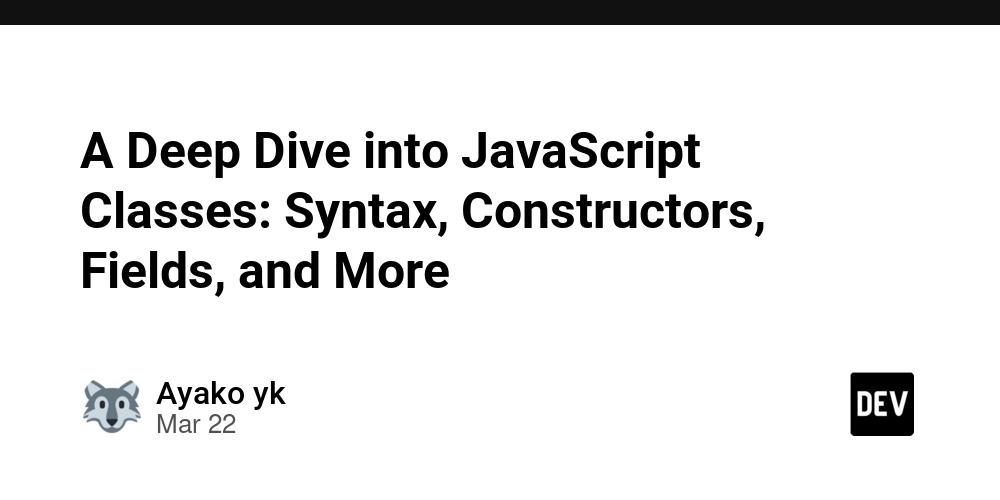
In the previous blog post, we discussed the difference between constructors and classes in JavaScript. In this blog and throughout the series, I'll delve deeper into classes. I realized that understanding classes is crucial, and instead of just mentioning syntax, I should explore each feature in detail.
In this blog, I'll cover the following topics:
- Class Syntax
- Types of Constructors
- Class Fields
- Getters and Setters
- Review of Methods
According to MDN,
Classes are a template for creating objects. They encapsulate data with code to work on that data. Classes in JS are built on prototypes but also have some syntax and semantics that are unique to classes.
MDN
Class Syntax
class MyClass {
// class methods
constructor() { ... }
method1() { ... }
method2() { ... }
method3() { ... }
...
}
The Modern JavaScript Tutorial
We use the new
operator to create an object from the class:
let user = new User("John");
When using the new
operator, the constructor implicitly creates the this
object and returns it.
Types of Constructors
Like other functions, classes can be declared in two ways: class declarations and class expressions.
// Declaration
class Rectangle {
constructor(height, width) {
this.height = height;
this.width = width;
}
}
// Expression; the class is anonymous but assigned to a variable
const Rectangle = class {
constructor(height, width) {
this.height = height;
this.width = width;
}
};
// Expression; the class has its own name
const Rectangle = class Rectangle2 {
constructor(height, width) {
this.height = height;
this.width = width;
}
};
Class Fields
The class syntax introduces a new feature: fields. A class field is a property that belongs to the instance of the class, not to its prototype.
class User {
name = "John"; // Field
sayHi() {
console.log(`Hi, ${this.name}!`); // Method
}
}
let user = new User();
console.log(user.name); // John
console.log(User.prototype.name); //undefined
Getters and Setters
In classes, we can define get
and set
accessors, which are shared by all instances. These allow controlled access to a property while maintaining encapsulation.
class User {
constructor (name) {
this._name = name;
}
get name () {
return this._name;
}
set name (value) {
if (value.length < 4) {
console.log("Name is too short.");
return;
}
this._name = value;
}
}
The example above is modified by adding "_" to private fields, which is a common convention.
The Modern JavaScript Tutorial
Review of Methods
There are several ways to declare methods. Below is a summary of each syntax and its usage.
Arrow Function:
foo: () => "bar";
This is a property of an object, and this
is inherited from its lexical scope.
However, this is not a class method because it does not belong to a class prototype.
Object Property:
foo: function () { return "bar"; }
This defines a method as a property of an object. this
is bound to the calling object. However, this is a property on the instance, not on the prototype.
Class Method Syntax:
foo () { return "bar"; }
This is the standard syntax for class methods. Class methods are shared across instances of the class. Additionally, this
is automatically bound to the instance when the method is called.
Getter and setter properties are defined on the prototype, so they are shared by instances of the class.