Functional Reactive Programming in JavaScript
Functional Reactive Programming in JavaScript: A Comprehensive Guide Introduction Functional Reactive Programming (FRP) is a powerful paradigm that merges functional programming principles with reactive programming, enabling the developer to manage asynchronous data streams and the relationships between these streams efficiently. This article delves into the nuanced aspects of FRP in JavaScript, offering a detailed historical context, complex code examples, performance considerations, and advanced debugging techniques. By the end, senior developers will have robust knowledge and practical skills to leverage FRP in real-world applications. Historical Context Proponents of functional programming like John Hughes have influenced this paradigm since the early 1980s. However, reactive programming gained traction with the advent of GUI frameworks, where asynchronous event handling became paramount. The term "reactive programming" was popularized in the 1990s with the introduction of languages like Elm and Rx (Reactive Extensions). In JavaScript, libraries such as RxJS (Reactive Extensions for JavaScript) define FRP by providing the means to create data streams, allowing developers to respond to changes in data as they occur rather than polling for updates. Key Principles of FRP First-Class Functions: Functions in FRP are treated as first-class citizens, which allows them to be passed as arguments, returned from other functions, and assigned to variables. Immutability: FRP emphasizes immutable data structures, allowing functions to avoid side effects and simplifying reasoning about code. Event Streams: FRP revolves around the concept of observable streams. These represent sequences of values over time, enabling the program to react to these values. Declarative Style: Employing a declarative approach allows developers to describe what they want to do, rather than how to do it. Composability: Functions and streams can be combined, mapped, filtered, and composed to create complex interactions in a manageable way. Technical Implementation Setting Up with RxJS We will utilize RxJS as it provides an extensive set of operators and powerful abstractions for handling observables. npm install rxjs Basic Example: Observables and Operators Let’s explore a simple example of creating an observable that emits values over time, then applying operators to manipulate these values. import { interval } from 'rxjs'; import { map, filter } from 'rxjs/operators'; // Create an observable that emits every second const source$ = interval(1000); // Transform the emitted value and filter const example$ = source$.pipe( map(value => value * 2), // Multiply emitted value by 2 filter(value => value % 3 === 0) // Filter to keep only multiples of 3 ); // Subscribe to the stream example$.subscribe(val => console.log(val)); In this scenario, every second, the observable emits a value starting from 0. The map operator multiplies the emitted number by 2, and the filter operator only allows values that are multiples of 3 to pass through. Complex Use Case: Combining Multiple Streams FRP shines when combining multiple event streams. Consider a scenario where we manage user input from a search box and a button click, combining both to fetch data. import { fromEvent, merge } from 'rxjs'; import { debounceTime, map, switchMap } from 'rxjs/operators'; import { ajax } from 'rxjs/ajax'; // Assume there’s an input field for searching and a button for submitting const inputElement = document.getElementById('search-input'); const buttonElement = document.getElementById('search-button'); // Observable for input events with debouncing const input$ = fromEvent(inputElement, 'input').pipe( debounceTime(300), map(event => event.target.value) ); // Observable for button clicks const buttonClick$ = fromEvent(buttonElement, 'click').pipe( map(() => inputElement.value) ); // Merge both observables const searchStream$ = merge(input$, buttonClick$).pipe( filter(term => term.length > 0), // Ignore empty searches switchMap(term => ajax.getJSON(`https://api.example.com/search?query=${term}`) ) ); // Subscribe to search results searchStream$.subscribe(results => { console.log('Search results:', results); }); In this example, we effectively merge the input changes and a button click event to trigger a search only when the user types or clicks. The debounceTime operator helps in reducing the number of API calls by limiting how often the function executes. Advanced Implementation Techniques Error Handling with Observables Error handling in reactive programming is crucial. Using RxJS, we can catch and react to errors seamlessly. import { of } from 'rxjs'; import { catchError } from 'rxjs/operators'; const safeSearchStream$ = searchStream$.pipe( catchError(error => { cons
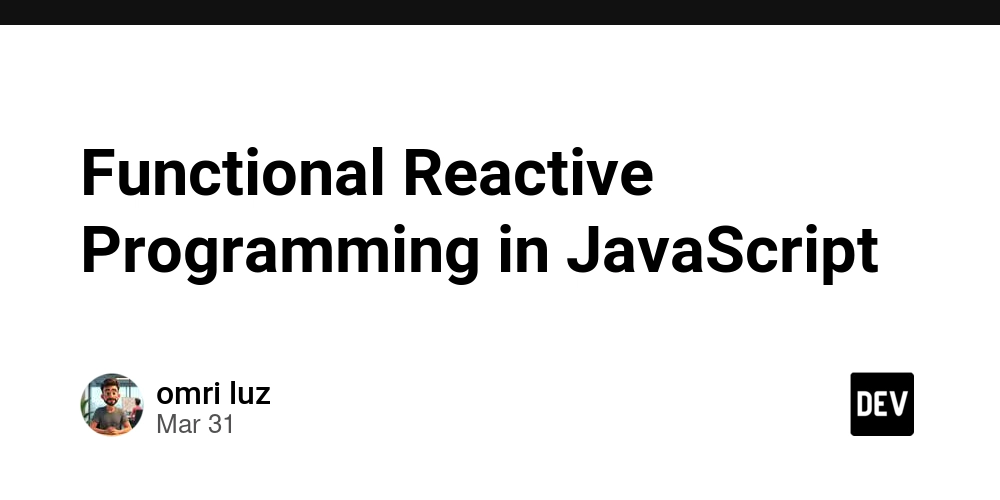
Functional Reactive Programming in JavaScript: A Comprehensive Guide
Introduction
Functional Reactive Programming (FRP) is a powerful paradigm that merges functional programming principles with reactive programming, enabling the developer to manage asynchronous data streams and the relationships between these streams efficiently. This article delves into the nuanced aspects of FRP in JavaScript, offering a detailed historical context, complex code examples, performance considerations, and advanced debugging techniques. By the end, senior developers will have robust knowledge and practical skills to leverage FRP in real-world applications.
Historical Context
Proponents of functional programming like John Hughes have influenced this paradigm since the early 1980s. However, reactive programming gained traction with the advent of GUI frameworks, where asynchronous event handling became paramount. The term "reactive programming" was popularized in the 1990s with the introduction of languages like Elm and Rx (Reactive Extensions).
In JavaScript, libraries such as RxJS (Reactive Extensions for JavaScript) define FRP by providing the means to create data streams, allowing developers to respond to changes in data as they occur rather than polling for updates.
Key Principles of FRP
First-Class Functions: Functions in FRP are treated as first-class citizens, which allows them to be passed as arguments, returned from other functions, and assigned to variables.
Immutability: FRP emphasizes immutable data structures, allowing functions to avoid side effects and simplifying reasoning about code.
Event Streams: FRP revolves around the concept of observable streams. These represent sequences of values over time, enabling the program to react to these values.
Declarative Style: Employing a declarative approach allows developers to describe what they want to do, rather than how to do it.
Composability: Functions and streams can be combined, mapped, filtered, and composed to create complex interactions in a manageable way.
Technical Implementation
Setting Up with RxJS
We will utilize RxJS as it provides an extensive set of operators and powerful abstractions for handling observables.
npm install rxjs
Basic Example: Observables and Operators
Let’s explore a simple example of creating an observable that emits values over time, then applying operators to manipulate these values.
import { interval } from 'rxjs';
import { map, filter } from 'rxjs/operators';
// Create an observable that emits every second
const source$ = interval(1000);
// Transform the emitted value and filter
const example$ = source$.pipe(
map(value => value * 2), // Multiply emitted value by 2
filter(value => value % 3 === 0) // Filter to keep only multiples of 3
);
// Subscribe to the stream
example$.subscribe(val => console.log(val));
In this scenario, every second, the observable emits a value starting from 0. The map
operator multiplies the emitted number by 2, and the filter
operator only allows values that are multiples of 3 to pass through.
Complex Use Case: Combining Multiple Streams
FRP shines when combining multiple event streams. Consider a scenario where we manage user input from a search box and a button click, combining both to fetch data.
import { fromEvent, merge } from 'rxjs';
import { debounceTime, map, switchMap } from 'rxjs/operators';
import { ajax } from 'rxjs/ajax';
// Assume there’s an input field for searching and a button for submitting
const inputElement = document.getElementById('search-input');
const buttonElement = document.getElementById('search-button');
// Observable for input events with debouncing
const input$ = fromEvent(inputElement, 'input').pipe(
debounceTime(300),
map(event => event.target.value)
);
// Observable for button clicks
const buttonClick$ = fromEvent(buttonElement, 'click').pipe(
map(() => inputElement.value)
);
// Merge both observables
const searchStream$ = merge(input$, buttonClick$).pipe(
filter(term => term.length > 0), // Ignore empty searches
switchMap(term =>
ajax.getJSON(`https://api.example.com/search?query=${term}`)
)
);
// Subscribe to search results
searchStream$.subscribe(results => {
console.log('Search results:', results);
});
In this example, we effectively merge the input changes and a button click event to trigger a search only when the user types or clicks. The debounceTime
operator helps in reducing the number of API calls by limiting how often the function executes.
Advanced Implementation Techniques
Error Handling with Observables
Error handling in reactive programming is crucial. Using RxJS, we can catch and react to errors seamlessly.
import { of } from 'rxjs';
import { catchError } from 'rxjs/operators';
const safeSearchStream$ = searchStream$.pipe(
catchError(error => {
console.error('Error occurred:', error);
return of([]); // Return an empty array on error
})
);
This ensures the application remains stable, even when an error occurs during an API call.
Managing Side Effects
Known as the "side effects" in functional programming, managing these effects is vital. Libraries like Redux Saga bring a declarative pattern for handling side effects in a more structured way. However, RxJS can also help manage side effects directly within observable chains using the tap
operator.
import { tap } from 'rxjs/operators';
const enhancedSearchStream$ = searchStream$.pipe(
tap(results => {
// Side effect: Update UI or store state
console.log('Update UI with new results:', results);
})
);
Comparing Approaches: FRP vs. Traditional Event Handlers
When contrasting FRP with traditional event handlers, we see significant advantages:
Scalability: FRP scales more gracefully with complexity. As the number of events increases, managing state transitions with flat observable chains is more manageable than using callback hell.
Testability: Observables can be easily mocked and tested due to their declarative nature.
Composability: Stream manipulation via operators fosters a clean and readable style.
Real-World Use Cases
Web Applications: FRP is especially useful in complex user interfaces, such as those found in Angular or React applications, where interactivity and asynchronicity abound.
Data Visualization Dashboards: Handling multiple data streams and reactive updates is a perfect fit for FRP, particularly in real-time applications like stock market or health monitoring systems.
Game Development: FRP facilitates managing user inputs and game state in a reactive manner, simplifying the development of complex interaction patterns.
Performance Considerations
While powerful, FRP entails performance considerations:
- Memory Leaks: Always unsubscribe from observables to prevent memory leaks, especially in environments like single-page applications.
const subscription = example$.subscribe();
// Unsubscribe when component is unmounted
subscription.unsubscribe();
Heavy Computation: Offload heavy computations outside the stream if possible or use the
observeOn
operator to schedule tasks efficiently.Optimizing Subscriptions: Use operators like
shareReplay
to prevent multiple subscriptions from invoking the source observable.
import { shareReplay } from 'rxjs/operators';
const sharedExample$ = example$.pipe(shareReplay(1));
Common Pitfalls
Overusing Operators: Developers may be tempted to chain numerous operators, leading to performance degradation. Assess the necessity of each operator to maintain clarity.
Mismanaging Subscriptions: Neglecting to unsubscribe from observables creates memory leaks, particularly when returning to views that create new subscriptions.
Not Handling Errors Gracefully: Failure to implement error handling can cause entire application failures.
Debugging Techniques
Using
tap
for Inspection: Utilize thetap
operator to print intermediate values for debugging purposes.Leverage RxJS DevTools: Use visualization tools designed for RxJS to inspect the streams at runtime.
Custom Operators: Create and log custom operators for complex transformations to trace data flow.
Conclusion
Functional Reactive Programming in JavaScript opens a wide array of possibilities for building robust, scalable applications that are easy to reason about and maintain. By mastering observables, operators, and error management while understanding the trade-offs of this paradigm, senior developers can harness the full potential of FRP.
References
- RxJS Official Documentation
- Functional Reactive Programming
- JavaScript: The Good Parts
- Understanding Observables in RxJS
Through this deep dive, we hope to empower a new wave of developers to embrace FRP in their JavaScript applications, equipped with the knowledge and skills necessary to face complex challenges head-on.