Event-Driven Architecture in NestJS
Event-Driven Architecture (EDA) is an increasingly popular approach in modern systems that require high scalability, low coupling, and asynchronous processing. We'll explore how to implement this architecture using NestJS. What is Event-Driven Architecture? In an event-driven architecture: Events represent a change in state or a significant action in the system. Producers generate events. Consumers subscribe to specific events and execute actions when those events occur. An event bus acts as an intermediary, enabling communication between producers and consumers without direct coupling. This architecture enhances scalability and allows components to evolve independently. Benefits of EDA Decoupling: Services do not depend directly on each other. Scalability: Each component can be scaled independently. Reactivity: Systems react to events in real-time. Fault Tolerance: A failure in a consumer does not affect the producer. Implementing EDA in NestJS NestJS supports event emission and listening through the @nestjs/event-emitter package. Installation npm install @nestjs/event-emitter Module Configuration // app.module.ts import { Module } from '@nestjs/common'; import { EventEmitterModule } from '@nestjs/event-emitter'; @Module({ imports: [EventEmitterModule.forRoot()], }) export class AppModule {} Creating an Event // events/order-created.event.ts export class OrderCreatedEvent { constructor( public readonly orderId: string, public readonly userId: string, public readonly amount: number, ) {} } Emitting the Event // orders.service.ts import { Injectable } from '@nestjs/common'; import { EventEmitter2 } from '@nestjs/event-emitter'; import { OrderCreatedEvent } from './events/order-created.event'; @Injectable() export class OrdersService { constructor(private eventEmitter: EventEmitter2) {} async createOrder(userId: string, amount: number) { const orderId = 'order123'; // Simulation // Business logic to create the order this.eventEmitter.emit('order.created', new OrderCreatedEvent(orderId, userId, amount)); return { orderId, userId, amount }; } } Consuming the Event // payment.service.ts import { Injectable } from '@nestjs/common'; import { OnEvent } from '@nestjs/event-emitter'; import { OrderCreatedEvent } from './events/order-created.event'; @Injectable() export class PaymentService { @OnEvent('order.created') handleOrderCreated(event: OrderCreatedEvent) { console.log(`Processing payment for order: ${event.orderId}`); // Payment processing logic } } Advanced Considerations For more complex or distributed applications: Use RabbitMQ or Kafka for messaging infrastructure. Integrate patterns like CQRS and Event Sourcing for better traceability and control. Conclusion Event-driven architecture in NestJS provides a powerful way to build decoupled, scalable, and reactively efficient systems. Adopting this approach can help you create modern solutions that are ready for growth and the ever-changing development landscape.
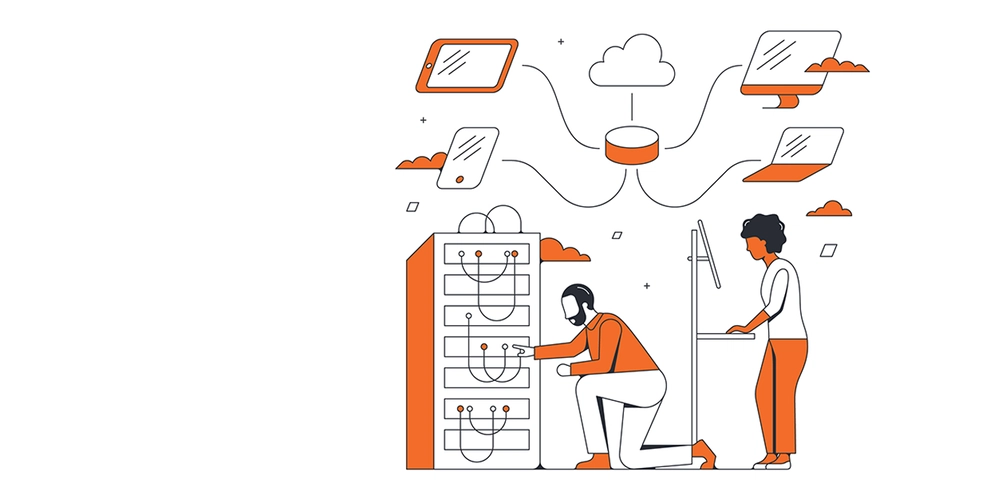
Event-Driven Architecture (EDA) is an increasingly popular approach in modern systems that require high scalability, low coupling, and asynchronous processing. We'll explore how to implement this architecture using NestJS.
What is Event-Driven Architecture?
In an event-driven architecture:
- Events represent a change in state or a significant action in the system.
- Producers generate events.
- Consumers subscribe to specific events and execute actions when those events occur.
- An event bus acts as an intermediary, enabling communication between producers and consumers without direct coupling.
This architecture enhances scalability and allows components to evolve independently.
Benefits of EDA
- Decoupling: Services do not depend directly on each other.
- Scalability: Each component can be scaled independently.
- Reactivity: Systems react to events in real-time.
- Fault Tolerance: A failure in a consumer does not affect the producer.
Implementing EDA in NestJS
NestJS supports event emission and listening through the @nestjs/event-emitter package.
Installation
npm install @nestjs/event-emitter
Module Configuration
// app.module.ts
import { Module } from '@nestjs/common';
import { EventEmitterModule } from '@nestjs/event-emitter';
@Module({
imports: [EventEmitterModule.forRoot()],
})
export class AppModule {}
Creating an Event
// events/order-created.event.ts
export class OrderCreatedEvent {
constructor(
public readonly orderId: string,
public readonly userId: string,
public readonly amount: number,
) {}
}
Emitting the Event
// orders.service.ts
import { Injectable } from '@nestjs/common';
import { EventEmitter2 } from '@nestjs/event-emitter';
import { OrderCreatedEvent } from './events/order-created.event';
@Injectable()
export class OrdersService {
constructor(private eventEmitter: EventEmitter2) {}
async createOrder(userId: string, amount: number) {
const orderId = 'order123'; // Simulation
// Business logic to create the order
this.eventEmitter.emit('order.created', new OrderCreatedEvent(orderId, userId, amount));
return { orderId, userId, amount };
}
}
Consuming the Event
// payment.service.ts
import { Injectable } from '@nestjs/common';
import { OnEvent } from '@nestjs/event-emitter';
import { OrderCreatedEvent } from './events/order-created.event';
@Injectable()
export class PaymentService {
@OnEvent('order.created')
handleOrderCreated(event: OrderCreatedEvent) {
console.log(`Processing payment for order: ${event.orderId}`);
// Payment processing logic
}
}
Advanced Considerations
For more complex or distributed applications:
Use RabbitMQ or Kafka for messaging infrastructure.
Integrate patterns like CQRS and Event Sourcing for better traceability and control.
Conclusion
Event-driven architecture in NestJS provides a powerful way to build decoupled, scalable, and reactively efficient systems. Adopting this approach can help you create modern solutions that are ready for growth and the ever-changing development landscape.