My Python and Raku Language Solutions to Task 1: Count Common from The Weekly Challenge 308
1. Introduction The Weekly Challenge, organized by Mohammad S. Anwar, is a friendly competition in which developers compete by solving a pair of tasks. It encourages participation from developers of all languages and levels through learning, sharing, and having fun. Task 1: Count Common from The Weekly Challenge prompts developers to count the number of common strings in two arrays. In this post I present my Python and Raku language solutions to Task 1: Count Common and finish with a brief conclusion. 2. Task 1: Count Common You are given two arrays of strings, @str1 and @str2. Write a script to return the count of common strings in both arrays. The Weekly Challenge 308, Task 1: Count Common Examples 1 - 3 present the expected outputs from given inputs. Example 1 Input: @str1 = ("perl", "weekly", "challenge") @str2 = ("raku", "weekly", "challenge") Output: 2 Output: 2 since the two strings "weekly" and "challenge" are common to @str1 and @str2. Example 2 Input: @str1 = ("perl", "raku", "python") @str2 = ("python", "java") Output: 1 Example 3 Input: @str1 = ("guest", "contribution") @str2 = ("fun", "weekly", "challenge") Output: 0 3. My solutions to Task 1: Count Common Set operations simplify the process of finding and counting common elements between sets. Both Python and Raku have functionality for operating on sets. Python uses the set data type and Raku uses the Set class. Both languages also have methods for converting other data types into sets. My solutions use set operations and methods to count the strings common between the two arrays. Both solutions follow the same approach: Convert @str1 and @str2 to sets. Find the intersection of the two sets. Return the number of elements in the intersection. Python def count_common(str1: [str], str2: [str]) -> int: return len(set(str1).intersection(set(str2))) set(str1) and set(str2) convert str1 and str2 to set data types. set(str1).intersection(set(str2)) returns the set of common elements of set(str1) and set(str2). return len(set(str1).intersection(set(str2))) returns the number of elements in the set of common elements. Raku sub count_common (@str1, @str2) { my $set1 = set @str1; my $set2 = set @str2; return ($set1 (&) $set2).elems; } my $set1 = set @str1 and my $set2 = set @str2 convert @str1 and @str2 to set objects. ($set1 (&) $set2) returns the set of intersecting elements of $set1 and $set2. return ($set1 (&) $set2).elems returns the number elements in the set of intersecting elements. 4. Conclusion In this post I presented my Python and Raku language solutions to Task 1 - Count Common from The Weekly Challenge 308. For more information on participating in The Weekly Challenge, please visit theweeklychallenge.org. Learn more about Raku at https://raku.org. Learn more about Python at https://python.org.
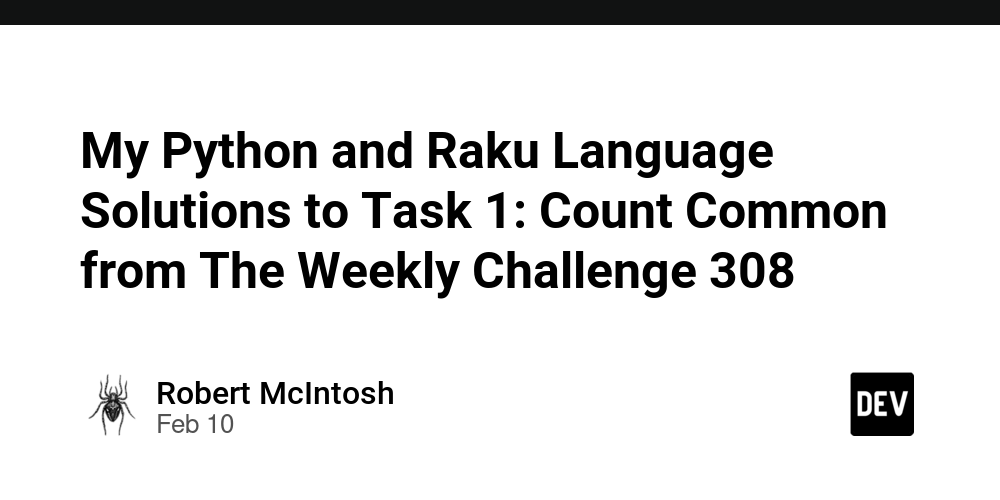
1. Introduction
The Weekly Challenge, organized by Mohammad S. Anwar, is a friendly competition in which developers compete by solving a pair of tasks. It encourages participation from developers of all languages and levels through learning, sharing, and having fun.
Task 1: Count Common from The Weekly Challenge prompts developers to count the number of common strings in two arrays.
In this post I present my Python and Raku language solutions to Task 1: Count Common and finish with a brief conclusion.
2. Task 1: Count Common
You are given two arrays of strings,
@str1
and@str2
.Write a script to return the count of common strings in both arrays.
Examples 1 - 3 present the expected outputs from given inputs.
Example 1
Input: @str1 = ("perl", "weekly", "challenge")
@str2 = ("raku", "weekly", "challenge")
Output: 2
Output: 2
since the two strings "weekly"
and "challenge"
are common to @str1
and @str2
.
Example 2
Input: @str1 = ("perl", "raku", "python")
@str2 = ("python", "java")
Output: 1
Example 3
Input: @str1 = ("guest", "contribution")
@str2 = ("fun", "weekly", "challenge")
Output: 0
3. My solutions to Task 1: Count Common
Set operations simplify the process of finding and counting common elements between sets. Both Python and Raku have functionality for operating on sets. Python uses the set
data type and Raku uses the Set
class. Both languages also have methods for converting other data types into sets.
My solutions use set operations and methods to count the strings common between the two arrays. Both solutions follow the same approach:
- Convert
@str1
and@str2
to sets. - Find the intersection of the two sets.
- Return the number of elements in the intersection.
Python
def count_common(str1: [str], str2: [str]) -> int:
return len(set(str1).intersection(set(str2)))
-
set(str1)
andset(str2)
convertstr1
andstr2
toset
data types. -
set(str1).intersection(set(str2))
returns the set of common elements ofset(str1)
andset(str2)
. -
return len(set(str1).intersection(set(str2)))
returns the number of elements in the set of common elements.
Raku
sub count_common (@str1, @str2) {
my $set1 = set @str1;
my $set2 = set @str2;
return ($set1 (&) $set2).elems;
}
-
my $set1 = set @str1
andmy $set2 = set @str2
convert@str1
and@str2
toset
objects. -
($set1 (&) $set2)
returns theset
of intersecting elements of$set1
and$set2
. -
return ($set1 (&) $set2).elems
returns the number elements in the set of intersecting elements.
4. Conclusion
In this post I presented my Python and Raku language solutions to Task 1 - Count Common from The Weekly Challenge 308.
For more information on participating in The Weekly Challenge, please visit theweeklychallenge.org.
Learn more about Raku at https://raku.org.
Learn more about Python at https://python.org.