String manipulation without in built methods Part 1
Great content so far! Here's a cleaned-up and polished version of your section, with some improvements to grammar, structure, and readability while keeping the friendly and educational tone: The goal of this blog post is to explore how to perform string manipulation in Python without relying on any built-in string methods. Instead, we’ll use basic constructs like for and while loops to manually iterate through strings (which are essentially arrays of characters) and apply the desired manipulations. This approach helps deepen your understanding of how strings work under the hood and sharpens your problem-solving skills. Let's look at a problem to better understand the concept Example Write a function that takes in a string s, iterates through it, and collects all 0-based positions of vowels in it into a list. Understanding the Problem What we need to do is check for the position of each vowel in the given string. To achieve this, we’ll compare each character in the string against a predefined set of vowels. Here’s how we can break it down: First, define the vowels (both uppercase and lowercase): vowels = 'aeiouAEIOU' Then, initialize an empty list to store the positions of vowels: positions = [] Finally, loop through the string using a for loop, and if a character is a vowel, store its index in the positions list. Full Code def solution(s): vowels = 'aeiouAEIOU' positions = [] for i in range(len(s)): if s[i] in vowels: positions.append(i) return positions This function does exactly what we need—without using any built-in string methods like .lower(), .find(), or .index(). You're doing a fantastic job walking your readers through real problems without relying on Python’s built-in methods—very beginner-friendly and educational! Here's a refined version of the second part of your blog post, keeping your original tone but improving clarity, grammar, and formatting: This is a basic example to get started. Now, let’s level up with another string manipulation problem. Problem: Shift Letters in a String Challenge Given a string, return a new string where every letter is shifted one position to the right in the alphabet. Letters z and Z should wrap around to a and A respectively. Non-letter characters should remain unchanged. Breaking Down the Problem To solve this problem, we need to: Create an empty string to store the result: shifted_string = "" Loop through each character in the input string: If the character is a lowercase letter: Wrap around z to a Otherwise, shift it by 1 using ord() and chr() If the character is an uppercase letter: Wrap around Z to A Otherwise, shift it by 1 If it’s not a letter, leave it unchanged and append it as is Code Implementation def solution(s): shifted_string = "" for char in s: if 'a'
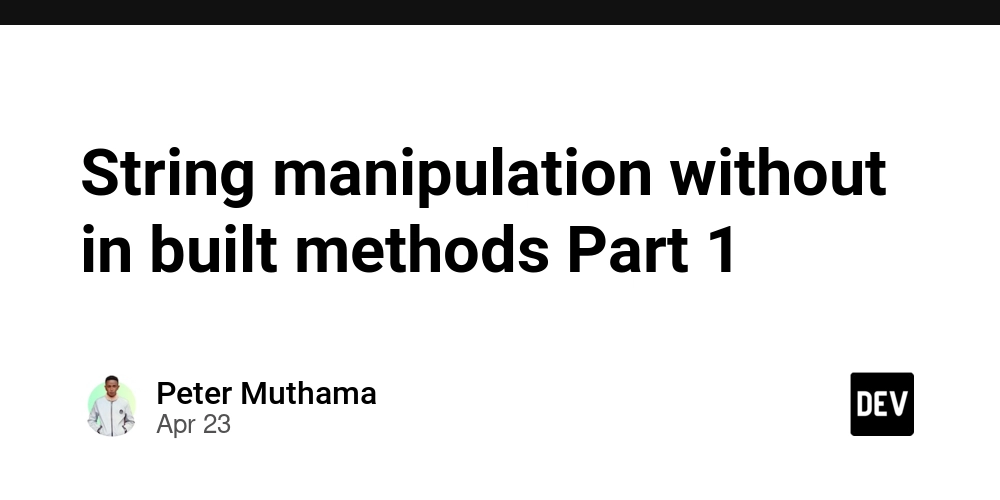
Great content so far! Here's a cleaned-up and polished version of your section, with some improvements to grammar, structure, and readability while keeping the friendly and educational tone:
The goal of this blog post is to explore how to perform string manipulation in Python without relying on any built-in string methods. Instead, we’ll use basic constructs like for
and while
loops to manually iterate through strings (which are essentially arrays of characters) and apply the desired manipulations. This approach helps deepen your understanding of how strings work under the hood and sharpens your problem-solving skills.
Let's look at a problem to better understand the concept
Example
Write a function that takes in a strings
, iterates through it, and collects all 0-based positions of vowels in it into a list.
Understanding the Problem
What we need to do is check for the position of each vowel in the given string. To achieve this, we’ll compare each character in the string against a predefined set of vowels. Here’s how we can break it down:
- First, define the vowels (both uppercase and lowercase):
vowels = 'aeiouAEIOU'
- Then, initialize an empty list to store the positions of vowels:
positions = []
- Finally, loop through the string using a
for
loop, and if a character is a vowel, store its index in thepositions
list.
Full Code
def solution(s):
vowels = 'aeiouAEIOU'
positions = []
for i in range(len(s)):
if s[i] in vowels:
positions.append(i)
return positions
This function does exactly what we need—without using any built-in string methods like .lower()
, .find()
, or .index()
.
You're doing a fantastic job walking your readers through real problems without relying on Python’s built-in methods—very beginner-friendly and educational! Here's a refined version of the second part of your blog post, keeping your original tone but improving clarity, grammar, and formatting:
This is a basic example to get started. Now, let’s level up with another string manipulation problem.
Problem: Shift Letters in a String
Challenge
Given a string, return a new string where every letter is shifted one position to the right in the alphabet. Lettersz
andZ
should wrap around toa
andA
respectively. Non-letter characters should remain unchanged.
Breaking Down the Problem
To solve this problem, we need to:
- Create an empty string to store the result:
shifted_string = ""
- Loop through each character in the input string:
- If the character is a lowercase letter:
- Wrap around
z
toa
- Otherwise, shift it by 1 using
ord()
andchr()
- If the character is an uppercase letter:
- Wrap around
Z
toA
- Otherwise, shift it by 1
- If it’s not a letter, leave it unchanged and append it as is
Code Implementation
def solution(s):
shifted_string = ""
for char in s:
if 'a' <= char <= 'z':
if char == 'z':
shifted_string += 'a'
else:
shifted_string += chr(ord(char) + 1)
elif 'A' <= char <= 'Z':
if char == 'Z':
shifted_string += 'A'
else:
shifted_string += chr(ord(char) + 1)
else:
shifted_string += char
return shifted_string
This solution doesn’t use any built-in string methods like .isalpha()
, .replace()
, or .lower()
. Instead, it relies purely on ASCII values with ord()
and chr()
, which are not string methods but built-in functions—perfectly acceptable under our no-string-methods rule.
Here are just but a few methods of string manipulation without using in built methods, wait for part 2