Entity/DAO for any combination of values?
You have a nice set of entities but then someone decides to add one or two more stupid values that are used only in one place (or maybe, not so stupid but ones I would rather avoid) Now, you have a choice: to add extra fields to your entity (that are not going to be used for the most part) or write a whole new entity / response DTO, copying all the fields from the existing entity (probably, a lot) and adding a couple of fields. And it's going to sit idle most of the time I don't like either choice A concrete example. I collaborate on a medical desktop Swing app. We have an entity for vaccines. Vaccines and infections are in a many-to-many relationship, there's a join table. Don't expect we use some ORM framework with @AllThoseNeatAnnotations. In one window, we need to render vaccines with asterisks beside their names if they protect against more than one infection. And the data we get also includes one field for the number of "additional" infections, qty (the vaccine table or its corresponding entity doesn't). For example, "vaccine 1" that protects against "infection A" and "infection B" would come from the DB with text as "vaccine 1", vaccine_text as "vaccine 1*" and qty as "1" (if it doesn't make a ton of sense to you, I can relate). Am I supposed to create a whole new entity / DTO for that? I guess I can make some decorator that wraps the entity and a map of additional fields. So far, it's the most appealing option import java.util.Map; public class ExtendedEntity { private final T entity; private final Map additionalProperties; private ExtendedEntity(T entity, Map additionalProperties) { this.entity = entity; this.additionalProperties = additionalProperties; } public ExtendedEntity of(T entity, Map additionalProperties) { return new ExtendedEntity(entity, additionalProperties); } @Override public T getEntity() { return entity; } public Object getAdditionalValue(String propertyName) { return additionalProperties.get(propertyName); } } What should I do, as a frontend developer?
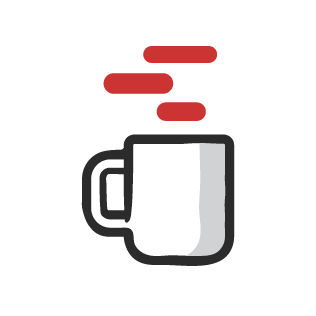
You have a nice set of entities
but then someone decides to add one or two more stupid values that are used only in one place (or maybe, not so stupid but ones I would rather avoid)
Now, you have a choice: to add extra fields to your entity (that are not going to be used for the most part) or write a whole new entity / response DTO, copying all the fields from the existing entity (probably, a lot) and adding a couple of fields. And it's going to sit idle most of the time
I don't like either choice
A concrete example. I collaborate on a medical desktop Swing app. We have an entity for vaccines. Vaccines and infections are in a many-to-many relationship, there's a join table. Don't expect we use some ORM framework with @AllThoseNeatAnnotations
. In one window, we need to render vaccines with asterisks beside their names if they protect against more than one infection. And the data we get also includes one field for the number of "additional" infections, qty
(the vaccine table or its corresponding entity doesn't). For example, "vaccine 1" that protects against "infection A" and "infection B" would come from the DB with text
as "vaccine 1", vaccine_text
as "vaccine 1*" and qty
as "1" (if it doesn't make a ton of sense to you, I can relate). Am I supposed to create a whole new entity / DTO for that?
I guess I can make some decorator that wraps the entity and a map of additional fields. So far, it's the most appealing option
import java.util.Map;
public class ExtendedEntity {
private final T entity;
private final Map additionalProperties;
private ExtendedEntity(T entity, Map additionalProperties) {
this.entity = entity;
this.additionalProperties = additionalProperties;
}
public ExtendedEntity of(T entity, Map additionalProperties) {
return new ExtendedEntity<>(entity, additionalProperties);
}
@Override
public T getEntity() {
return entity;
}
public Object getAdditionalValue(String propertyName) {
return additionalProperties.get(propertyName);
}
}
What should I do, as a frontend developer?