Is Your Java Leaking? GC to the Rescue!
Memory management is fundamental to software development. In languages like C/C++, developers must manually allocate and deallocate memory, leading to potential errors like memory leaks. Java simplifies this with automatic memory management through its Garbage Collector (GC). The GC automatically reclaims memory occupied by objects no longer in use. This article will demystify the GC, explaining its core concepts and practical implications. I. What is Garbage Collection? (Core Concepts) In Java, 'garbage' refers to objects that are no longer reachable by the application. These objects reside in the 'heap,' a dynamic memory area managed by the GC. The GC's primary function is to reclaim the memory occupied by these unreachable objects. The core process involves: Marking: The GC identifies live objects by tracing references from a set of root objects (e.g., static variables, local variables). Reachable objects are marked. Sweeping/Deleting: The GC scans the heap and reclaims the memory of unmarked (unreachable) objects. Compacting (Optional): Some GC algorithms compact the heap by moving live objects to contiguous memory locations, reducing fragmentation. II. Generational Garbage Collection (Practical Application) "Java's GC often employs generational garbage collection, dividing the heap into: Young Generation: This is where newly created objects are allocated. It's designed for frequent minor GC cycles, as most objects are short-lived. Eden Space: New objects are initially allocated here. Survivor Spaces (S0 and S1): Objects that survive minor GCs are moved to survivor spaces. Minor GC Cycles: These cycles reclaim memory in the young generation. Old Generation (Tenured Generation): Objects that survive multiple minor GCs are promoted to the old generation. Major GC Cycles: These cycles reclaim memory in the old generation and are less frequent but more time-consuming. Objects are promoted from the young generation to the old generation based on their survival count." III. "Stop-the-World" Events (Real-World Implications) During a GC cycle, particularly a major GC, the Java Virtual Machine (JVM) may pause application threads. These pauses are known as 'stop-the-world' (STW) events. STW events are necessary to ensure a consistent view of the heap for accurate garbage collection. However, they can impact application performance by introducing latency. Strategies to minimize STW events include: Selecting appropriate GC algorithms (e.g., G1, ZGC). Optimizing heap size and GC parameters. Writing code that minimizes object allocation. IV. Practical Tips and Tuning (Actionable Advice) To optimize GC performance: Use JVM flags like -Xms, -Xmx, and -XX:+UseG1GC to configure heap size and GC algorithms. Minimize object creation and promote object reuse. Avoid finalizers, as they can impact GC performance. Utilize tools like JConslole and GC logs (-Xlog:gc*) to monitor GC activity. When an object is no longer needed, set the object reference to null. Consider different garbage collectors when needed. V. Conclusion (Recap & Takeaway) Java's GC automates memory management, enhancing developer productivity and preventing memory leaks. Understanding its core concepts and tuning strategies is essential for building efficient Java applications. By optimizing GC performance, you can minimize latency and ensure a smooth user experience. Continue to explore GC algorithms and best practices to further enhance your Java development skills. Leave a comment if you have a question or learnt something today
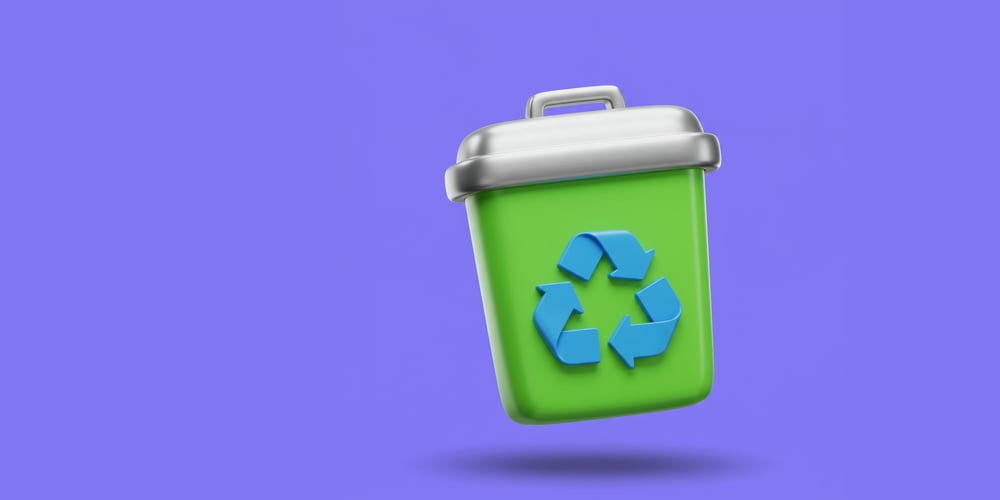
Memory management is fundamental to software development. In languages like C/C++, developers must manually allocate and deallocate memory, leading to potential errors like memory leaks. Java simplifies this with automatic memory management through its Garbage Collector (GC). The GC automatically reclaims memory occupied by objects no longer in use. This article will demystify the GC, explaining its core concepts and practical implications.
I. What is Garbage Collection? (Core Concepts)
In Java, 'garbage' refers to objects that are no longer reachable by the application. These objects reside in the 'heap,' a dynamic memory area managed by the GC. The GC's primary function is to reclaim the memory occupied by these unreachable objects.
The core process involves:
- Marking: The GC identifies live objects by tracing references from a set of root objects (e.g., static variables, local variables). Reachable objects are marked.
- Sweeping/Deleting: The GC scans the heap and reclaims the memory of unmarked (unreachable) objects.
- Compacting (Optional): Some GC algorithms compact the heap by moving live objects to contiguous memory locations, reducing fragmentation.
II. Generational Garbage Collection (Practical Application)
"Java's GC often employs generational garbage collection, dividing the heap into:
-
Young Generation: This is where newly created objects are allocated. It's designed for frequent minor GC cycles, as most objects are short-lived.
- Eden Space: New objects are initially allocated here.
- Survivor Spaces (S0 and S1): Objects that survive minor GCs are moved to survivor spaces.
- Minor GC Cycles: These cycles reclaim memory in the young generation.
-
Old Generation (Tenured Generation): Objects that survive multiple minor GCs are promoted to the old generation.
- Major GC Cycles: These cycles reclaim memory in the old generation and are less frequent but more time-consuming.
Objects are promoted from the young generation to the old generation based on their survival count."
III. "Stop-the-World" Events (Real-World Implications)
During a GC cycle, particularly a major GC, the Java Virtual Machine (JVM) may pause application threads. These pauses are known as 'stop-the-world' (STW) events.
STW events are necessary to ensure a consistent view of the heap for accurate garbage collection. However, they can impact application performance by introducing latency.
Strategies to minimize STW events include:
- Selecting appropriate GC algorithms (e.g., G1, ZGC).
- Optimizing heap size and GC parameters.
- Writing code that minimizes object allocation.
IV. Practical Tips and Tuning (Actionable Advice)
To optimize GC performance:
- Use JVM flags like
-Xms
,-Xmx
, and-XX:+UseG1GC
to configure heap size and GC algorithms. - Minimize object creation and promote object reuse.
- Avoid finalizers, as they can impact GC performance.
- Utilize tools like JConslole and GC logs (
-Xlog:gc*
) to monitor GC activity. - When an object is no longer needed, set the object reference to null.
- Consider different garbage collectors when needed.
V. Conclusion (Recap & Takeaway)
Java's GC automates memory management, enhancing developer productivity and preventing memory leaks. Understanding its core concepts and tuning strategies is essential for building efficient Java applications. By optimizing GC performance, you can minimize latency and ensure a smooth user experience. Continue to explore GC algorithms and best practices to further enhance your Java development skills.
Leave a comment if you have a question or learnt something today