Redux Toolkit Fundamentals: Simplifying State Management
If you have ever used Redux, you probably remember how complicated it could get—setting up the store, writing actions and reducers, and making sure everything stayed immutable. It required a lot of upfront decisions, which made state management feel overwhelming. Luckily, Redux Toolkit (RTK) changes the game! It streamlines setup, enforces best practices, and comes with built-in tools like Immer for seamless immutable updates, Redux Thunk for handling async logic, and automatic store configuration. With RTK, managing state is easier, cleaner, and way less frustrating. In this article, we'll cover Redux Toolkit fundamentals—its core concepts (like configureStore and createSlice), how it works, and why it's the standard for Redux development. Whether you're new or experienced, RTK is the best approach to Redux development. Stay tuned for the next article, where we'll dive into advanced topics like multiple stores, combining reducers, shared actions across slices, and custom hooks. What is Redux, BTW? Before diving into Redux Toolkit, let’s quickly revisit Redux itself. Redux is a state management library that helps us manage global state in JavaScript applications, especially but not only in React. It uses a centralised state management pattern, ensuring structured data flow and predictable state updates. At its core, Redux is built around three main principles: Single Source of Truth: The global application state is stored in a single Redux store, making it easier to manage, debug, and track state changes throughout the app. State is Read-Only: The state cannot be modified directly. Instead, we dispatch actions to describe changes, ensuring that updates always follow a predictable and traceable flow. Changes are Made with Pure Functions: Instead of mutating state directly, reducers process actions and return a new state, ensuring predictable updates. While Redux provides simple, but powerful state management, it requires a lot of boilerplate code and manual setup—which is where Redux Toolkit comes in to simplify things. Let’s see how!
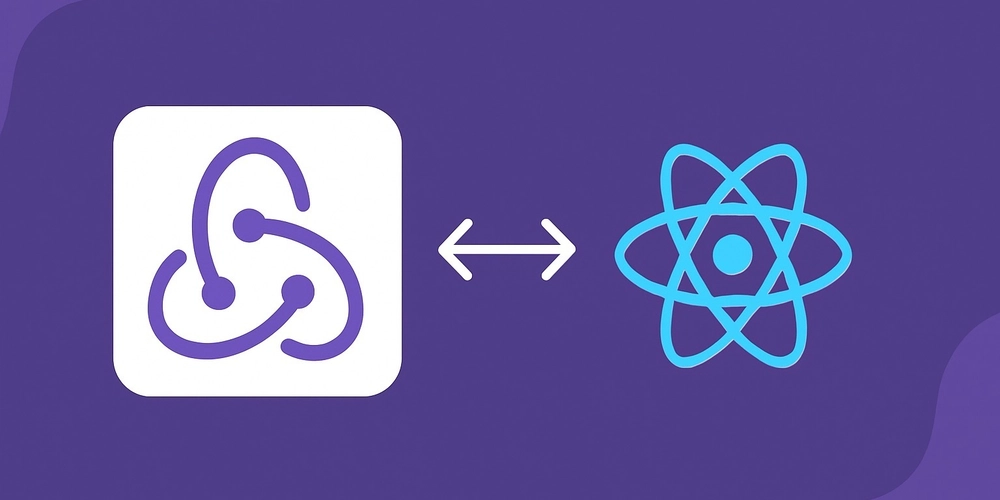
If you have ever used Redux, you probably remember how complicated it could get—setting up the store, writing actions and reducers, and making sure everything stayed immutable. It required a lot of upfront decisions, which made state management feel overwhelming. Luckily, Redux Toolkit (RTK) changes the game! It streamlines setup, enforces best practices, and comes with built-in tools like Immer for seamless immutable updates, Redux Thunk for handling async logic, and automatic store configuration. With RTK, managing state is easier, cleaner, and way less frustrating.
In this article, we'll cover Redux Toolkit fundamentals—its core concepts (like configureStore
and createSlice
), how it works, and why it's the standard for Redux development. Whether you're new or experienced, RTK is the best approach to Redux development. Stay tuned for the next article, where we'll dive into advanced topics like multiple stores, combining reducers, shared actions across slices, and custom hooks.
What is Redux, BTW?
Before diving into Redux Toolkit, let’s quickly revisit Redux itself.
Redux is a state management library that helps us manage global state in JavaScript applications, especially but not only in React. It uses a centralised state management pattern, ensuring structured data flow and predictable state updates.
At its core, Redux is built around three main principles:
- Single Source of Truth: The global application state is stored in a single Redux store, making it easier to manage, debug, and track state changes throughout the app.
- State is Read-Only: The state cannot be modified directly. Instead, we dispatch actions to describe changes, ensuring that updates always follow a predictable and traceable flow.
- Changes are Made with Pure Functions: Instead of mutating state directly, reducers process actions and return a new state, ensuring predictable updates.
While Redux provides simple, but powerful state management, it requires a lot of boilerplate code and manual setup—which is where Redux Toolkit comes in to simplify things. Let’s see how!