Cross-Origin Resource Sharing
What is (CORS) Cross-Origin Resource Sharing ? In simple words, is a mechanism for integrating applications.For example, your application may use your browser to pull videos from a video platform API, use fonts from a public font library, or display weather data from a national weather database. CORS allows the client browser to check with the third-party servers if the request is authorized before any data transfers. What does "other-domain" means? means the URL being accessed differs from the location that the JavaScript is running from, by having: a different scheme (HTTP or HTTPS) a different domain a different port Why do we need CORS? This is a worst-case scenario, where everything is wide open. But it still exemplifies what a CORS attack looks like. In fact, in 2016, Facebook was found to be vulnerable to such a CORS attack. One domain + port combination cannot read the response to a request from a different domain + port combination. How to allow CORS in react.js? Using proxy in package.json file. Add the API part to package.json file. Then make request :- React.useEffect(() => { axios .get('api/profile/') .then(function (response) { console.log(response); }) .catch(function (error) { console.log(error); }); }); How to prevent CORS-based attacks? Specify the allowed origins Only allow trusted sites Don’t whitelist “null” Implement proper server-side security policies For more information and installation visit - www.npmjs.com ⓢⓗⓞⓦ ⓛⓞⓥⓔ ⓐⓝⓓ ⓢⓤⓟⓟⓞⓡⓣ
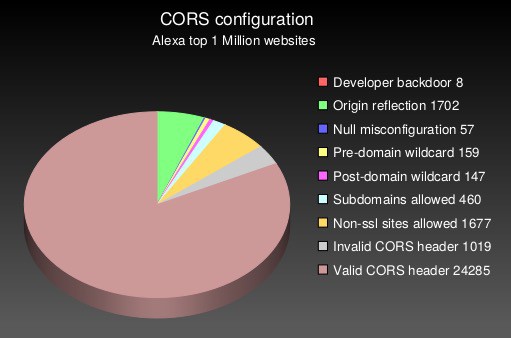
What is (CORS) Cross-Origin Resource Sharing ?
In simple words, is a mechanism for integrating applications.For example, your application may use your browser to pull videos from a video platform API, use fonts from a public font library, or display weather data from a national weather database. CORS allows the client browser to check with the third-party servers if the request is authorized before any data transfers.
What does "other-domain" means?
means the URL being accessed differs from the location that the JavaScript is running from, by having:
- a different scheme (HTTP or HTTPS)
- a different domain
- a different port
Why do we need CORS?
This is a worst-case scenario, where everything is wide open. But it still exemplifies what a CORS attack looks like. In fact, in 2016, Facebook was found to be vulnerable to such a CORS attack.
One domain + port combination cannot read the response to a request from
a different domain + port combination.
How to allow CORS in react.js?
- Using
proxy
inpackage.json
file. - Add the API part to
package.json
file. - Then make request :-
React.useEffect(() => {
axios
.get('api/profile/')
.then(function (response) {
console.log(response);
})
.catch(function (error) {
console.log(error);
});
});
How to prevent CORS-based attacks?
- Specify the allowed origins
- Only allow trusted sites
- Don’t whitelist “null”
- Implement proper server-side security policies
For more information and installation visit - www.npmjs.com
ⓢⓗⓞⓦ ⓛⓞⓥⓔ ⓐⓝⓓ ⓢⓤⓟⓟⓞⓡⓣ