Cruise on Kubernetes with Helm
Introduction Managing Kubernetes resources efficiently can be complex, especially when deploying applications across multiple environments. Helm, an open-source package manager for Kubernetes, simplifies this process by providing a templating mechanism, version control, and reusable configuration management. In this blog, we'll explore Helm's capabilities with some use cases and examples. Helm Charts overview Helm is a tool that helps deploy, manage, and version Kubernetes applications using Helm charts. A Helm chart is a collection of YAML files that define a Kubernetes application and its dependencies in a structured and reusable way. Why is Helm Needed for Kubernetes? Kubernetes provides powerful resource management but lacks an efficient way to package, version, and distribute applications leading to multiple identical YAML manifests. Helm fills this gap by: Standardizing deployments with predefined templates. Templating reduces redundancy by allowing parameterized configurations so different values can be passed across different environments to the same template. Providing version control for application deployments and maintaining revision history. Managing complex dependencies between Kubernetes resources, ensuring they are installed alongside the main application. Built-in Functions in Helm and their Use Cases Helm provides several built-in functions to simplify templating. Here are some commonly used ones with their syntax: 1. include Used to include content from other templates or process templates dynamically. {{ include "common.labels" . }} 2. toYaml Converts an object into YAML format. config: {{ .Values.config | toYaml | indent 2 }} 3. default Provides a default value if none is supplied. replicas: {{ .Values.replicas | default 3 }} 4. required Ensures a value is provided; otherwise, it throws an error. apiVersion: v1 metadata: name: {{ required "A name is required" .Values.name }} You can check out the full list of Template functions offered by Helm here --> Template Function List Role of _helpers.tpl File? The _helpers.tpl file defines reusable template functions that keep Helm charts DRY (Don't Repeat Yourself). It also gives you the capability of writing a custom template function based on your use case. Example: Defining a Common Label {{- define "common.labels" -}} app.kubernetes.io/name: {{ .Chart.Name }} app.kubernetes.io/version: {{ .Chart.Version }} {{- end -}} This function can be included in other YAML files: metadata: labels: {{ include "common.labels" . | indent 4 }} Custom Helper Functions Custom functions help standardize naming conventions and ensure consistency. For example, here's a simple function I applied on a personal project while using helm. More about the project repository in the README file here {{/* Add resource kind as prefix to metadata.name */}} {{- define "resourceName" -}} {{- $kind := (base .Template.Name) | trimSuffix ".yaml" -}} {{- printf "%s-%s" $kind .Chart.Name | trunc 63 | trimSuffix "-" }} {{- end -}} Then, use it in a deployment file: metadata: name: {{ include "resourceName" . }} The result would have resource kind as a prefix in resourceName: Important Annotations: Helm Hooks Hooks allow users to perform tasks or execute scripts before or after a deployment. These provide an event based control to the user which can be applied in cases like enabling pre-install checks, database migrations, or cleanup after deployment. apiVersion: v1 kind: Job metadata: name: "{{ .Release.Name }}-pre-install" annotations: "helm.sh/hook": pre-install To view available hooks and their descriptions, click here Hook weight and deletion policy Hooks weights determines the order of execution of the corresponding hook resource, starting from lowest to highest number. Hook weight can also be negative and the same principle would apply to it. By default, all hooks are set at "0". Hook weight must be represented as strings. annotations: "helm.sh/hook-weight": "5" Hook deletion policy as name suggests would determine when a hook will be deleted. By default, it's set to "beforeHookCreation". It also supports multiple deletion policies at a time, like: annotations: "helm.sh/hook-delete-policy": before-hook-creation,hook-succeeded Checkout the current supported delete policies in helm: Conclusion Helm simplifies Kubernetes application management by making deployments reproducible, scalable, and version-controlled. With Helm, DevOps engineers can efficiently manage complex deployments, reduce configuration errors, and enhance maintainability. Mastering Helm will significantly improve your Kubernetes workflows, making deployments smoother and more automated.
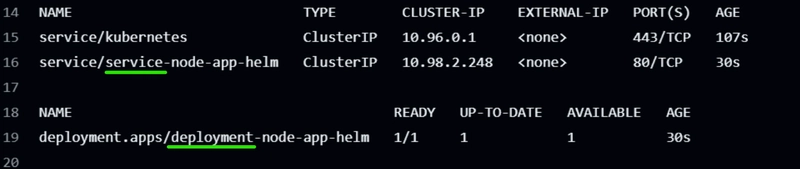
Introduction
Managing Kubernetes resources efficiently can be complex, especially when deploying applications across multiple environments. Helm, an open-source package manager for Kubernetes, simplifies this process by providing a templating mechanism, version control, and reusable configuration management. In this blog, we'll explore Helm's capabilities with some use cases and examples.
Helm Charts overview
Helm is a tool that helps deploy, manage, and version Kubernetes applications using Helm charts. A Helm chart is a collection of YAML files that define a Kubernetes application and its dependencies in a structured and reusable way.
Why is Helm Needed for Kubernetes?
Kubernetes provides powerful resource management but lacks an efficient way to package, version, and distribute applications leading to multiple identical YAML manifests. Helm fills this gap by:
- Standardizing deployments with predefined templates. Templating reduces redundancy by allowing parameterized configurations so different values can be passed across different environments to the same template.
- Providing version control for application deployments and maintaining revision history.
- Managing complex dependencies between Kubernetes resources, ensuring they are installed alongside the main application.
Built-in Functions in Helm and their Use Cases
Helm provides several built-in functions to simplify templating. Here are some commonly used ones with their syntax:
1. include
Used to include content from other templates or process templates dynamically.
{{ include "common.labels" . }}
2. toYaml
Converts an object into YAML format.
config: {{ .Values.config | toYaml | indent 2 }}
3. default
Provides a default value if none is supplied.
replicas: {{ .Values.replicas | default 3 }}
4. required
Ensures a value is provided; otherwise, it throws an error.
apiVersion: v1
metadata:
name: {{ required "A name is required" .Values.name }}
You can check out the full list of Template functions offered by Helm here --> Template Function List
Role of _helpers.tpl
File?
The _helpers.tpl
file defines reusable template functions that keep Helm charts DRY (Don't Repeat Yourself). It also gives you the capability of writing a custom template function based on your use case.
Example: Defining a Common Label
{{- define "common.labels" -}}
app.kubernetes.io/name: {{ .Chart.Name }}
app.kubernetes.io/version: {{ .Chart.Version }}
{{- end -}}
This function can be included in other YAML files:
metadata:
labels:
{{ include "common.labels" . | indent 4 }}
Custom Helper Functions
Custom functions help standardize naming conventions and ensure consistency. For example, here's a simple function I applied on a personal project while using helm. More about the project repository in the README file here
{{/*
Add resource kind as prefix to metadata.name
*/}}
{{- define "resourceName" -}}
{{- $kind := (base .Template.Name) | trimSuffix ".yaml" -}}
{{- printf "%s-%s" $kind .Chart.Name | trunc 63 | trimSuffix "-" }}
{{- end -}}
Then, use it in a deployment file:
metadata:
name: {{ include "resourceName" . }}
The result would have resource kind as a prefix in resourceName:
Important Annotations:
Helm Hooks
Hooks allow users to perform tasks or execute scripts before or after a deployment. These provide an event based control to the user which can be applied in cases like enabling pre-install checks, database migrations, or cleanup after deployment.
apiVersion: v1
kind: Job
metadata:
name: "{{ .Release.Name }}-pre-install"
annotations:
"helm.sh/hook": pre-install
To view available hooks and their descriptions, click here
Hook weight and deletion policy
Hooks weights determines the order of execution of the corresponding hook resource, starting from lowest to highest number. Hook weight can also be negative and the same principle would apply to it. By default, all hooks are set at "0". Hook weight must be represented as strings.
annotations:
"helm.sh/hook-weight": "5"
Hook deletion policy as name suggests would determine when a hook will be deleted. By default, it's set to "beforeHookCreation". It also supports multiple deletion policies at a time, like:
annotations:
"helm.sh/hook-delete-policy": before-hook-creation,hook-succeeded
Checkout the current supported delete policies in helm:
Conclusion
Helm simplifies Kubernetes application management by making deployments reproducible, scalable, and version-controlled. With Helm, DevOps engineers can efficiently manage complex deployments, reduce configuration errors, and enhance maintainability. Mastering Helm will significantly improve your Kubernetes workflows, making deployments smoother and more automated.
Thanks for making it till the end. Drop some feedback or queries in the comments below.