The Django Iceberg
Hey buddy, what framework will you go in for after studying programming? Django!!! Django has always been my go-to framework when I need to build something quickly without sacrificing quality. There's something spacial about how it balances structure with flexibility and ease. But over the years, I've come to realize that Django is like an iceberg - what you see initially is just the beginning of something much deeper and more powerful than you thought. When I first started with Django, I was immediately struck by how approachable it was. The documentation was clear, the structure made sense, and I could build something functional in a matter of hours. Little did I know that beneath this friendly surface lay layers upon layers of functionality waiting to be discovered. The Tip of the Iceberg: What Newbies See Every Django journey begins with the framework's most visible features, the ones that make it accessible to developers of all backgrounds. These initial encounters with Django create a foundation that supports everything that comes later. MVC Architecture (MTV, Really) Django's "batteries-included" philosophy starts with its Model-Template-View structure. As a newcomer, you learn to define data models, create HTML templates, and write views to handle requests. The simplicity of models.py, views.py, and urls.py feels almost magical. It's like Django is saying, "Here's everything you need to get started - no fuss, no complicated setup." ORM: The Database Abstraction Layer The Object-Relational Mapper lets you interact with databases using Python code instead of raw SQL. For those new to databases, queries like MyModel.objects.filter(name="Django") are intuitive and powerful. It abstracts away the complexity while still giving you room to grow. Admin Interface The auto-generated admin panel (admin.py) is a game-changer. With minimal code, you can manage data through a sleek UI - a feature that saves weeks of boilerplate work. It's like having a built-in control center for your application. Templates and Forms Django's templating engine and form system handle frontend logic with minimal fuss. Newbies quickly build forms with validation and render dynamic HTML. The framework guides you toward best practices without being restrictive. Built-In Authentication User registration, login, and permissions are handled via Django's django.contrib.auth. Features like @login_required decorators make security seem effortless. It's reassuring to know that authentication is handled securely out of the box. The Hidden Depths: What Senior Developers Uncover As you spend more time with Django, you begin to notice patterns and features that weren't obvious at first. These deeper aspects of the framework transform how you think about web development and what's possible. Middleware: The Silent Guardian Middleware processes requests and responses at every step. Seniors use it for tasks like rate-limiting (e.g., django-ratelimit), logging, or even modifying headers. Custom middleware unlocks granular control over HTTP flows. It's like having a series of filters that can inspect or modify traffic as it passes through your application. Custom Management Commands Beyond the basic runserver and migrate commands, developers can create their own CLI tools (manage.py) for cron jobs, data imports, or cleanup tasks. It's like having a personal admin for your project - automation becomes second nature. Deployment Complexity While newbies might deploy with gunicorn and nginx, experienced developers navigate Docker, Kubernetes, and cloud services (AWS, Heroku). They optimize static files with whitenoise or CDNs and secure settings with environment variables. Deployment transforms from a mysterious process into a well-understood, repeatable workflow. Performance Tuning Caching: Using django-cache-machine or Redis to reduce database hits. Database Optimization: Indexing, raw SQL, and connection pooling (e.g., pgbouncer). Async/Await: Leveraging Django 3.1+ async views and consumers for real-time features. Performance becomes a first-class citizen in your development process. Advanced ORM Features Queryset Chaining: Combining annotate(), aggregate(), and F() expressions for complex queries. Database Routers: Routing queries to different databases for read/write separation. Raw SQL: When the ORM can't handle it, seniors write optimized SQL without fear. The ORM evolves from a convenience tool to a powerful instrument in your toolkit. Signals and Hooks Signals like post_save or pre_delete let you trigger actions (e.g., sending emails) when models change. Custom signals add event-driven logic to apps. It's like giving your application a nervous system that responds to internal events. Testing and CI/CD Seniors write tests with pytest or Django's TestCase, mock external APIs, and automate deployments via GitHub Actions or GitLab CI. Testing transforms from a chore into an essential part of your develo
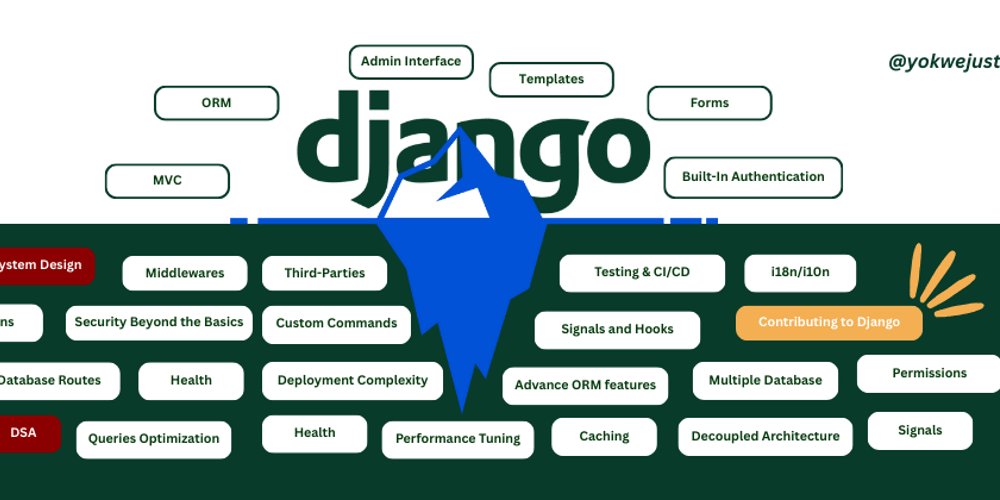
Hey buddy, what framework will you go in for after studying programming? Django!!!
Django has always been my go-to framework when I need to build something quickly without sacrificing quality. There's something spacial about how it balances structure with flexibility and ease. But over the years, I've come to realize that Django is like an iceberg - what you see initially is just the beginning of something much deeper and more powerful than you thought.
When I first started with Django, I was immediately struck by how approachable it was. The documentation was clear, the structure made sense, and I could build something functional in a matter of hours. Little did I know that beneath this friendly surface lay layers upon layers of functionality waiting to be discovered.
The Tip of the Iceberg: What Newbies See
Every Django journey begins with the framework's most visible features, the ones that make it accessible to developers of all backgrounds. These initial encounters with Django create a foundation that supports everything that comes later.
MVC Architecture (MTV, Really)
Django's "batteries-included" philosophy starts with its Model-Template-View structure. As a newcomer, you learn to define data models, create HTML templates, and write views to handle requests. The simplicity ofmodels.py
,views.py
, andurls.py
feels almost magical. It's like Django is saying, "Here's everything you need to get started - no fuss, no complicated setup."ORM: The Database Abstraction Layer
The Object-Relational Mapper lets you interact with databases using Python code instead of raw SQL. For those new to databases, queries likeMyModel.objects.filter(name="Django")
are intuitive and powerful. It abstracts away the complexity while still giving you room to grow.Admin Interface
The auto-generated admin panel (admin.py
) is a game-changer. With minimal code, you can manage data through a sleek UI - a feature that saves weeks of boilerplate work. It's like having a built-in control center for your application.Templates and Forms
Django's templating engine and form system handle frontend logic with minimal fuss. Newbies quickly build forms with validation and render dynamic HTML. The framework guides you toward best practices without being restrictive.Built-In Authentication
User registration, login, and permissions are handled via Django'sdjango.contrib.auth
. Features like@login_required
decorators make security seem effortless. It's reassuring to know that authentication is handled securely out of the box.
The Hidden Depths: What Senior Developers Uncover
As you spend more time with Django, you begin to notice patterns and features that weren't obvious at first. These deeper aspects of the framework transform how you think about web development and what's possible.
Middleware: The Silent Guardian
Middleware processes requests and responses at every step. Seniors use it for tasks like rate-limiting (e.g.,django-ratelimit
), logging, or even modifying headers. Custom middleware unlocks granular control over HTTP flows. It's like having a series of filters that can inspect or modify traffic as it passes through your application.Custom Management Commands
Beyond the basicrunserver
andmigrate
commands, developers can create their own CLI tools (manage.py
) for cron jobs, data imports, or cleanup tasks. It's like having a personal admin for your project - automation becomes second nature.Deployment Complexity
While newbies might deploy withgunicorn
andnginx
, experienced developers navigate Docker, Kubernetes, and cloud services (AWS, Heroku). They optimize static files withwhitenoise
or CDNs and secure settings with environment variables. Deployment transforms from a mysterious process into a well-understood, repeatable workflow.-
Performance Tuning
-
Caching: Using
django-cache-machine
or Redis to reduce database hits. -
Database Optimization: Indexing, raw SQL, and connection pooling (e.g.,
pgbouncer
). - Async/Await: Leveraging Django 3.1+ async views and consumers for real-time features. Performance becomes a first-class citizen in your development process.
-
Caching: Using
-
Advanced ORM Features
-
Queryset Chaining: Combining
annotate()
,aggregate()
, andF()
expressions for complex queries. - Database Routers: Routing queries to different databases for read/write separation.
- Raw SQL: When the ORM can't handle it, seniors write optimized SQL without fear. The ORM evolves from a convenience tool to a powerful instrument in your toolkit.
-
Queryset Chaining: Combining
Signals and Hooks
Signals likepost_save
orpre_delete
let you trigger actions (e.g., sending emails) when models change. Custom signals add event-driven logic to apps. It's like giving your application a nervous system that responds to internal events.Testing and CI/CD
Seniors write tests withpytest
or Django'sTestCase
, mock external APIs, and automate deployments via GitHub Actions or GitLab CI. Testing transforms from a chore into an essential part of your development workflow.Third-Party Integrations
Django's ecosystem includes packages likedjango-rest-framework
(APIs),celery
(task queues), anddjango-allauth
(social auth). Knowing when to extend core Django vs. adopting external tools becomes a nuanced decision rather than a guess.-
Security Beyond the Basics
-
CSRF/XXS Protection: Tweaking
CSP
headers orSECURE_HSTS_SECONDS
. -
Dependency Scans: Using
safety
orbandit
to audit vulnerabilities. Security becomes proactive rather than reactive.
-
CSRF/XXS Protection: Tweaking
Contributing to Django Itself
Top-tier developers dive into Django's source code, fix bugs, or propose new features. This reveals how the framework's internals - like the request-response cycle or middleware hooks - truly work. Contributing becomes a way to give back to the community that has supported your growth.
The Deepest Mysteries: Rarely Seen, Often Overlooked
These are the features that remain hidden until you've spent significant time with Django, the ones that surprise even experienced developers when they're discovered.
- Database Backends: Swapping PostgreSQL for SQLite or even custom backends.
- Geodjango: Building GIS applications with spatial data.
- Django as a Service: Using Django outside web apps (scripts, cron jobs...).
- Middleware-Driven Architecture: Designing apps where middleware handles authentication, logging, or even business logic.
Conclusion: Embracing the Iceberg
Django's beauty lies in its balance: it's approachable for beginners yet infinitely scalable for experts. The tip of the iceberg - MVC, ORM, and the admin - gets you building fast. But the deeper layers - middleware, async, and ecosystem mastery - turn good developers into architects.
So, the next time you hear "It's just Django," remember: the framework's true power lies beneath the surface, waiting for those willing to dive in.
Final Thought: The Django Iceberg isn't just about features - it's a journey. Every developer's path is unique, but the deeper you go, the more you realize how much there is to explore.