User-Defined Exceptions in Java
In Java, you can create your own exception classes to represent specific error conditions in your application. These are called user-defined or custom exceptions. Why Create Custom Exceptions? To represent application-specific error conditions To provide more meaningful exception messages To include additional error information To create a hierarchy of exceptions specific to your domain How to Create Custom Exceptions There are two main types of exceptions you can create: 1. Checked Exceptions (extend Exception) These must be declared in the method signature or handled with try-catch. 2. Unchecked Exceptions (extend RuntimeException) These don't need to be declared or caught. Creating a Custom Exception Basic Structure // For checked exception class MyException extends Exception { public MyException(String message) { super(message); } } // For unchecked exception class MyRuntimeException extends RuntimeException { public MyRuntimeException(String message) { super(message); } } Complete Example // Custom checked exception class InvalidAgeException extends Exception { public InvalidAgeException(String message) { super(message); } } // Custom unchecked exception class InsufficientFundsException extends RuntimeException { private double balance; private double amount; public InsufficientFundsException(double balance, double amount) { super("Insufficient funds: Attempted to withdraw " + amount + " but only " + balance + " available"); this.balance = balance; this.amount = amount; } public double getBalance() { return balance; } public double getAmount() { return amount; } } public class BankAccount { private double balance; public BankAccount(double balance) { this.balance = balance; } public void withdraw(double amount) throws InvalidAgeException { if (amount balance) { throw new InsufficientFundsException(balance, amount); } balance -= amount; } public static void main(String[] args) { BankAccount account = new BankAccount(1000); try { account.withdraw(1500); // This will throw InsufficientFundsException } catch (InvalidAgeException e) { System.out.println("Error: " + e.getMessage()); } try { account.withdraw(-100); // This will throw InvalidAgeException } catch (InvalidAgeException e) { System.out.println("Error: " + e.getMessage()); } } } Best Practices for Custom Exceptions Naming: End your exception class names with "Exception" (e.g., InvalidInputException) Constructors: Provide at least a constructor that takes a String message Serialization: For serializable exceptions, declare a serialVersionUID: private static final long serialVersionUID = 1L; Documentation: Document when your exception should be thrown using @throws in Javadoc Avoid overuse: Only create custom exceptions when standard Java exceptions don't suffice When to Use Custom Exceptions When you need to pass additional information about the error When you want to catch specific exceptions in your application When you want to create a hierarchy of related exceptions When standard Java exceptions don't precisely describe your error condition Remember that custom exceptions should follow the same general principles as Java's built-in exceptions - they should be used for exceptional conditions, not for normal flow control.
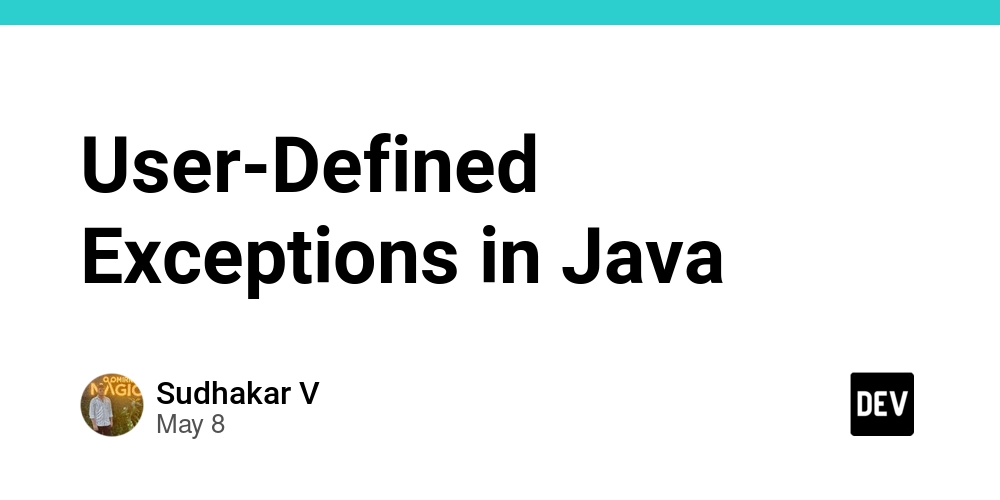
In Java, you can create your own exception classes to represent specific error conditions in your application. These are called user-defined or custom exceptions.
Why Create Custom Exceptions?
- To represent application-specific error conditions
- To provide more meaningful exception messages
- To include additional error information
- To create a hierarchy of exceptions specific to your domain
How to Create Custom Exceptions
There are two main types of exceptions you can create:
1. Checked Exceptions (extend Exception
)
These must be declared in the method signature or handled with try-catch.
2. Unchecked Exceptions (extend RuntimeException
)
These don't need to be declared or caught.
Creating a Custom Exception
Basic Structure
// For checked exception
class MyException extends Exception {
public MyException(String message) {
super(message);
}
}
// For unchecked exception
class MyRuntimeException extends RuntimeException {
public MyRuntimeException(String message) {
super(message);
}
}
Complete Example
// Custom checked exception
class InvalidAgeException extends Exception {
public InvalidAgeException(String message) {
super(message);
}
}
// Custom unchecked exception
class InsufficientFundsException extends RuntimeException {
private double balance;
private double amount;
public InsufficientFundsException(double balance, double amount) {
super("Insufficient funds: Attempted to withdraw " + amount + " but only " + balance + " available");
this.balance = balance;
this.amount = amount;
}
public double getBalance() {
return balance;
}
public double getAmount() {
return amount;
}
}
public class BankAccount {
private double balance;
public BankAccount(double balance) {
this.balance = balance;
}
public void withdraw(double amount) throws InvalidAgeException {
if (amount < 0) {
throw new InvalidAgeException("Withdrawal amount cannot be negative");
}
if (amount > balance) {
throw new InsufficientFundsException(balance, amount);
}
balance -= amount;
}
public static void main(String[] args) {
BankAccount account = new BankAccount(1000);
try {
account.withdraw(1500); // This will throw InsufficientFundsException
} catch (InvalidAgeException e) {
System.out.println("Error: " + e.getMessage());
}
try {
account.withdraw(-100); // This will throw InvalidAgeException
} catch (InvalidAgeException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
Best Practices for Custom Exceptions
-
Naming: End your exception class names with "Exception" (e.g.,
InvalidInputException
) - Constructors: Provide at least a constructor that takes a String message
-
Serialization: For serializable exceptions, declare a
serialVersionUID
:
private static final long serialVersionUID = 1L;
-
Documentation: Document when your exception should be thrown using
@throws
in Javadoc - Avoid overuse: Only create custom exceptions when standard Java exceptions don't suffice
When to Use Custom Exceptions
- When you need to pass additional information about the error
- When you want to catch specific exceptions in your application
- When you want to create a hierarchy of related exceptions
- When standard Java exceptions don't precisely describe your error condition
Remember that custom exceptions should follow the same general principles as Java's built-in exceptions - they should be used for exceptional conditions, not for normal flow control.