Graph Theory and Implementation in Java
Java Graph Implementation Introduction Graph Problems in Java is the theory of a fundamental branch of computer science and mathematics that focuses on the study of graphs. A graph consists of nodes (also called vertices) and edges (connections between nodes). It is widely used in various domains, including computer networks, social media analysis, pathfinding in maps, and recommendation systems. In this Java tutorial, we will explore the basics of graph theory and discuss the key aspects of Java graph implementation. Understanding how graphs work and how to implement them in Java is essential for solving complex computational problems efficiently. Importance of Graphs in Computing Graphs are used extensively in software development and real-world applications. They help in solving problems such as: Navigation Systems: Used in GPS applications for shortest path finding. Social Networks: Representing connections between users. Web Crawlers: Indexing and ranking web pages. Dependency Management: Used in compilers and task scheduling. Biological Research: Gene sequencing and protein interaction networks. The ability to implement and manipulate graphs efficiently in Java tutorial is a valuable tutorial for software engineers and data scientists. Types of Graphs Before diving into Java graph implementation, it is important to understand the different types of graphs: Directed vs. Undirected Graphs Directed Graph (Digraph): Edges have a direction, meaning connections go one way. Undirected Graph: Edges do not have a direction, allowing connections in both directions. Weighted vs. Unweighted Graphs Weighted Graph: Each edge has a weight or cost associated with it (e.g., road distances in maps). Unweighted Graph: All edges have equal weight. Cyclic vs. Acyclic Graphs Cyclic Graph: Contains at least one cycle. Acyclic Graph: Does not contain any cycles (e.g., trees are a special case of acyclic graphs). Sparse vs. Dense Graphs Sparse Graph: Has fewer edges relative to the number of vertices. Dense Graph: Contains many edges compared to the number of vertices. Java Graph Implementation Overview To implement a graph in Java, we need a way to store vertices and edges. There are several approaches to representing graphs in Java: 1. Adjacency Matrix Representation This method uses a two-dimensional array where rows and columns represent vertices, and the presence of an edge is marked in the matrix. This approach is simple but consumes more memory for large graphs. 2. Adjacency List Representation In this method, each vertex maintains a list of adjacent vertices. This is more memory-efficient and is widely used in Java graph implementation. 3. Edge List Representation Here, a list of edges is maintained, each defining a connection between two vertices. It is useful for graph traversal algorithms. Key Graph Operations When implementing a graph in Java, several key operations need to be considered: Adding and Removing Vertices: Dynamically managing the nodes in a graph. Adding and Removing Edges: Establishing and deleting connections between vertices. Graph Traversal: Methods like Breadth-First Search (BFS) and Depth-First Search (DFS) to explore the graph. Shortest Path Algorithms: Techniques like Dijkstra’s Algorithm and Bellman-Ford Algorithm to find the shortest path between nodes. Cycle Detection: Identifying cycles in directed and undirected graphs. Connected Components: Finding distinct groups of connected nodes within a graph. Applications of Java Graph Implementation Implementing graphs in Java enables the development of efficient solutions for real-world problems. Some practical applications include: Network Routing: Optimizing data transmission in computer networks. Task Scheduling: Managing dependencies in parallel computing. Artificial Intelligence: Graph-based search algorithms for decision-making. Blockchain Technology: Managing transaction history in distributed ledgers. Challenges in Graph Implementation While graphs are powerful, their implementation comes with challenges: Memory Consumption: Large graphs can be memory-intensive. Computational Complexity: Some graph algorithms have high time complexity. Data Structure Selection: Choosing the right representation (adjacency list vs. adjacency matrix) affects performance. Conclusion Graph troubles in Java theory plays a crucial role in computer science, and mastering Java graph implementation is essential for solving complex problems. This Java tutorial covered the importance of graphs, different types, key operations, and real-world applications. Understanding these concepts will help developers create efficient and scalable solutions using graphs in Java. For further learning, explore advanced Java graph algorithms like Kruskal’s and Prim’s alg
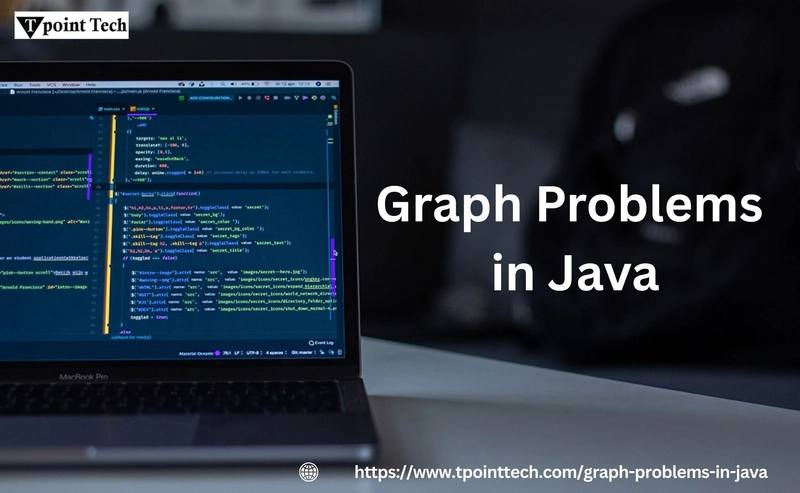
Java Graph Implementation
Introduction
Graph Problems in Java is the theory of a fundamental branch of computer science and mathematics that focuses on the study of graphs. A graph consists of nodes (also called vertices) and edges (connections between nodes). It is widely used in various domains, including computer networks, social media analysis, pathfinding in maps, and recommendation systems.
In this Java tutorial, we will explore the basics of graph theory and discuss the key aspects of Java graph implementation. Understanding how graphs work and how to implement them in Java is essential for solving complex computational problems efficiently.
Importance of Graphs in Computing
Graphs are used extensively in software development and real-world applications. They help in solving problems such as:
- Navigation Systems: Used in GPS applications for shortest path finding.
- Social Networks: Representing connections between users.
- Web Crawlers: Indexing and ranking web pages.
- Dependency Management: Used in compilers and task scheduling.
- Biological Research: Gene sequencing and protein interaction networks.
The ability to implement and manipulate graphs efficiently in Java tutorial is a valuable tutorial for software engineers and data scientists.
Types of Graphs
Before diving into Java graph implementation, it is important to understand the different types of graphs:
-
Directed vs. Undirected Graphs
- Directed Graph (Digraph): Edges have a direction, meaning connections go one way.
- Undirected Graph: Edges do not have a direction, allowing connections in both directions.
-
Weighted vs. Unweighted Graphs
- Weighted Graph: Each edge has a weight or cost associated with it (e.g., road distances in maps).
- Unweighted Graph: All edges have equal weight.
-
Cyclic vs. Acyclic Graphs
- Cyclic Graph: Contains at least one cycle.
- Acyclic Graph: Does not contain any cycles (e.g., trees are a special case of acyclic graphs).
-
Sparse vs. Dense Graphs
- Sparse Graph: Has fewer edges relative to the number of vertices.
- Dense Graph: Contains many edges compared to the number of vertices.
Java Graph Implementation Overview
To implement a graph in Java, we need a way to store vertices and edges. There are several approaches to representing graphs in Java:
1. Adjacency Matrix Representation
This method uses a two-dimensional array where rows and columns represent vertices, and the presence of an edge is marked in the matrix. This approach is simple but consumes more memory for large graphs.
2. Adjacency List Representation
In this method, each vertex maintains a list of adjacent vertices. This is more memory-efficient and is widely used in Java graph implementation.
3. Edge List Representation
Here, a list of edges is maintained, each defining a connection between two vertices. It is useful for graph traversal algorithms.
Key Graph Operations
When implementing a graph in Java, several key operations need to be considered:
- Adding and Removing Vertices: Dynamically managing the nodes in a graph.
- Adding and Removing Edges: Establishing and deleting connections between vertices.
- Graph Traversal: Methods like Breadth-First Search (BFS) and Depth-First Search (DFS) to explore the graph.
- Shortest Path Algorithms: Techniques like Dijkstra’s Algorithm and Bellman-Ford Algorithm to find the shortest path between nodes.
- Cycle Detection: Identifying cycles in directed and undirected graphs.
- Connected Components: Finding distinct groups of connected nodes within a graph.
Applications of Java Graph Implementation
Implementing graphs in Java enables the development of efficient solutions for real-world problems. Some practical applications include:
- Network Routing: Optimizing data transmission in computer networks.
- Task Scheduling: Managing dependencies in parallel computing.
- Artificial Intelligence: Graph-based search algorithms for decision-making.
- Blockchain Technology: Managing transaction history in distributed ledgers.
Challenges in Graph Implementation
While graphs are powerful, their implementation comes with challenges:
- Memory Consumption: Large graphs can be memory-intensive.
- Computational Complexity: Some graph algorithms have high time complexity.
- Data Structure Selection: Choosing the right representation (adjacency list vs. adjacency matrix) affects performance.
Conclusion
Graph troubles in Java theory plays a crucial role in computer science, and mastering Java graph implementation is essential for solving complex problems. This Java tutorial covered the importance of graphs, different types, key operations, and real-world applications. Understanding these concepts will help developers create efficient and scalable solutions using graphs in Java.
For further learning, explore advanced Java graph algorithms like Kruskal’s and Prim’s algorithms for minimum spanning trees, and Floyd-Warshall’s algorithm for all-pairs shortest paths.