MY UNDERSTANDING ON PROGRAMMING SESSION DAY 2 :
Deep Dive into Operators, Language Types, and Programming History** ✅ 1. In Java: & vs && — What’s the Difference? Operator Name What it Does When to Use & Bitwise AND Compares each bit of two numbers Used in bitwise logic && Logical AND Checks if both conditions are true Used in if statements // Bitwise int a = 5; // 0101 int b = 3; // 0011 System.out.println(a & b); // 0001 → 1 // Logical if (a > 0 && b ` ✅ 3. Compare Operators in Java, Python, JS, C++ Concept Java Python JavaScript C++ Equality ==, .equals() ==, is, equals() ==, === == Strict Equality N/A N/A === N/A Increment/Decrement ++, -- ❌ Not available ++, -- ++, -- Power Math.pow ** ** (ES6) pow() ✅ 4. What is Floor Division? Floor Division (//) gives the largest integer less than or equal to the division result. 7 // 2 = 3 (not 3.5) ✅ 5. Why Is It Called "Floor" Division? Because it "rounds down" the result to the nearest lower whole number — it applies the mathematical floor function. ✅ 6. Power in Python: / vs // vs ** Operator Meaning Output Type Example / Normal Division Float 5 / 2 = 2.5 // Floor Division Integer 5 // 2 = 2 ** Power (Exponent) Integer/Float 2 ** 3 = 8 Other languages: Java: Math.pow(2, 3) JS: 2 ** 3 C++: pow(2, 3) ✅ 7. Why Python is Dynamically Typed? No need to declare type. x = 10 # x is int x = "hi" # now x is string
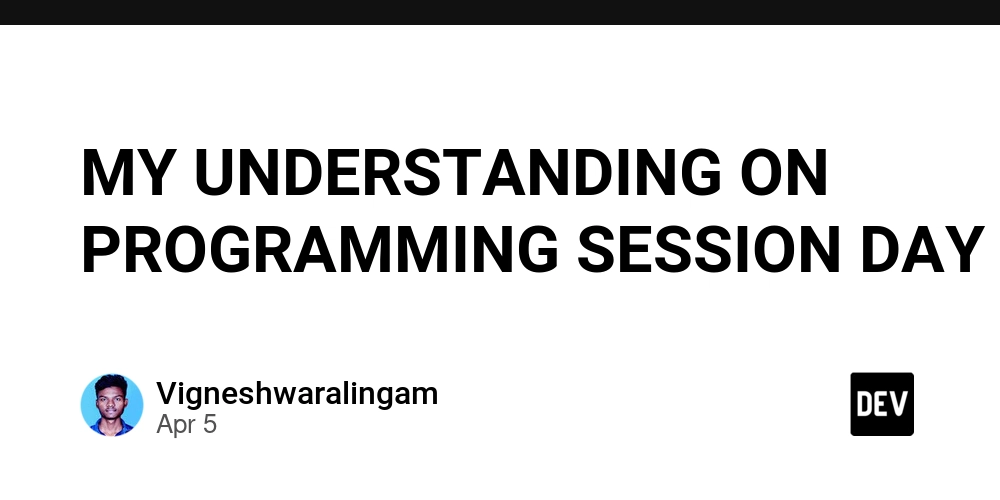
Deep Dive into Operators, Language Types, and Programming History**
✅ 1. In Java: &
vs &&
— What’s the Difference?
Operator | Name | What it Does | When to Use |
---|---|---|---|
& |
Bitwise AND | Compares each bit of two numbers | Used in bitwise logic |
&& |
Logical AND | Checks if both conditions are true | Used in if statements |
// Bitwise
int a = 5; // 0101
int b = 3; // 0011
System.out.println(a & b); // 0001 → 1
// Logical
if (a > 0 && b < 10) {
System.out.println("Valid range");
}
✅ 2. Operators in Java vs Python
Type | Java | Python |
---|---|---|
Arithmetic | + - * / % ++ -- |
+ - * / % // ** |
Logical | `&& | |
Comparison | {% raw %}== != > <
|
== != > < |
Assignment | = += -= *= |
= += -= *= |
Bitwise | `& | ^ ~ << >>` |
✅ 3. Compare Operators in Java, Python, JS, C++
Concept | Java | Python | JavaScript | C++ |
---|---|---|---|---|
Equality |
== , .equals()
|
== , is , equals()
|
== , ===
|
== |
Strict Equality | N/A | N/A | === |
N/A |
Increment/Decrement |
++ , --
|
❌ Not available |
++ , --
|
++ , --
|
Power | Math.pow |
** |
** (ES6) |
pow() |
✅ 4. What is Floor Division?
Floor Division (//
) gives the largest integer less than or equal to the division result.
7 // 2 = 3 (not 3.5)
✅ 5. Why Is It Called "Floor" Division?
Because it "rounds down" the result to the nearest lower whole number — it applies the mathematical floor function.
✅ 6. Power in Python: /
vs //
vs **
Operator | Meaning | Output Type | Example |
---|---|---|---|
/ |
Normal Division | Float | 5 / 2 = 2.5 |
// |
Floor Division | Integer | 5 // 2 = 2 |
** |
Power (Exponent) | Integer/Float | 2 ** 3 = 8 |
Other languages:
-
Java:
Math.pow(2, 3)
-
JS:
2 ** 3
-
C++:
pow(2, 3)
✅ 7. Why Python is Dynamically Typed?
- No need to declare type.
x = 10 # x is int
x = "hi" # now x is string