Stop Fetching the Same Data in Go: Cache It Instead!
If you’ve been working with Go and have ever thought: “Why am I fetching this value again? It hasn’t changed!” Then let me introduce you to one of the cleanest little gems in the standard library: sync.Once. This article is all about using sync.Once to cache values that you fetch frequently but that never really change after the first time. The Problem Sometimes, you need to fetch a value that is expensive to retrieve: A config value from your database A list of supported languages A long-lived token from an API But once you have it… you really don’t need to fetch it again. Fetching it over and over? Wasteful. Fetching it once and reusing it? Beautiful. The Go Way: sync.Once The sync.Once type ensures that a piece of code runs exactly one time, no matter how many goroutines try to call it. That’s perfect for lazy-loaded, one-time cache situations. Here’s what it looks like in practice: package main import ( "fmt" "sync" "time" ) var ( configValue string once sync.Once ) func fetchConfigFromDB() string { fmt.Println("Fetching config from DB...") time.Sleep(2 * time.Second) // Simulate delay return "my-config-value" } func GetConfig() string { once.Do(func() { configValue = fetchConfigFromDB() }) return configValue } func main() { fmt.Println("First call:", GetConfig()) fmt.Println("Second call:", GetConfig()) fmt.Println("Third call:", GetConfig()) } Output: Fetching config from DB... First call: my-config-value Second call: my-config-value Third call: my-config-value Notice how "Fetching config from DB..." only prints once? That’s the magic of sync.Once. When to Use This This approach is great when: The value doesn’t change after the first load You want lazy loading (don’t fetch at startup) Thread safety matters (it’s built-in) Examples: First-time loading of environment-based config Reading a file only once Caching an API key/token that’s valid for a long time When Not to Use It sync.Once is not for values that: Change over time Need to be refreshed Depend on request-specific data For those cases, consider a cache with expiry, or something more dynamic. Final Thoughts sync.Once is one of those tools that’s incredibly simple but solves a real problem in a clean way. If you ever find yourself writing something like: var loaded = false var mu sync.Mutex func Load() { mu.Lock() defer mu.Unlock() if !loaded { // do something once loaded = true } } …you probably just want sync.Once. If you're a software developer who enjoys exploring different technologies and techniques like this one, check out LiveAPI. It’s a super-convenient tool that lets you generate interactive API docs instantly. So, if you’re working with a codebase that lacks documentation, just use LiveAPI to generate it and save time! You can instantly try it out here!
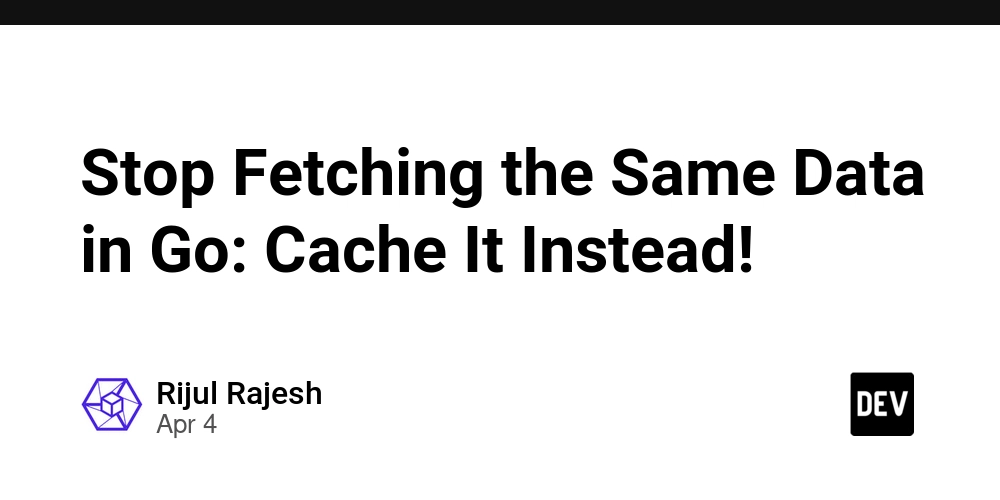
If you’ve been working with Go and have ever thought:
“Why am I fetching this value again? It hasn’t changed!”
Then let me introduce you to one of the cleanest little gems in the standard library: sync.Once
.
This article is all about using sync.Once
to cache values that you fetch frequently but that never really change after the first time.
The Problem
Sometimes, you need to fetch a value that is expensive to retrieve:
- A config value from your database
- A list of supported languages
- A long-lived token from an API
But once you have it… you really don’t need to fetch it again.
Fetching it over and over? Wasteful.
Fetching it once and reusing it? Beautiful.
The Go Way: sync.Once
The sync.Once
type ensures that a piece of code runs exactly one time, no matter how many goroutines try to call it.
That’s perfect for lazy-loaded, one-time cache situations.
Here’s what it looks like in practice:
package main
import (
"fmt"
"sync"
"time"
)
var (
configValue string
once sync.Once
)
func fetchConfigFromDB() string {
fmt.Println("Fetching config from DB...")
time.Sleep(2 * time.Second) // Simulate delay
return "my-config-value"
}
func GetConfig() string {
once.Do(func() {
configValue = fetchConfigFromDB()
})
return configValue
}
func main() {
fmt.Println("First call:", GetConfig())
fmt.Println("Second call:", GetConfig())
fmt.Println("Third call:", GetConfig())
}
Output:
Fetching config from DB...
First call: my-config-value
Second call: my-config-value
Third call: my-config-value
Notice how "Fetching config from DB..."
only prints once?
That’s the magic of sync.Once
.
When to Use This
This approach is great when:
- The value doesn’t change after the first load
- You want lazy loading (don’t fetch at startup)
- Thread safety matters (it’s built-in)
Examples:
- First-time loading of environment-based config
- Reading a file only once
- Caching an API key/token that’s valid for a long time
When Not to Use It
sync.Once
is not for values that:
- Change over time
- Need to be refreshed
- Depend on request-specific data
For those cases, consider a cache with expiry, or something more dynamic.
Final Thoughts
sync.Once
is one of those tools that’s incredibly simple but solves a real problem in a clean way. If you ever find yourself writing something like:
var loaded = false
var mu sync.Mutex
func Load() {
mu.Lock()
defer mu.Unlock()
if !loaded {
// do something once
loaded = true
}
}
…you probably just want sync.Once
.
If you're a software developer who enjoys exploring different technologies and techniques like this one, check out LiveAPI. It’s a super-convenient tool that lets you generate interactive API docs instantly.
So, if you’re working with a codebase that lacks documentation, just use LiveAPI to generate it and save time!
You can instantly try it out here!