Sentiment Analysis AI Programming
Sentiment analysis, a subfield of Natural Language Processing (NLP), focuses on identifying and extracting subjective information from text. It helps determine the emotional tone behind words, making it a valuable tool for businesses, social media monitoring, and market research. In this post, we'll explore the fundamentals of sentiment analysis programming, popular techniques, and how to build your own sentiment analysis model. What is Sentiment Analysis? Sentiment analysis involves categorizing text into positive, negative, or neutral sentiments. It leverages algorithms to interpret and classify emotions expressed in written content, such as reviews, social media posts, and feedback. Key Applications of Sentiment Analysis Brand Monitoring: Track public perception of brands through social media analysis. Customer Feedback: Analyze product reviews and customer support interactions to improve services. Market Research: Gauge consumer sentiment about products, trends, and competitors. Political Analysis: Analyze public sentiment during elections or major political events. Content Recommendation: Improve recommendation engines based on user sentiments. Popular Libraries for Sentiment Analysis NLTK (Natural Language Toolkit): A powerful Python library for text processing and sentiment analysis. TextBlob: A user-friendly library for processing textual data, including sentiment analysis. VADER: A rule-based sentiment analysis tool optimized for social media texts. Transformers (Hugging Face): Offers pre-trained models for state-of-the-art sentiment analysis. spaCy: An efficient NLP library that can be used for custom sentiment analysis tasks. Example: Sentiment Analysis with TextBlob from textblob import TextBlob Sample text text = "I love programming with Python! It's so much fun and easy to learn." Create a TextBlob object blob = TextBlob(text) Get sentiment polarity polarity = blob.sentiment.polarity if polarity > 0: print("Positive sentiment") elif polarity < 0: print("Negative sentiment") else: print("Neutral sentiment") Advanced Techniques for Sentiment Analysis Machine Learning Models: Train classifiers using algorithms like SVM, Random Forest, or neural networks. Deep Learning: Use LSTM or Transformer-based models to capture context and sentiment from large datasets. Aspect-Based Sentiment Analysis: Analyze sentiments related to specific aspects of products or services. Data Preparation for Sentiment Analysis Data Collection: Gather text data from sources like social media, reviews, or forums. Data Cleaning: Remove noise (punctuation, stop words) and normalize text (lowercasing, stemming). Labeling: Assign sentiment labels (positive, negative, neutral) for supervised learning. Challenges in Sentiment Analysis Contextual understanding can be difficult; sarcasm and irony often lead to misinterpretation. Domain-specific language or jargon may not be captured effectively by generic models. Sentiment expressed in images or videos is harder to analyze than text alone. Conclusion Sentiment analysis is a powerful tool that enables businesses and researchers to gain insights into public opinion and emotional responses. By leveraging NLP techniques and machine learning, you can build systems that understand and classify sentiments, providing value in numerous applications. Start experimenting with the tools and techniques mentioned above to unlock the potential of sentiment analysis in your projects!
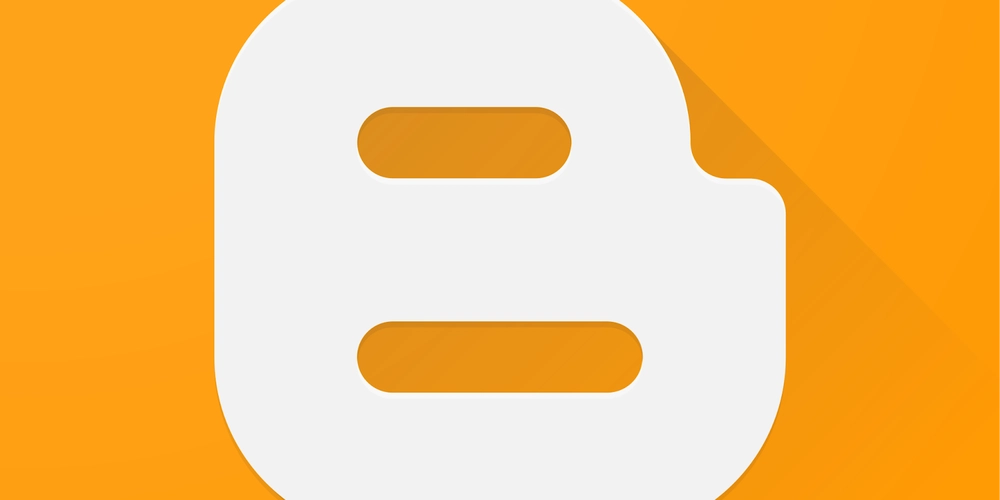
Sentiment analysis, a subfield of Natural Language Processing (NLP), focuses on identifying and extracting subjective information from text. It helps determine the emotional tone behind words, making it a valuable tool for businesses, social media monitoring, and market research. In this post, we'll explore the fundamentals of sentiment analysis programming, popular techniques, and how to build your own sentiment analysis model.
What is Sentiment Analysis?
Sentiment analysis involves categorizing text into positive, negative, or neutral sentiments. It leverages algorithms to interpret and classify emotions expressed in written content, such as reviews, social media posts, and feedback.
Key Applications of Sentiment Analysis
- Brand Monitoring: Track public perception of brands through social media analysis.
- Customer Feedback: Analyze product reviews and customer support interactions to improve services.
- Market Research: Gauge consumer sentiment about products, trends, and competitors.
- Political Analysis: Analyze public sentiment during elections or major political events.
- Content Recommendation: Improve recommendation engines based on user sentiments.
Popular Libraries for Sentiment Analysis
- NLTK (Natural Language Toolkit): A powerful Python library for text processing and sentiment analysis.
- TextBlob: A user-friendly library for processing textual data, including sentiment analysis.
- VADER: A rule-based sentiment analysis tool optimized for social media texts.
- Transformers (Hugging Face): Offers pre-trained models for state-of-the-art sentiment analysis.
- spaCy: An efficient NLP library that can be used for custom sentiment analysis tasks.
Example: Sentiment Analysis with TextBlob
from textblob import TextBlob
Sample text
text = "I love programming with Python! It's so much fun and easy to learn."
Create a TextBlob object
blob = TextBlob(text)
Get sentiment polarity
polarity = blob.sentiment.polarity
if polarity > 0:
print("Positive sentiment")
elif polarity < 0:
print("Negative sentiment")
else:
print("Neutral sentiment")
Advanced Techniques for Sentiment Analysis
- Machine Learning Models: Train classifiers using algorithms like SVM, Random Forest, or neural networks.
- Deep Learning: Use LSTM or Transformer-based models to capture context and sentiment from large datasets.
- Aspect-Based Sentiment Analysis: Analyze sentiments related to specific aspects of products or services.
Data Preparation for Sentiment Analysis
- Data Collection: Gather text data from sources like social media, reviews, or forums.
- Data Cleaning: Remove noise (punctuation, stop words) and normalize text (lowercasing, stemming).
- Labeling: Assign sentiment labels (positive, negative, neutral) for supervised learning.
Challenges in Sentiment Analysis
- Contextual understanding can be difficult; sarcasm and irony often lead to misinterpretation.
- Domain-specific language or jargon may not be captured effectively by generic models.
- Sentiment expressed in images or videos is harder to analyze than text alone.
Conclusion
Sentiment analysis is a powerful tool that enables businesses and researchers to gain insights into public opinion and emotional responses. By leveraging NLP techniques and machine learning, you can build systems that understand and classify sentiments, providing value in numerous applications. Start experimenting with the tools and techniques mentioned above to unlock the potential of sentiment analysis in your projects!